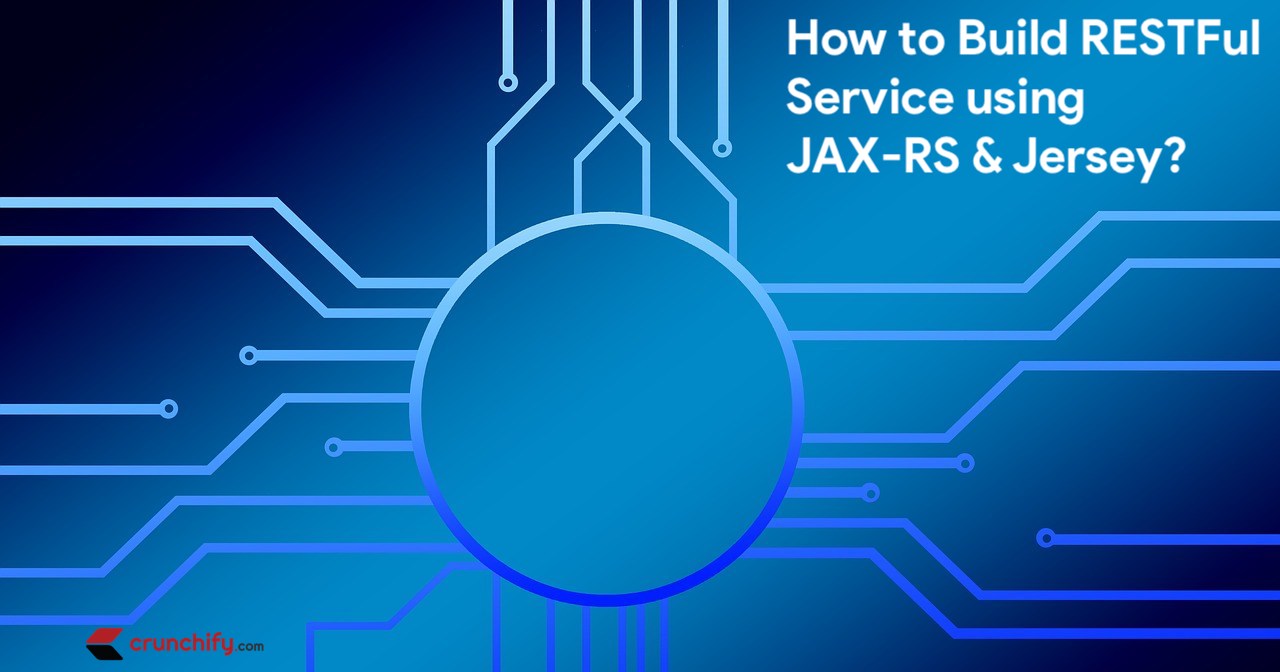
Do you have one of the below query/question?
- Write a java REST service to convert Fahrenheit to Celsius and Celcius to Fahrenheit
- REST with Java (JAX-RS) using Jersey
- Conversion from Fahrenheit to Celsius Method in Java
- Homework help w/ conversion of degrees(CtoF, FtoC)
- Java API for RESTful Web Services
- web services – Which is the best Java REST API?
- REST API for Java?
- ctof and ftoc tutorial. ctof and ftoc Java Tutorial
- Create RESTful Web services with Java technology
- RESTful web API framework for Java
RESTful Service
: Representational State Transfer (REST) has gained widespread acceptance across the Web as a simpler alternative to SOAP and Web Services Description Language (WSDL) based Web services.
REST defines a set of architectural principles by which you can design Web services that focus on a system’s resources, including how resource states are addressed and transferred over HTTP by a wide range of clients written in different languages. If measured by the number of Web services that use it, REST has emerged in the last few years alone as a predominant Web service design model. In fact, REST has had such a large impact on the Web that it has mostly displaced SOAP- and WSDL-based interface design because it’s a considerably simpler style to use.
RESTFul Vs. SOAP Tutorial.
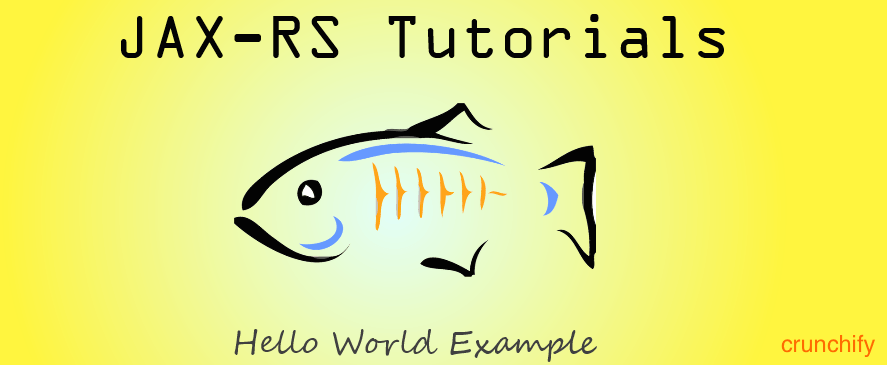
JAX-RS:
Java API for RESTful Web Services (JAX-RS), is a set if APIs to developer REST service. JAX-RS is part of the Java EE6, and make developers to develop REST web application easily.
Jersey:
Jersey is the open source, production quality, JAX-RS (JSR 311) Reference Implementation for building RESTful Web services. But, it is also more than the Reference Implementation. Jersey provides an API so that developers may extend Jersey to suit their needs.
Let’s start building simple RESTful API
with below steps:
Step-1
In Eclipse => File => New => Dynamic Web Project. Name it as “CrunchifyRESTJerseyExample
“.
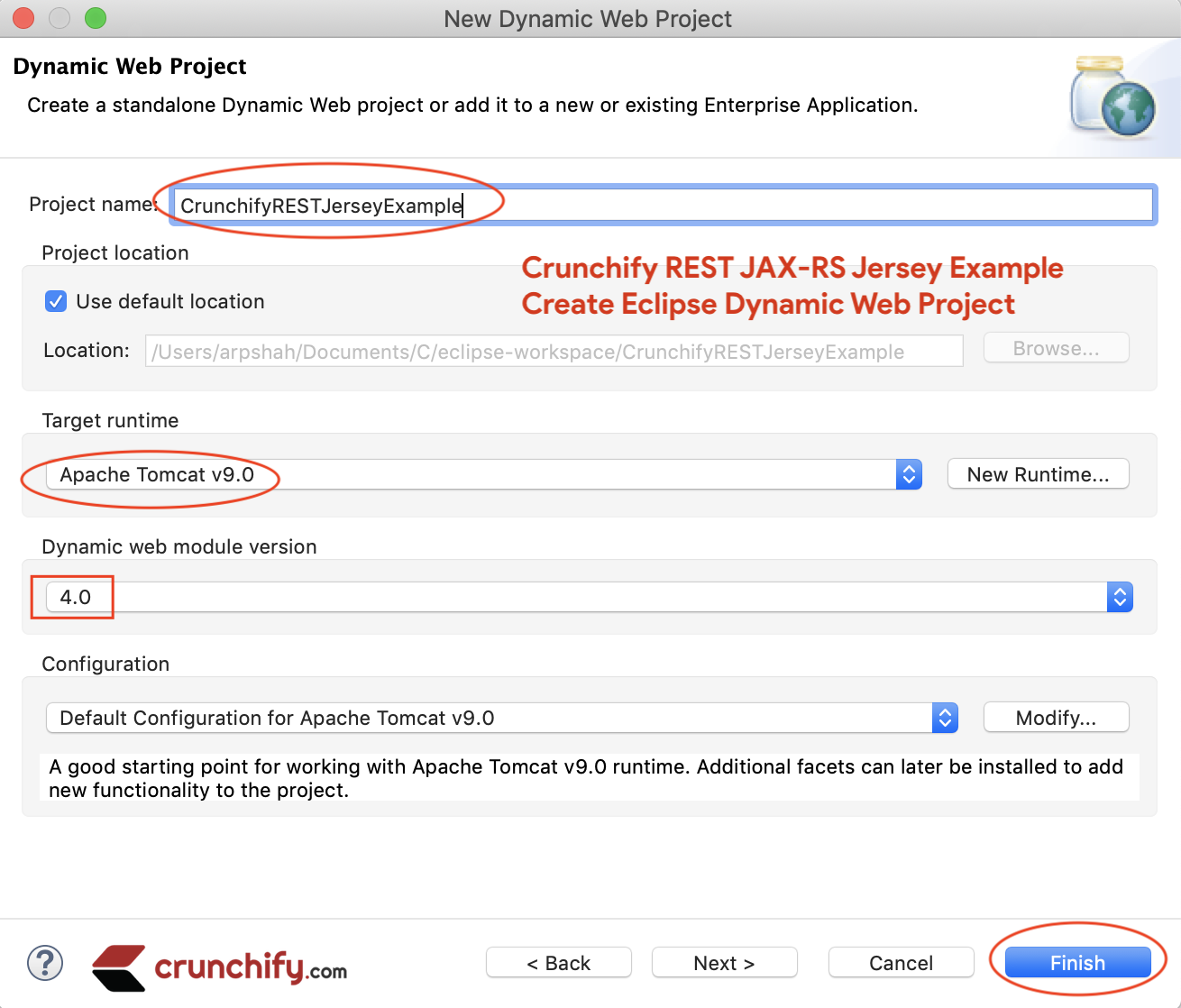
Make sure you set:
- Target runtime: Apache Tomcat v9.0
- Dynamic web module version: 4.0
Step-2
If you don’t see web.xml
(deployment descriptor) then follow these steps. OR
- Right click on project
- Select
Java EE Tools
- Click on
Generate Deployment Descriptor Stub
This will create web.xml
file under /WebContent/WEB-INF/
folder.
Step-3
Now convert Project to Maven Project
so we could add required .jar files as dependencies.
Steps:
- Right click on project
- Click on
Configure
- Select option
Convert to Maven Project
.
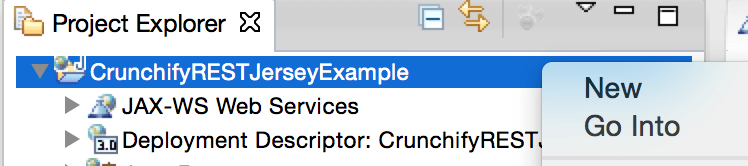

Just click on Finish button
without making any changes.
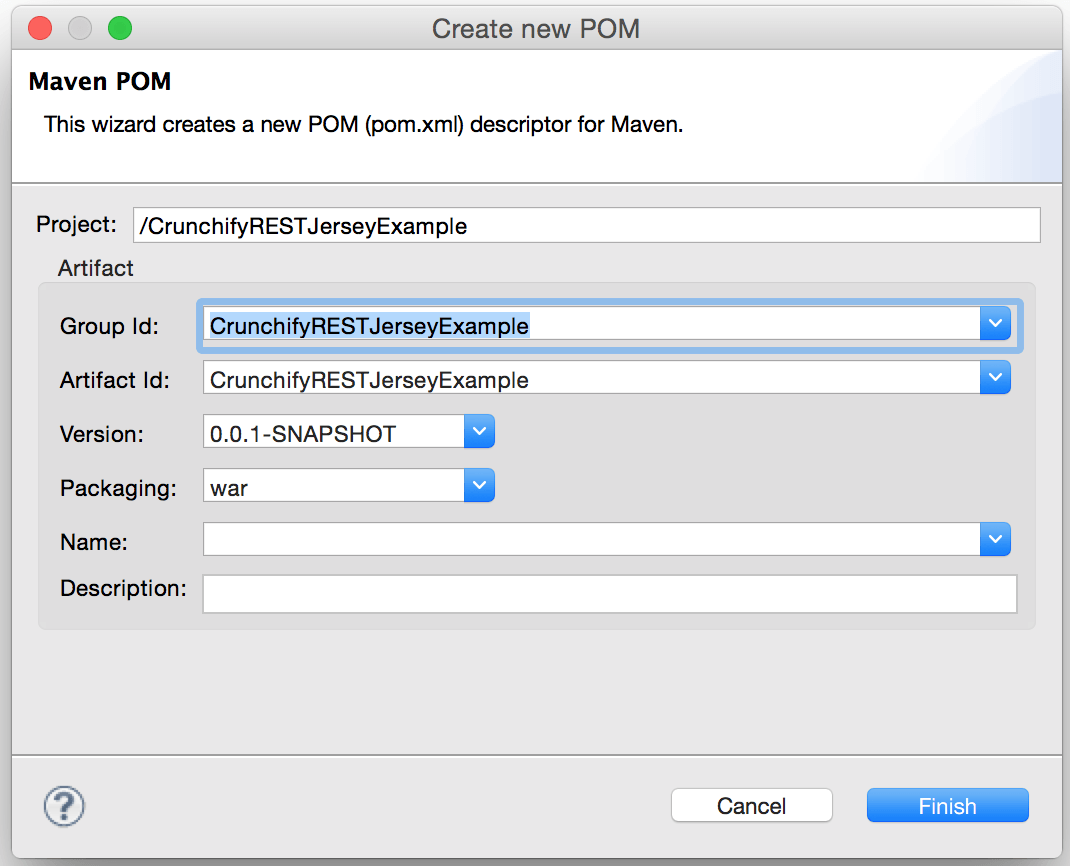
Step-4
Open pom.xml
file and add below dependencies.
- asm.jar
- jersey-bundle.jar
- json.jar
- jersey-server.jar
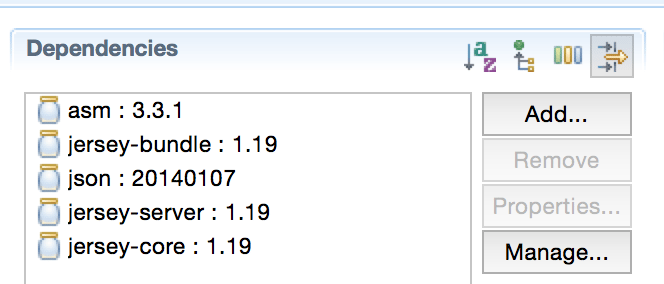
Here is my pom.xml
file.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>CrunchifyRESTJerseyExample</groupId> <artifactId>CrunchifyRESTJerseyExample</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.7.0</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>asm</groupId> <artifactId>asm</artifactId> <version>3.3.1</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-bundle</artifactId> <version>1.19.4</version> </dependency> <dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20170516</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-server</artifactId> <version>1.19.4</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-core</artifactId> <version>1.19.4</version> </dependency> </dependencies> </project>
Step-5
Update your web.xml file with this one. Here is my web.xml
file copy:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>CrunchifyRESTJerseyExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>Jersey Web Application</servlet-name> <servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>Jersey Web Application</servlet-name> <url-pattern>/crunchify/*</url-pattern> </servlet-mapping> </web-app>
Step-6
- Go to
Java Resources
- Click on src
- Right click -> New -> Class
- Package: com.crunchify.restjersey
- Name: CtoFService
CtoFService.java
package com.crunchify.restjersey; /** * @author Crunchify.com * * Description: This program converts unit from Centigrade to Fahrenheit. * Last updated: 12/28/2018 */ import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; @Path("/ctofservice") public class CtoFService { @GET @Produces("application/xml") public String convertCtoF() { Double fahrenheit; Double celsius = 36.8; fahrenheit = ((celsius * 9) / 5) + 32; String result = "@Produces(\"application/xml\") Output: \n\nC to F Converter Output: \n\n" + fahrenheit; return "<ctofservice>" + "<celsius>" + celsius + "</celsius>" + "<ctofoutput>" + result + "</ctofoutput>" + "</ctofservice>"; } @Path("{c}") @GET @Produces("application/xml") public String convertCtoFfromInput(@PathParam("c") Double c) { Double fahrenheit; Double celsius = c; fahrenheit = ((celsius * 9) / 5) + 32; String result = "@Produces(\"application/xml\") Output: \n\nC to F Converter Output: \n\n" + fahrenheit; return "<ctofservice>" + "<celsius>" + celsius + "</celsius>" + "<ctofoutput>" + result + "</ctofoutput>" + "</ctofservice>"; } }
Step-7
Same way create FtoCService.java
FtoCService.java
package com.crunchify.restjersey; /** * @author Crunchify, LLC * Description: This program converts unit from Fahrenheit to Centigrade. * */ import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.Response; import org.json.JSONException; import org.json.JSONObject; @Path("/ftocservice") public class FtoCService { @GET @Produces("application/json") public Response convertFtoC() throws JSONException { JSONObject jsonObject = new JSONObject(); Double fahrenheit = 98.24; Double celsius; celsius = (fahrenheit - 32) * 5 / 9; jsonObject.put("F Value", fahrenheit); jsonObject.put("C Value", celsius); String result = "@Produces(\"application/json\") Output: \n\nF to C Converter Output: \n\n" + jsonObject; return Response.status(200).entity(result).build(); } @Path("{f}") @GET @Produces("application/json") public Response convertFtoCfromInput(@PathParam("f") float f) throws JSONException { JSONObject jsonObject = new JSONObject(); float celsius; celsius = (f - 32) * 5 / 9; jsonObject.put("F Value", f); jsonObject.put("C Value", celsius); String result = "@Produces(\"application/json\") Output: \n\nF to C Converter Output: \n\n" + jsonObject; return Response.status(200).entity(result).build(); } }
Step-8
Now let’s clean eclipse workspace and build project.
1. Project -> Clean 2. Project -> Right click -> Maven -> Update Project 3. Project -> Right click -> Run As.. -> Maven Build (option 5) -> Add "clean install" -> Run
- For
point 3
above usethese screenshots
: Maven Build, clean install. - You should see build success message.
Step-9
Deploy project CrunchifyRESTJerseyExample
on Tomcat. Here are detailed steps on how to setup Tomcat on Eclipse if you haven’t done so.
- Go to Server Tab
- Right click on Server
- Click on
Add and Remove Projects
- Selection project from left side and click on
Add
- Click finish
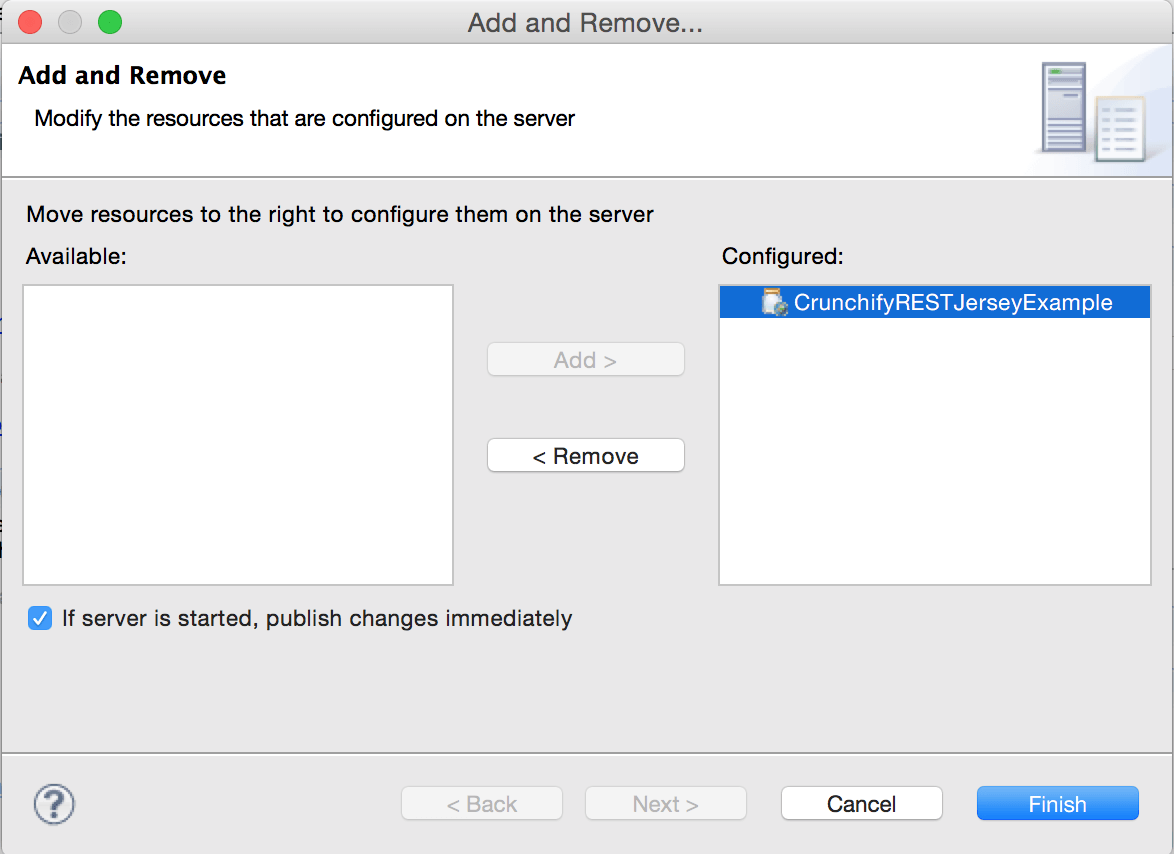
Step-10
- Start Tomcat Server 9.0
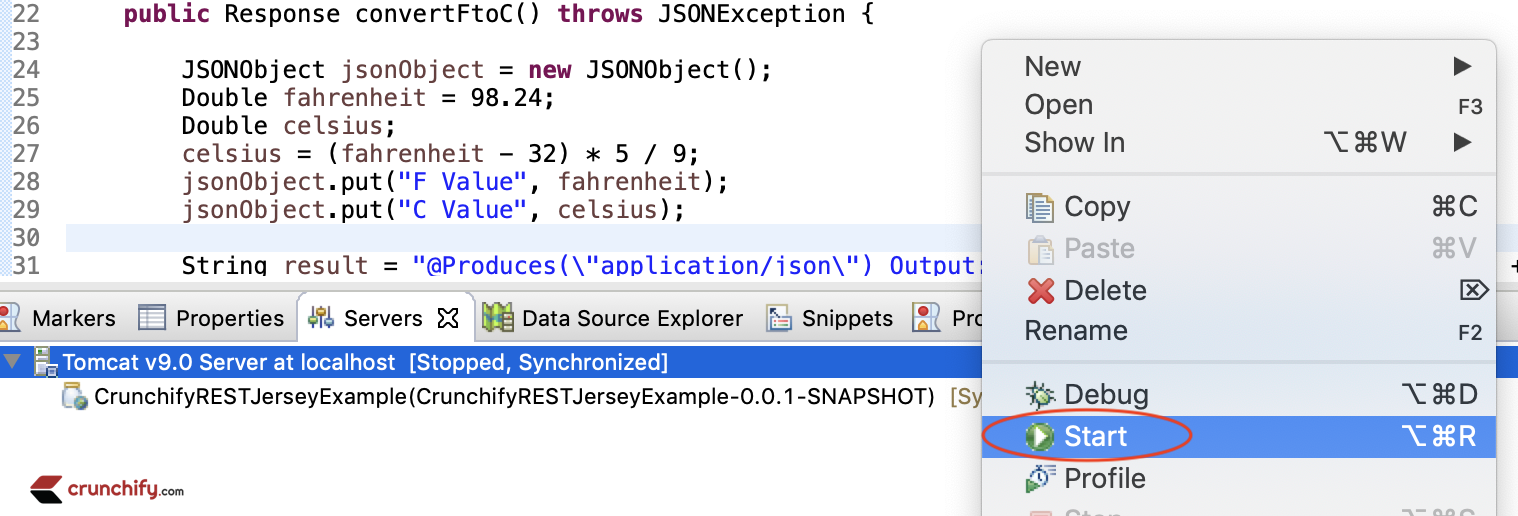
Complete project structure:
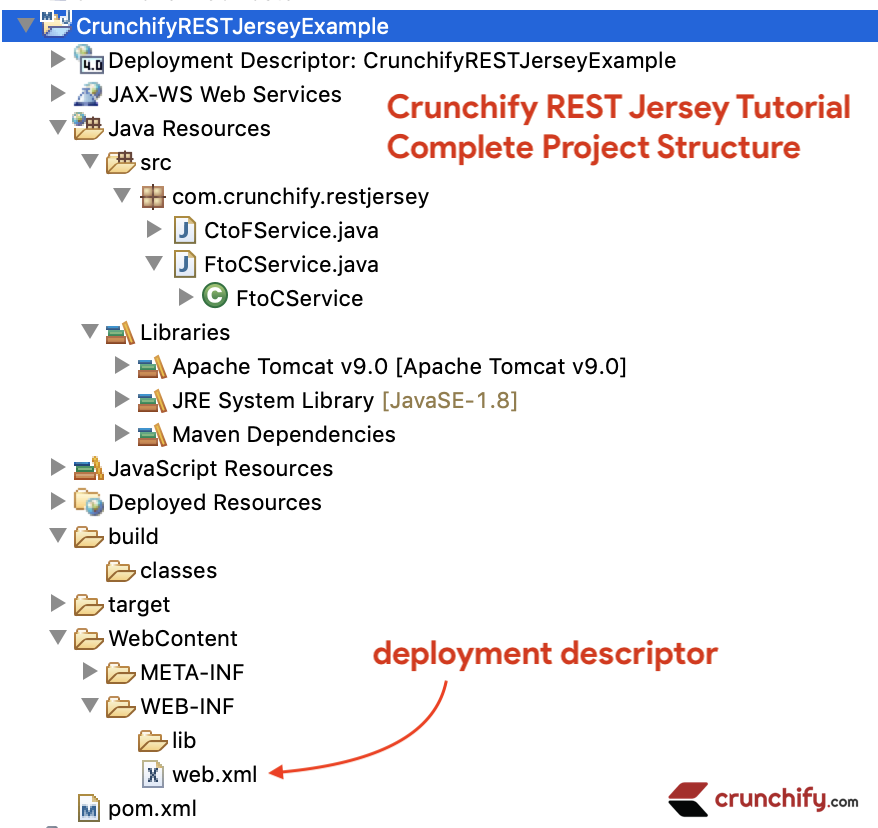
Here are Java Build Path Dependencies:
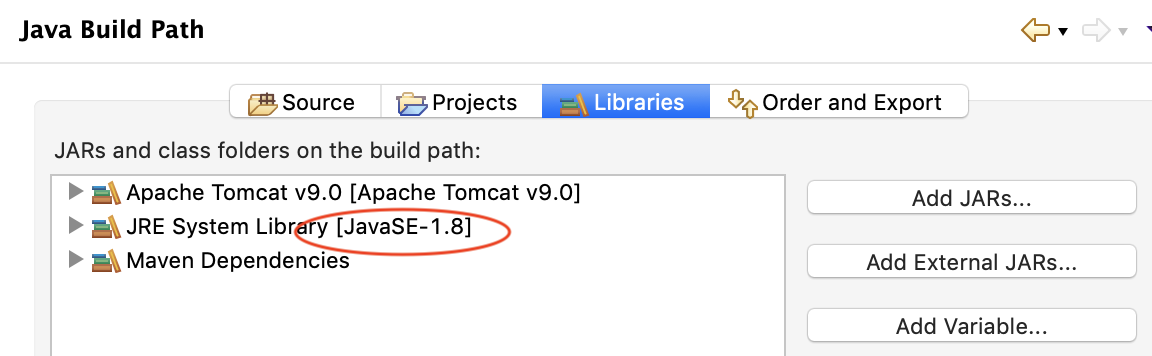
Make sure you use JDK 1.8 for this project. Starting Java 9 – JAXB, JAX-WS, JAF, JTA, CORBA modules are removed and you need to add those modules manually to your Maven pom.xml file.
All set.
Now let’s test you RESTful Web Service.
Test 1: Celsius to Fahrenheit web service without
parameter
Link: http://localhost:8080/CrunchifyRESTJerseyExample/crunchify/ctofservice/
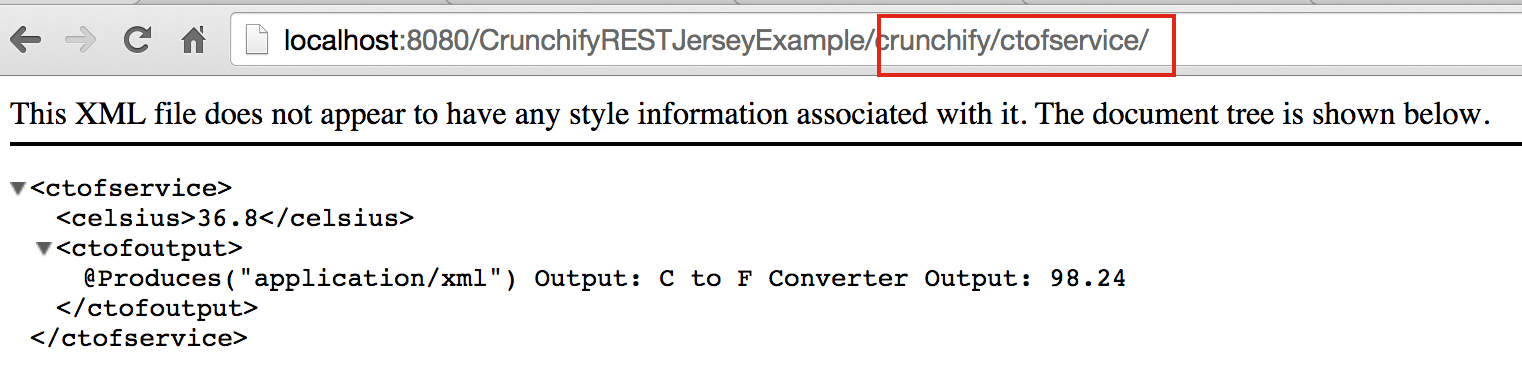
Test 2: Celsius to Fahrenheit web service with
parameter
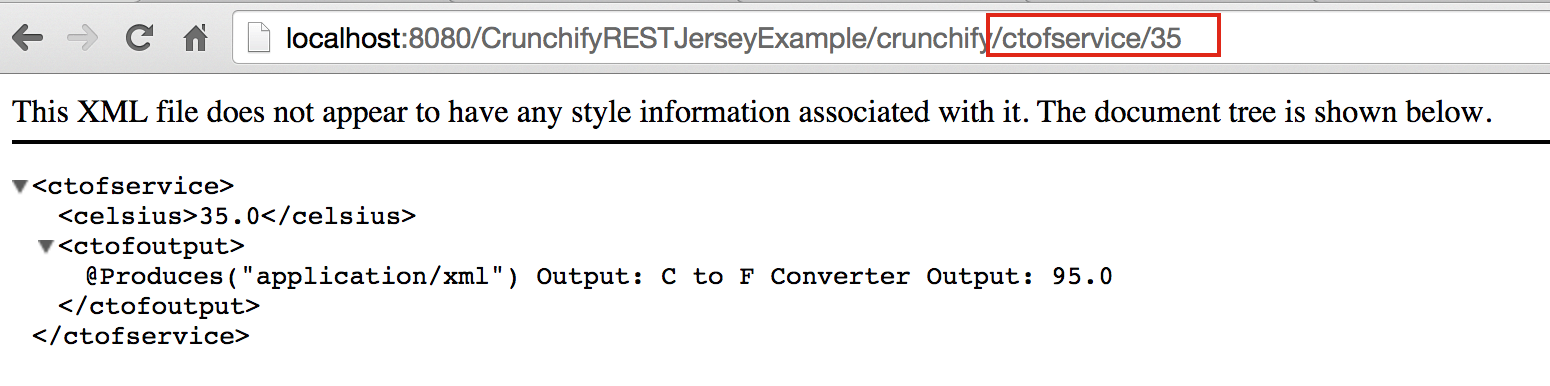
Test 3: Fahrenheit to Celsius web service without
parameter
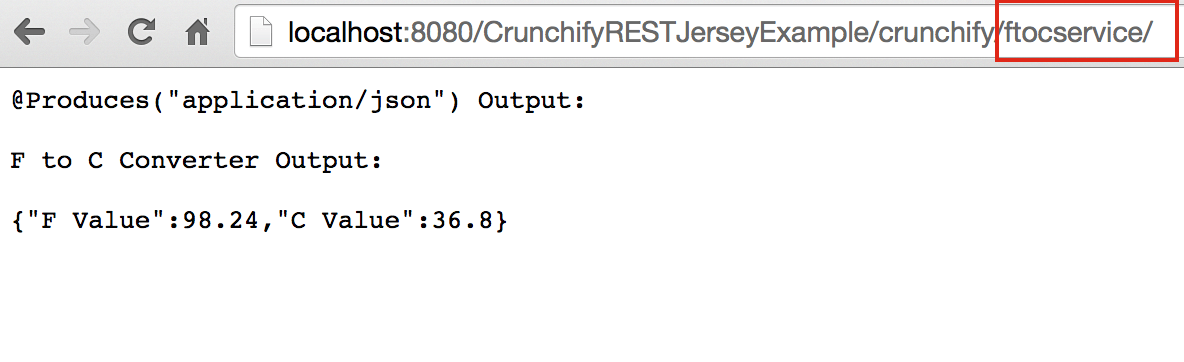
Test 4: Fahrenheit to Celsius web service with
parameter
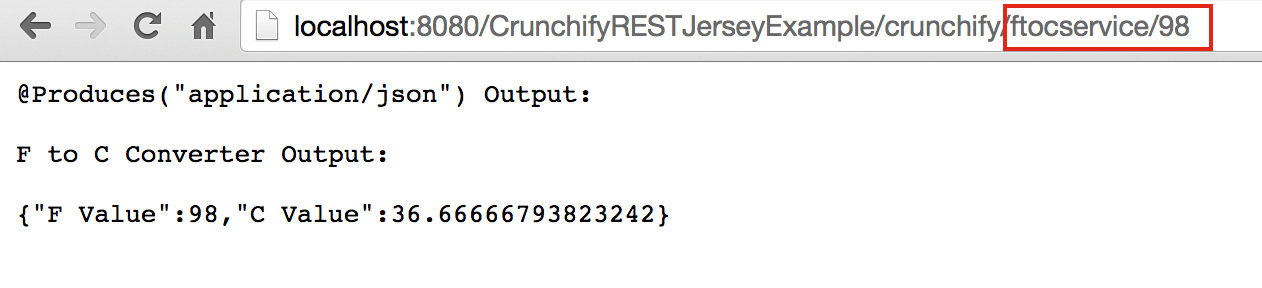
RESTFul Client Examples:
- How to Create RESTful Java Client using Apache HttpClient – Example
- How to Create RESTful Java Client With Java.Net.URL – Example
- How to Create RESTful Java Client With Jersey Client – Example
Another must read:
- CrunchifyJSONtoHTML.js – JSON to HTML table Converter Script
- How to Generate Out Of Memory (OOM) in Java Programatically
- How to Run Multiple Tomcat Instances on One Server?
- How to Read JSON Object From File in Java – Crunchify Tutorial
Having trouble running code?
Some triage steps:
Initially I used jersey-core
dependency. But added jersey-server
dependency too to avoid below issue.
SEVERE: Servlet [Jersey Web Application] in web application [/CrunchifyRESTJerseyExample] threw load() exception java.lang.ClassNotFoundException: com.sun.jersey.spi.container.servlet.ServletContainer at org.apache.catalina.loader.WebappClassLoaderBase.loadClass(WebappClassLoaderBase.java:1328) at org.apache.catalina.loader.WebappClassLoaderBase.loadClass(WebappClassLoaderBase.java:1157) at org.apache.catalina.core.DefaultInstanceManager.loadClass(DefaultInstanceManager.java:542) at org.apache.catalina.core.DefaultInstanceManager.loadClassMaybePrivileged(DefaultInstanceManager.java:523) at org.apache.catalina.core.DefaultInstanceManager.newInstance(DefaultInstanceManager.java:150) at org.apache.catalina.core.StandardWrapper.loadServlet(StandardWrapper.java:1032) at org.apache.catalina.core.StandardWrapper.load(StandardWrapper.java:971) at org.apache.catalina.core.StandardContext.loadOnStartup(StandardContext.java:4829) at org.apache.catalina.core.StandardContext.startInternal(StandardContext.java:5143) at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:183) at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1432) at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1422) at java.base/java.util.concurrent.FutureTask.run(FutureTask.java:264) at org.apache.tomcat.util.threads.InlineExecutorService.execute(InlineExecutorService.java:75) at java.base/java.util.concurrent.AbstractExecutorService.submit(AbstractExecutorService.java:140) at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:944) at org.apache.catalina.core.StandardHost.startInternal(StandardHost.java:831) at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:183) at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1432) at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1422) at java.base/java.util.concurrent.FutureTask.run(FutureTask.java:264) at org.apache.tomcat.util.threads.InlineExecutorService.execute(InlineExecutorService.java:75) at java.base/java.util.concurrent.AbstractExecutorService.submit(AbstractExecutorService.java:140) at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:944) at org.apache.catalina.core.StandardEngine.startInternal(StandardEngine.java:261) at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:183) at org.apache.catalina.core.StandardService.startInternal(StandardService.java:422) at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:183) at org.apache.catalina.core.StandardServer.startInternal(StandardServer.java:801) at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:183) at org.apache.catalina.startup.Catalina.start(Catalina.java:695) at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.base/java.lang.reflect.Method.invoke(Method.java:566) at org.apache.catalina.startup.Bootstrap.start(Bootstrap.java:350) at org.apache.catalina.startup.Bootstrap.main(Bootstrap.java:492)
If you are getting above error then try adding below maven dependency into your pom.xml file
<dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-server</artifactId> <version>1.17.1</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-core</artifactId> <version>1.17.1</version> </dependency> <dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-servlet</artifactId> <version>1.17.1</version> </dependency>