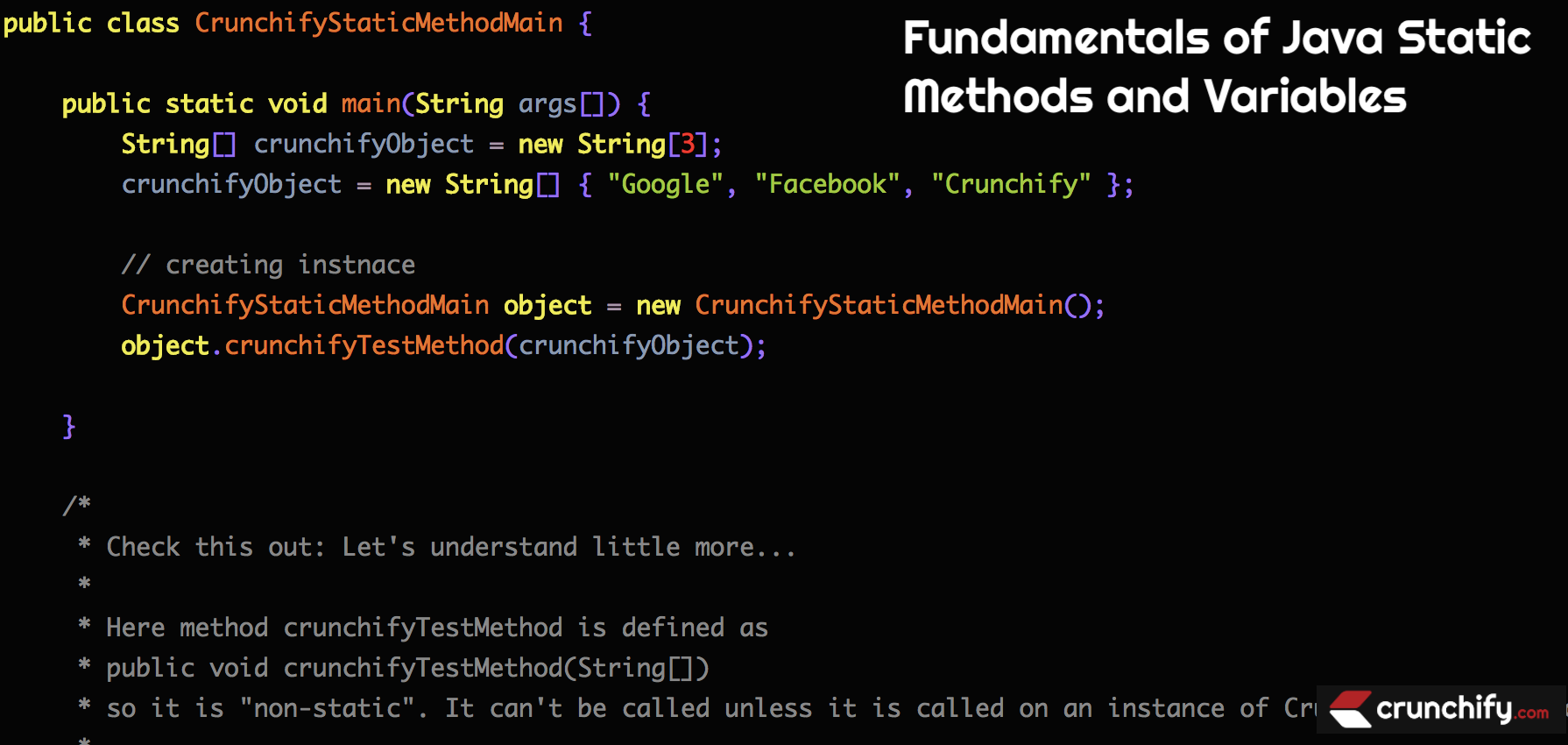
What is static in Java?
- Static keyword can be used with class, variable, method and block.
- Static members doesn’t belong to any of a specific instance.
- Static members belong to the class only.
- Once you make a member static, you can access it without any object.
Do you have any of below questions?
- Could you write down Java static methods best practices?
- What is Java static methods in interface?
- Have a question on Java static methods vs singleton?
- Java static methods vs instance methods performance
- Java static methods and variables
- Java static methods vs non static
- Java static methods in abstract class
The static keyword can be used in 3 scenarios:
- Static variables
- Static methods
- Static blocks of code.
static variable (“static” Keyword = Class Variables)
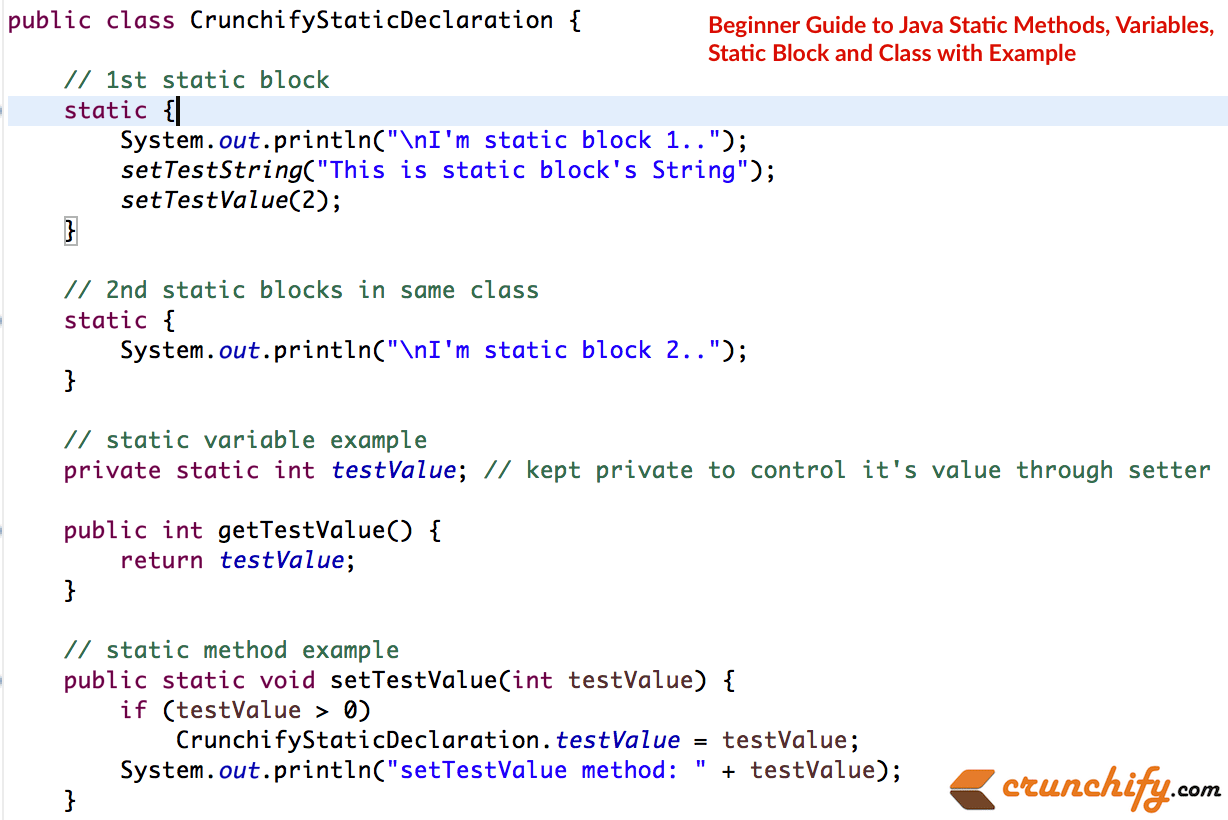
In Java Variables can be declared with the “static
” keyword.
Example: static int y = 0;
When a variable is declared with the keyword static
, it’s called a class variable
. All instances share the same copy of the variable. A class variable can be accessed directly with the class, without the need to create a instance.
No “static” Keyword = Instance Variables
Without the static keyword
, it’s called instance variable
, and each instance of the class has its own copy of the variable.
Example: static int crunchify_variable_name
;
- The static variables are shared among all the instances of the class.
- One of the main reason you need it when you want to do lots of memory management.
- For all static variable – there will be only one single copy available for you to use.
- You absolutely don’t need an class object in order to access static variable.
- Just use it directly. You don’t need
object.StaticVariable
🙂
- Just use it directly. You don’t need
What is Static block?
The static block, is a block of statement inside a Java class
that will be executed when a class is first loaded into the JVM.
Can you override private method in Java?
- Well, no. Private methods can’t be override as it’s not available to use outside of class.
Why can’t we override static methods in Java?
- Static methods also can’t be overridden as it’s part of a class rather than an object 🙂
Can we access non static variable in static context?
- To be able to access non static variable from your static methods they need to be static member variables.
Details on Static method
- How to identify? Just check this first. Do you need an class object to access static method? If you don’t need an object then it’s Static method.
- You need to use static keyword in order to specify static method
- Better use keyword static if that method is not going to change throughout your project at runtime.
- You can’t override static method.
Let’s take a look at below Examples:
Example-1:
package crunchify.com.tutorials; /** * @author Crunchify.com * */ public class CrunchifyStaticMethodMain { public static void main(String args[]) { String[] crunchifyObject = new String[3]; crunchifyObject = new String[] { "Google", "Facebook", "Crunchify" }; // creating instnace CrunchifyStaticMethodMain object = new CrunchifyStaticMethodMain(); object.crunchifyTestMethod(crunchifyObject); } /* * Check this out: Let's understand little more... * * Here method crunchifyTestMethod is defined as * public void crunchifyTestMethod(String[]) * so it is "non-static". It can't be called unless it is called on an instance of CrunchifyStaticMethodMain. * * If you declared your method as * public static void crunchifyTestMethod(int[]) * then you could call: CrunchifyStaticMethodMain.crunchifyTestMethod(arr); within main without having created an instance for it. */ public void crunchifyTestMethod(String[] crunchifyObject) { for (int i = 0; i < crunchifyObject.length; i++) System.out.println(crunchifyObject[i]); } }
Check it out the explanation in above code block
. We have to create instance of Class to access non-static method.
Example-2:
package com.crunchify.tutorials; /** * @author Crunchify.com */ public class CrunchifyStaticDeclaration { // 1st static block static { System.out.println("\nI'm static block 1.."); setTestString("This is static block's String"); setTestValue(2); } // 2nd static blocks in same class static { System.out.println("\nI'm static block 2.."); } // static variable example private static int testValue; // kept private to control it's value through setter public int getTestValue() { return testValue; } // static method example public static void setTestValue(int testValue) { if (testValue > 0) CrunchifyStaticDeclaration.testValue = testValue; System.out.println("setTestValue method: " + testValue); } public static String testString; /** * @return the testString */ public static String getTestString() { return testString; } /** * @param testString the testString to set */ public static void setTestString(String testString) { CrunchifyStaticDeclaration.testString = testString; System.out.println("setTestString method: " + testString); } // static util method public static int subValue(int i, int... js) { int sum = i; for (int x : js) sum -= x; return sum; } }
Now let’s do test:
package com.crunchify.tutorials; /** * @author Crunchify.com */ public class CrunchifyStaticExample { public static void main(String[] args) { CrunchifyStaticDeclaration.setTestValue(5); // non-private static variables can be accessed with class name CrunchifyStaticDeclaration.testString = "\nAssigning testString a value"; CrunchifyStaticDeclaration csd = new CrunchifyStaticDeclaration(); System.out.println(csd.getTestString()); // class and instance static variables are same System.out.print("\nCheck if Class and Instance Static Variables are same: "); System.out.println(CrunchifyStaticDeclaration.testString == csd.testString); System.out.println("Why? Because: CrunchifyStaticDeclaration.testString == csd.testString"); } }
Output:
I'm static block 1.. setTestString method: This is static block's String setTestValue method: 2 I'm static block 2.. setTestValue method: 5 Assigning testString a value Check if Class and Instance Static Variables are same: true Why? Because: CrunchifyStaticDeclaration.testString == csd.testString
I hope you really get good information about Static method, variable and blocks.
Let us know if you face any issue running above program or having an issue understanding Static keyword in Java.