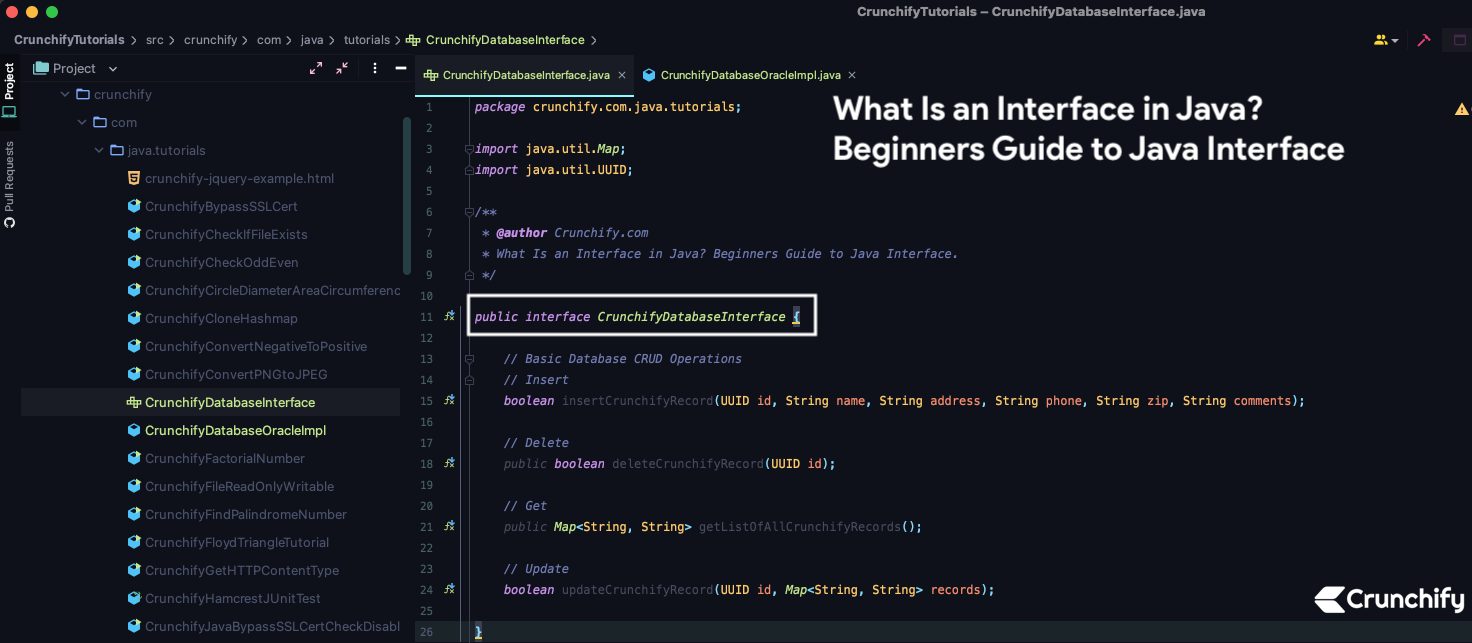
Why and when to use an Interfaces?
- An interface is a reference type in Java.
- It is similar to class.
- It is a collection of abstract methods.
- It is used to achieve total abstraction.
I believe this is the first question you might expect in Java Interview. Very basic questions but widely used in interview 🙂
There is no perfect answer for this and there are number of ways to answer this question. Might be your interviewer looking for practical approach of this questions? Possible.
Then let’s start with basic definition of a Java Interface
We will go over the same with multiple examples.
- What is an Interface in Java?
- What is an interface in java with realtime example?
- Why use an interface in java
- Interface Design Java
- Most common interview questions on Interface
Interface fundamentals:
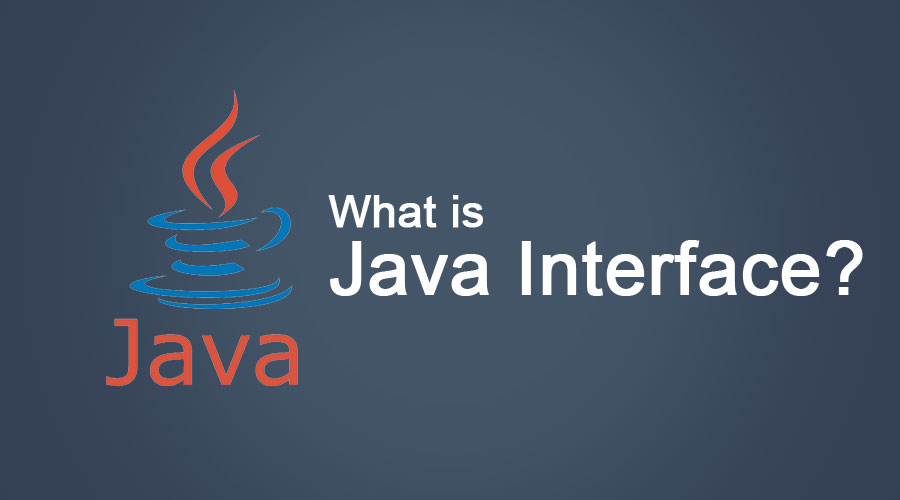
- An
interface
is just a contract, a description of the behavior an implementing class will have. The implementing class ensures, that it will have these methods that can be used on it. It is basically a contract or a promise the class has to make. - What if in your projects all of the various implementations share the same method signatures? Interface works best in that case.
- At the later stage after major project implementation let’s see if you have implemented interface definitions to ~50 places, what if you change interface? You have to make modification to all 50 places in your projects.
- It’s advisable to spend some more time defining Interface during design phase rather change it at later stage 🙂
- An interface is consist of
singleton
variables (public static final
) andpublic abstract
methods. We normally prefer interface in real time when we know what to do but don’t know how to do. An interface cannot contain instance fields. - The classes which implement the Interface must provide the method definition for all the methods present.
- A Class may implement several interfaces.
- An interface implementation may be added to any existing third party class.
- An interface can contain any number of methods.
- In Java you cannot instantiate an interface.
- An Interface does not contain any constructors.
- An interface is not extended by a class; it is implemented by a class.
- An interface can extend multiple interfaces.
Interface Examples:
Tip 1.
Create Interface CrunchifyDatabaseInterface.java
package crunchify.com.java.tutorials; import java.util.Map; import java.util.UUID; /** * @author Crunchify.com * What Is an Interface in Java? Beginners Guide to Java Interface. */ public interface CrunchifyDatabaseInterface { // Basic Database CRUD Operations // Insert boolean insertCrunchifyRecord(UUID id, String name, String address, String phone, String zip, String comments); // Delete public boolean deleteCrunchifyRecord(UUID id); // Get public Map<String, String> getListOfAllCrunchifyRecords(); // Update boolean updateCrunchifyRecord(UUID id, Map<String, String> records); }
Tip 2.
Implement Interface CrunchifyDatabaseOracleImpl.java
When you 1st implements an interface, Eclipse will show you add unimplemented methods.
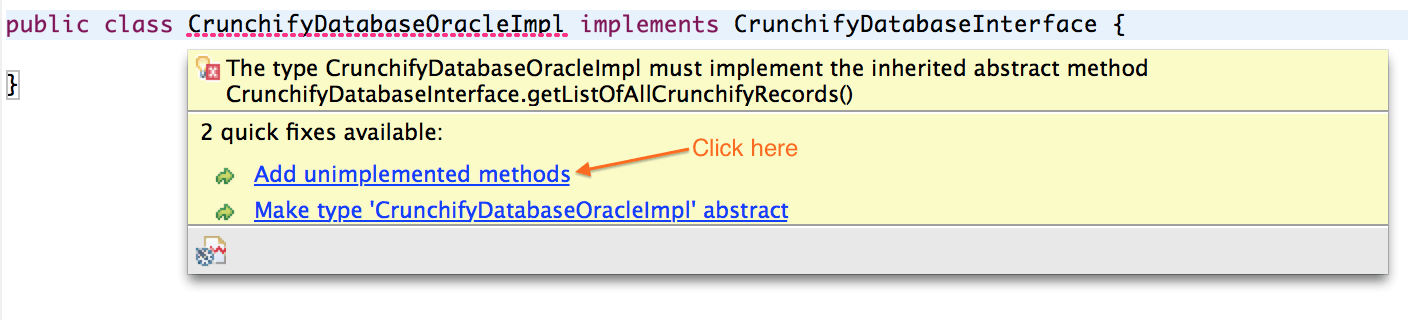
Just click on “Add unimplemented methods
” and your IMPL class should be ready with Auto-generated method stub
.
Tip 3. Actual Impl Method.
package crunchify.com.java.tutorials; import java.util.Map; import java.util.UUID; /** * @author Crunchify.com * What Is an Interface in Java? Beginners Guide to Java Interface. */ public class CrunchifyDatabaseOracleImpl implements CrunchifyDatabaseInterface { // Override: Indicates that a method declaration is intended to override a method declaration in a supertype. // If a method is annotated with this annotation type compilers are required to generate an error message unless at least one of the following conditions hold: // The method does override or implement a method declared in a supertype. // The method has a signature that is override-equivalent to that of any public method declared in Object. @Override public boolean insertCrunchifyRecord(UUID id, String name, String address, String phone, String zip, String comments) { // TODO Provide your actual implementation here based on your need specific to Oracle return false; } // UUID(): A class that represents an immutable universally unique identifier (UUID). // A UUID represents a 128-bit value. // There exist different variants of these global identifiers. // The methods of this class are for manipulating the Leach-Salz variant, although the constructors allow the creation of any variant of UUID @Override public boolean deleteCrunchifyRecord(UUID id) { // TODO Provide your actual implementation here based on your need specific to Oracle return false; } // Map(): An object that maps keys to values. A map cannot contain duplicate keys; each key can map to at most one value. // This interface takes the place of the Dictionary class, which was a totally abstract class rather than an interface. @Override public Map<String, String> getListOfAllCrunchifyRecords() { // TODO Provide your actual implementation here based on your need specific to Oracle return null; } @Override public boolean updateCrunchifyRecord(UUID id, Map<String, String> records) { // TODO Provide your actual implementation here based on your need specific to Oracle return false; } }
Tip 4.
Similar way you could use the same Interface to implement different Database specific operations. Like, for DB2, MySQL, MongoDB, Cassandra DB, etc.
What’s next?
In process of writing Tutorial on Abstract Class and then another tutorial which clearly shows Difference between Abstract Class and Interface.