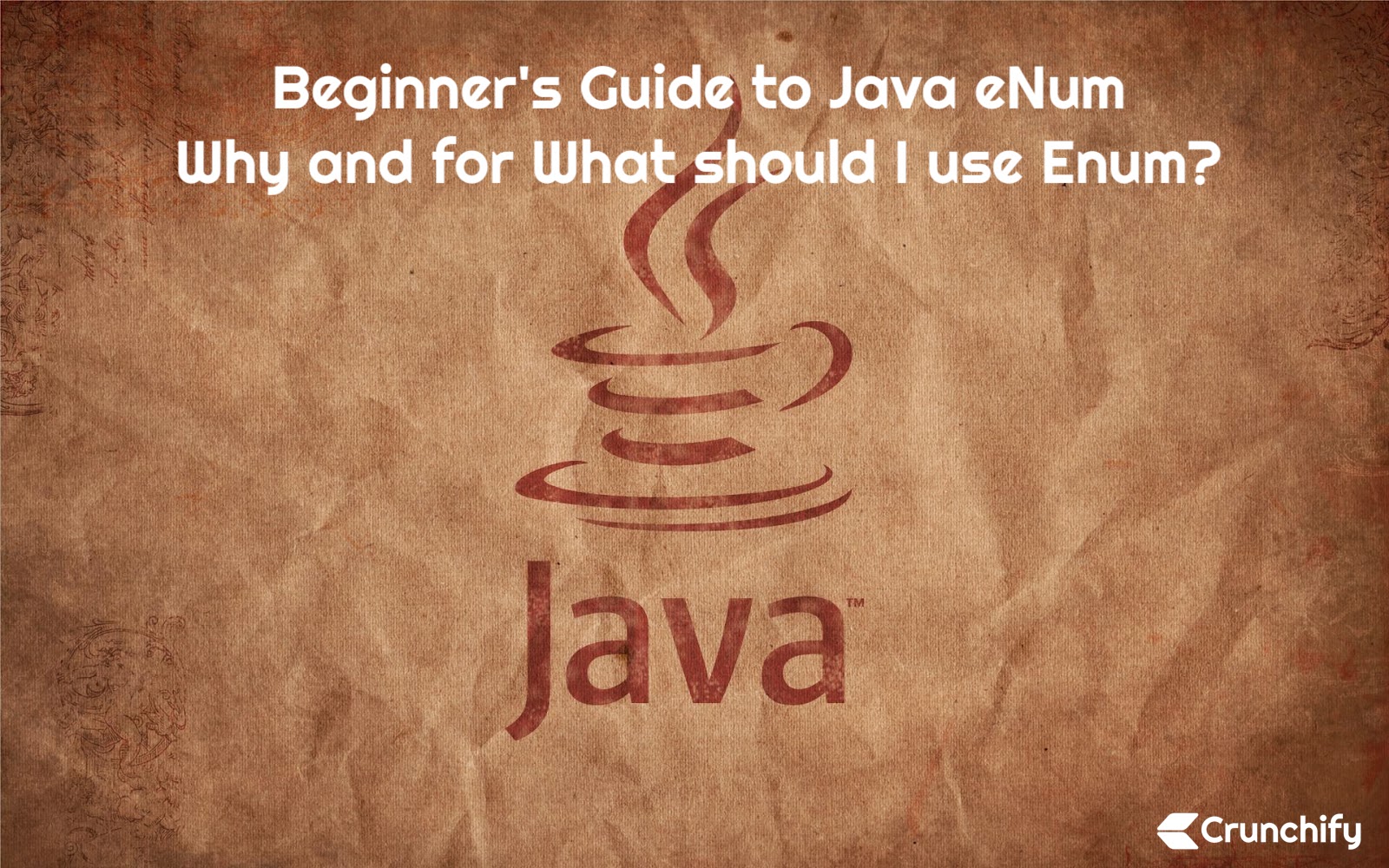
Enums are lists of constants
like unchangeable variables. Have you heard of Final keyword
? It’s like that.
When you need a predefined list of values which do represent some kind of numeric or textual data, you should use an enum. For instance, in a chess game you could represent the different types of pieces as an enum:
enum ChessPiece { PAWN, ROOK, KNIGHT, BISHOP, QUEEN, KING; }
You should always use enums when a variable (especially a method parameter) can only take one out of a small set of possible values. Examples would be things like type constants (contract status: “permanent”, “temp”, “apprentice”), or flags (“execute now”, “defer execution”).
If you use enums instead of integers (or String codes), you increase compile-time checking and avoid errors from passing in invalid constants, and you document which values are legal to use.
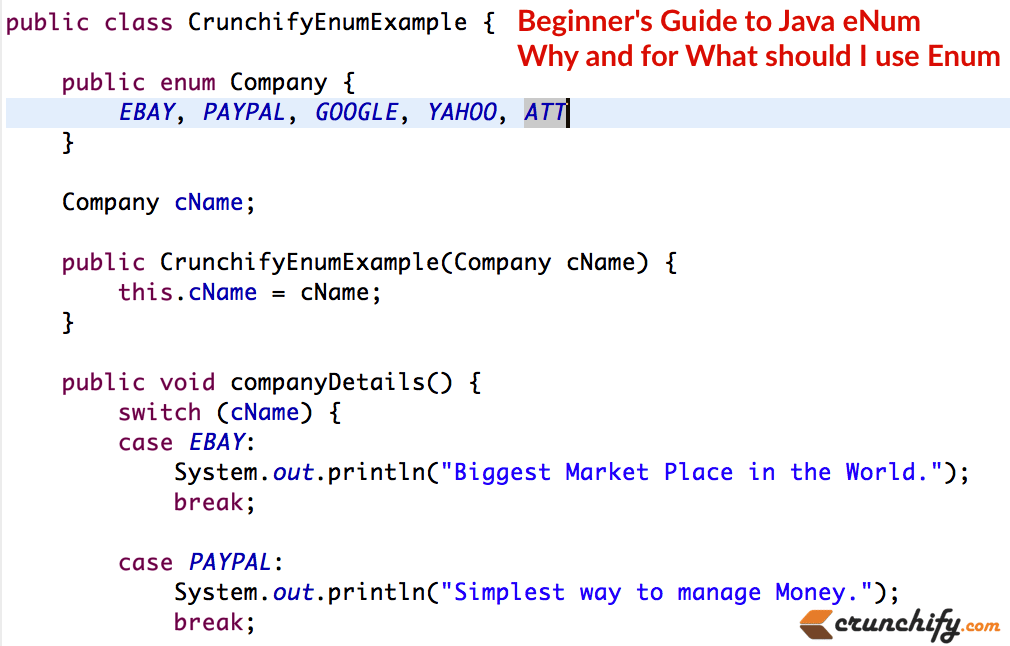
Java Example:
package com.crunchify.tutorials; /** * @author Crunchify.com */ public class CrunchifyEnumExample { public enum Company { EBAY, PAYPAL, GOOGLE, YAHOO, ATT } Company cName; public CrunchifyEnumExample(Company cName) { this.cName = cName; } public void companyDetails() { switch (cName) { case EBAY: System.out.println("EBAY: Biggest Market Place in the World."); break; case PAYPAL: System.out.println("PAYPAL: Simplest way to manage Money."); break; case GOOGLE: case YAHOO: System.out.println("YAHOO: 1st Web 2.0 Company."); break; default: System.out.println("DEFAULT: Google - biggest search giant.. ATT - my carrier provider.."); break; } } public static void main(String[] args) { CrunchifyEnumExample ebay = new CrunchifyEnumExample(Company.EBAY); ebay.companyDetails(); CrunchifyEnumExample paypal = new CrunchifyEnumExample(Company.PAYPAL); paypal.companyDetails(); CrunchifyEnumExample google = new CrunchifyEnumExample(Company.GOOGLE); google.companyDetails(); CrunchifyEnumExample yahoo = new CrunchifyEnumExample(Company.YAHOO); yahoo.companyDetails(); CrunchifyEnumExample att = new CrunchifyEnumExample(Company.ATT); att.companyDetails(); } }
Output:
EBAY: Biggest Market Place in the World. PAYPAL: Simplest way to manage Money. YAHOO: 1st Web 2.0 Company. YAHOO: 1st Web 2.0 Company. DEFAULT: Google - biggest search giant.. ATT - my carrier provider.. Process finished with exit code 0
Some very important points on Java Enum:
Point-1
All enums implicitly extend java.lang.Enum
. Since Java does not support multiple inheritance, an enum cannot extend anything else.
Point-2
Enum in Java are type-safe:
Enum has there own name-space. It means your enum will have a type for example “Company” in below example and you can not assign any value other than specified in Enum Constants.
public enum Company { EBAY, PAYPAL, GOOGLE, YAHOO, ATT } Company cName = Company.EBAY; cName = 1; // Compilation Error
Point-3
You can specify values of enum constants at the creation time. MyEnum.values()
returns an array of MyEnum’s values.
package com.crunchify.tutorial; /** * @author Crunchify.com */ public class CrunchifyEnumExample { public enum Company { EBAY(30), PAYPAL(10), GOOGLE(15), YAHOO(20), ATT(25); private int value; private Company(int value) { this.value = value; } } public static void main(String[] args) { for (Company cName : Company.values()) { System.out.println("Company Value: " + cName.value + " - Company Name: " + cName); } } }
Output:
Company Value: 30 - Company Name: EBAY Company Value: 10 - Company Name: PAYPAL Company Value: 15 - Company Name: GOOGLE Company Value: 20 - Company Name: YAHOO Company Value: 25 - Company Name: ATT
Point-4
Enum constants are implicitly static and final and can not be changed once created.
Point-5
Enum can be safely compare using:
- Switch-Case Statement
- == Operator
- .equals() methodPlease follow complete tutorial.
Company eBay = Company.EBAY; if(eBay == Company.EBAY){ log.info("enum in java can be compared using =="); }
Point-6
You can not create instance of enums by using new operator in Java because constructor of Enum in Java can only be private and Enums constants can only be created inside Enums itself.
Point-7
Instance of Enum in Java is created when any Enum constants are first called or referenced in code.
Point-8
An enum specifies a list of constant values assigned to a type.
Point-9
An enum can be declared outside or inside a class, but NOT in a method.
Point-10
An enum declared outside a class must NOT be marked static, final , abstract, protected , or private
Point-11
Enums can contain constructors, methods, variables, and constant class bodies.
Point-12
enum constants can send arguments to the enum constructor, using the syntax BIG(8), where the int literal 8 is passed to the enum constructor.
Point-13
enum constructors can have arguments, and can be overloaded.
Point-14
enum constructors can NEVER be invoked directly in code. They are always called automatically when an enum is initialized.
Point-15
The semicolon at the end of an enum declaration is optional.
These are legal:
enum Foo { ONE, TWO, THREE} enum Foo { ONE, TWO, THREE};
Another simple Java eNUM Example:
Enums are lists of constants. When you need a predefined list of values which do represent some kind of numeric or textual data, you should use an enum.
You should always use enums when a variable (especially a method parameter) can only take one out of a small set of possible values. Examples would be things like type constants (contract status: “permanent”, “temp”, “apprentice”), or flags (“execute now”, “defer execution”).
If you use enums instead of integers (or String codes), you increase compile-time checking and avoid errors from passing in invalid constants, and you document which values are legal to use.
Between, overuse of enums might mean that your methods do too much (it’s often better to have several separate methods, rather than one method that takes several flags which modify what it does), but if you have to use flags or type codes, enums are the way to go.
This is very simple Java eNum Example
/** * @author Crunchify.com */ public enum CrunchifyEnumCompany { GOOGLE("G"), YAHOO("Y"), EBAY("E"), PAYPAL("P"); private String companyLetter; private CrunchifyEnumCompany(String s) { companyLetter = s; } public String getCompanyLetter() { return companyLetter; } } package com.crunchify.tutorials; import com.crunchify.tutorials.CrunchifyEnumCompany; /** * @author Crunchify.com */ public class CrunchifyEnumExample { public static void main(String[] args) { System.out.println("Get enum value for Company 'eBay': " + CrunchifyEnumCompany.EBAY.getCompanyLetter()); } }
Output:
Get enum value for Company 'eBay': Value: E