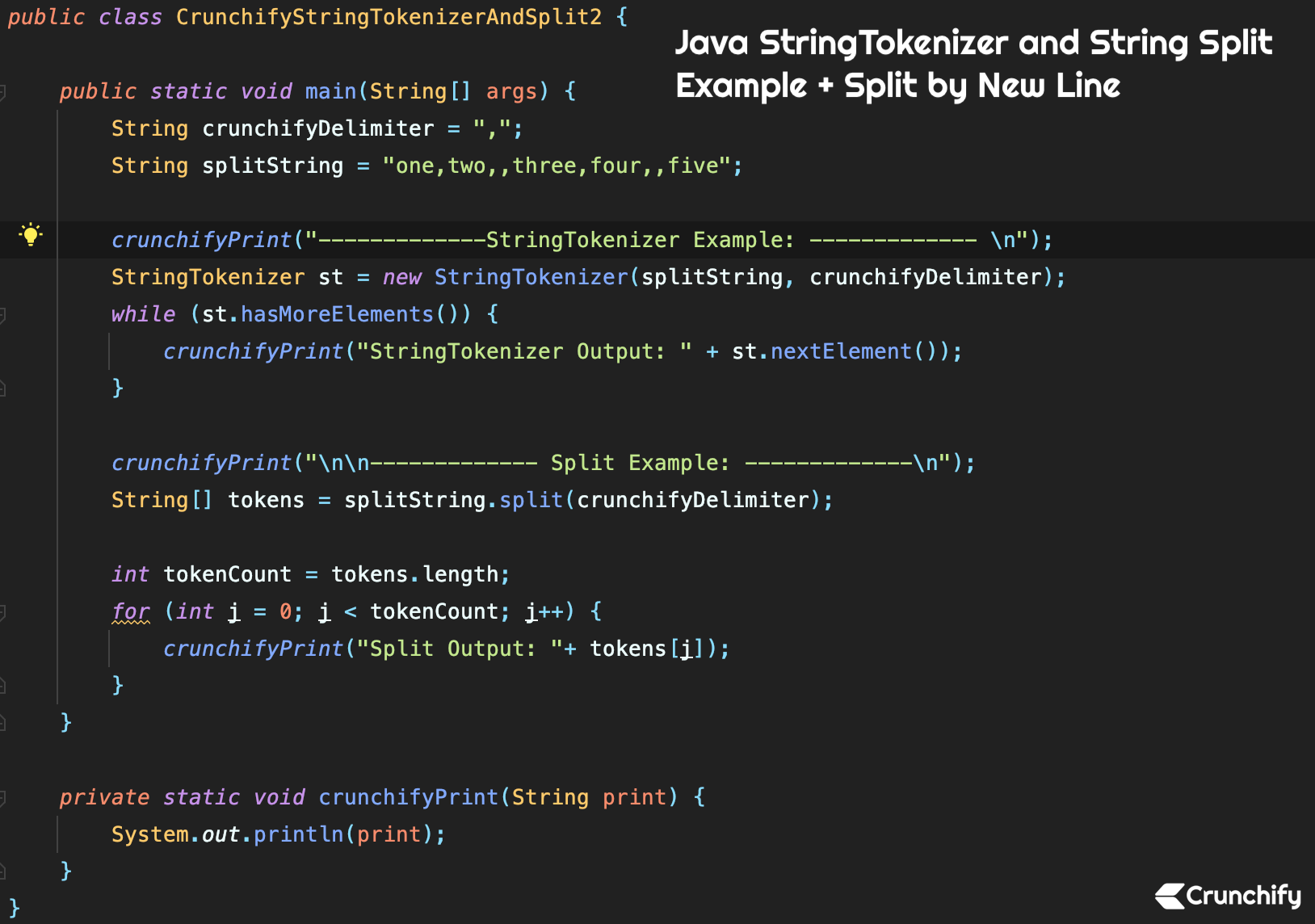
How to Split a string using String.split()
? Understanding the Java Split String Method.
StringTokenizer()
ignores empty string but split()
won’t. 🙂
Let’s get started.
Java StringTokenizer:
In Java, the string tokenizer class allows an application to break a string into tokens. The tokenization method is much simpler than the one used by the StreamTokenizer
class.
The StringTokenizer
methods do not distinguish among identifiers, numbers, and quoted strings, nor do they recognize and skip comments.
Java String Split:
Splits this string around matches of the given regular expression.
This method works as if by invoking the two-argument split
method with the given expression and a limit argument of zero. Trailing empty strings are therefore not included in the resulting array.
If you want to split by new line
then you could use below:
String lines[] = String.split("\\r?\\n");
There’s only two newlines
(UNIX and Windows) that you need to worry about.
Other must read:
- https://crunchify.com/write-java-program-to-print-fibonacci-series-upto-n-number/
- List of more than 100 Java Tutorial
Create class: CrunchifyStringTokenizerAndSplit.java
Add below code to Java file.
package crunchify.com.tutorials; import java.util.StringTokenizer; /** * @author Crunchify.com * */ public class CrunchifyStringTokenizerAndSplit2 { public static void main(String[] args) { String crunchifyDelimiter = ","; String splitString = "one,two,,three,four,,five"; crunchifyPrint("-------------StringTokenizer Example: ------------- \n"); StringTokenizer st = new StringTokenizer(splitString, crunchifyDelimiter); while (st.hasMoreElements()) { crunchifyPrint("StringTokenizer Output: " + st.nextElement()); } crunchifyPrint("\n\n------------- Split Example: -------------\n"); String[] tokens = splitString.split(crunchifyDelimiter); int tokenCount = tokens.length; for (int j = 0; j < tokenCount; j++) { crunchifyPrint("Split Output: "+ tokens[j]); } } private static void crunchifyPrint(String print) { System.out.println(print); } }
Output:
Just run above program in Eclipse console you should see result as below.
/Library/Java/JavaVirtualMachines/jdk-15.jdk/Contents/Home/bin/java -javaagent:/Applications/IntelliJ :/Users/app/.m2/repository/org/springframework/spring-context-support/5.1.3.RELEASE/ spring-context-support-5.1.3.RELEASE.jar crunchify.com.tutorials.CrunchifyStringTokenizerAndSplit2 -------------StringTokenizer Example: ------------- StringTokenizer Output: one StringTokenizer Output: two StringTokenizer Output: three StringTokenizer Output: four StringTokenizer Output: five ------------- Split Example: ------------- Split Output: one Split Output: two Split Output: Split Output: three Split Output: four Split Output: Split Output: five Process finished with exit code 0
Let me know if you see any issue running this program and we are happy to check it out quickly.