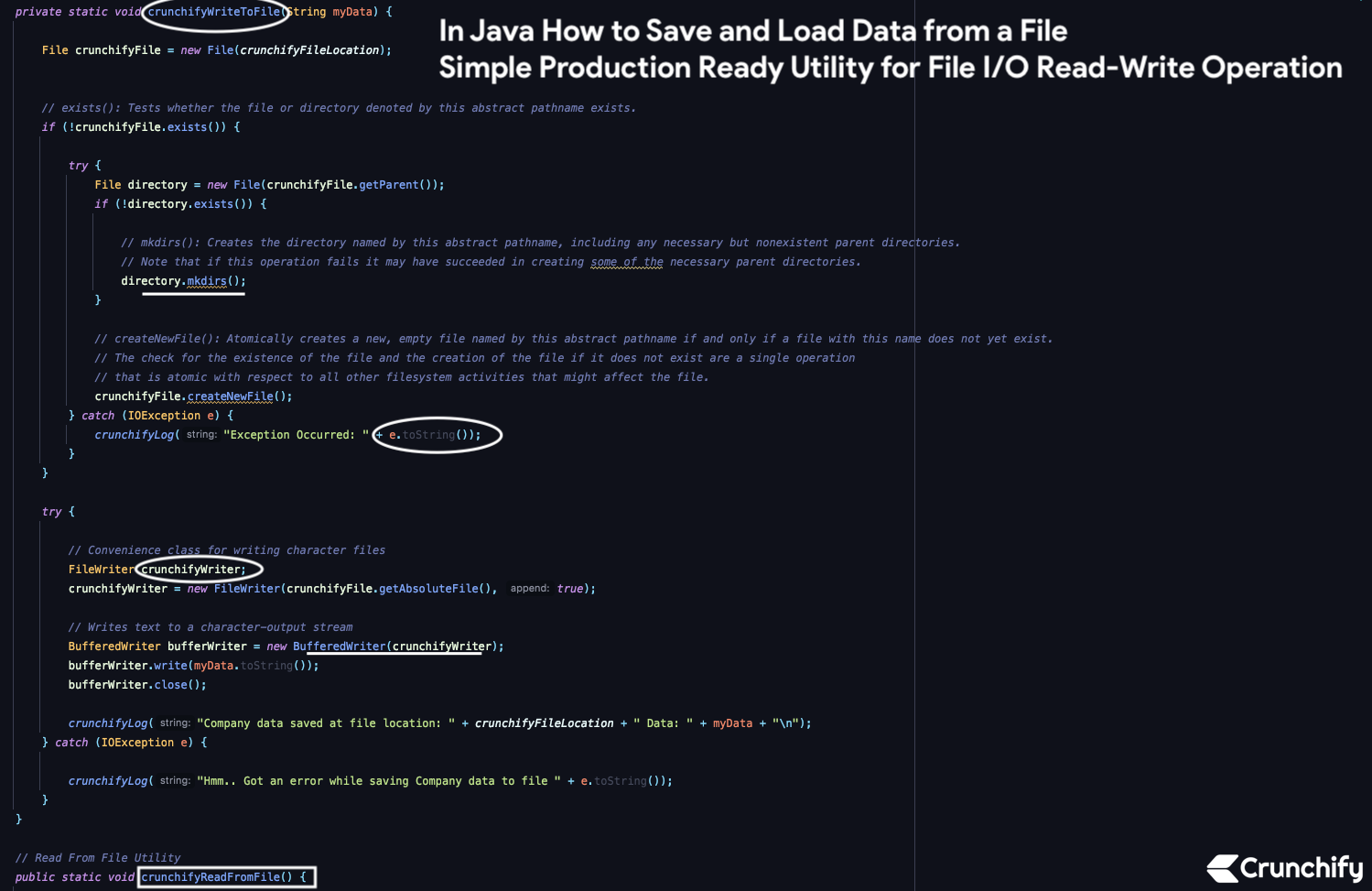
How do I write an object to a file and read it back?
Java is pretty amazing with lots of API and with Java 8 we are fully enabled with lots more APIs like Lambda, Method reference, Default methods, Better type interface, Repeating annotations, Method parameter reflections and lot more.
Sometime back I’ve written an article on How to Read JSON Object From File in Java. It was simple java read operation. But in this tutorial we are going to save and load
data from file with simple Production Ready Java Utility.
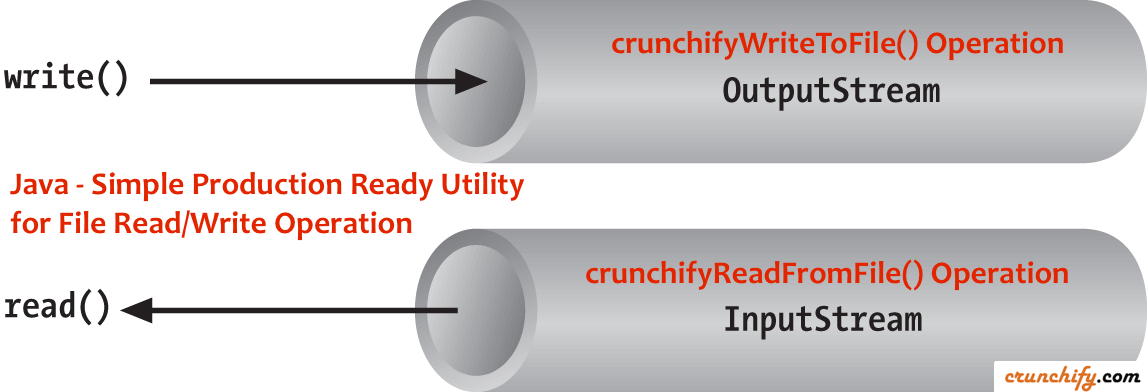
We are not only saving simple object but we will create simple Java POJO of type CrunchifyCompany and going to save and retrieve object using GSON
. You need below dependency in order for below program to run.
Put below dependency to your maven project. If you have Dynamic Web Project and want to convert it into Maven project then follow these steps.
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.3</version> </dependency>
Here is a flow:
- Create class
CrunchifyReadWriteUtilityForFile.java
- Create private inner class
CrunchifyCompany
with two fields- private int
employees
; - private String
companyName
;
- private int
- Create object
crunchify
inside main method - Convert object to
Gson
so it will be saved to file - Use method
crunchifyWriteToFile
to save data to file in Java - Use method
crunchifyReadFromFile
to retrieve data from file in Java
Here is a complete example:
package crunchify.com.tutorial; import com.google.gson.Gson; import com.google.gson.stream.JsonReader; import java.io.*; import java.nio.charset.StandardCharsets; /** * @author Crunchify.com * Best and simple Production ready utility to save/load * (read/write) data from/to file */ public class CrunchifyReadWriteUtilityForFile { private static final String crunchifyFileLocation = "/Users/appshah/Documents/crunchify.txt"; private static final Gson gson = new Gson(); // CrunchifyComapny Class with two fields // - Employees // - CompanyName private static class CrunchifyCompany { private int employees; private String companyName; public int getEmployees() { return employees; } public void setEmployees(int employees) { this.employees = employees; } public String getCompanyName() { return companyName; } public void setCompanyName(String companyName) { this.companyName = companyName; } } // Main Method public static void main(String[] args) { CrunchifyCompany crunchify = new CrunchifyCompany(); crunchify.setCompanyName("Crunchify.com"); crunchify.setEmployees(4); // Save data to file crunchifyWriteToFile(gson.toJson(crunchify)); // Retrieve data from file crunchifyReadFromFile(); } // Save to file Utility private static void crunchifyWriteToFile(String myData) { File crunchifyFile = new File(crunchifyFileLocation); // exists(): Tests whether the file or directory denoted by this abstract pathname exists. if (!crunchifyFile.exists()) { try { File directory = new File(crunchifyFile.getParent()); if (!directory.exists()) { // mkdirs(): Creates the directory named by this abstract pathname, including any necessary but nonexistent parent directories. // Note that if this operation fails it may have succeeded in creating some of the necessary parent directories. directory.mkdirs(); } // createNewFile(): Atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. // The check for the existence of the file and the creation of the file if it does not exist are a single operation // that is atomic with respect to all other filesystem activities that might affect the file. crunchifyFile.createNewFile(); } catch (IOException e) { crunchifyLog("Exception Occurred: " + e.toString()); } } try { // Convenience class for writing character files FileWriter crunchifyWriter; crunchifyWriter = new FileWriter(crunchifyFile.getAbsoluteFile(), true); // Writes text to a character-output stream BufferedWriter bufferWriter = new BufferedWriter(crunchifyWriter); bufferWriter.write(myData.toString()); bufferWriter.close(); crunchifyLog("Company data saved at file location: " + crunchifyFileLocation + " Data: " + myData + "\n"); } catch (IOException e) { crunchifyLog("Hmm.. Got an error while saving Company data to file " + e.toString()); } } // Read From File Utility public static void crunchifyReadFromFile() { // File: An abstract representation of file and directory pathnames. // User interfaces and operating systems use system-dependent pathname strings to name files and directories. File crunchifyFile = new File(crunchifyFileLocation); if (!crunchifyFile.exists()) crunchifyLog("File doesn't exist"); InputStreamReader isReader; try { isReader = new InputStreamReader(new FileInputStream(crunchifyFile), StandardCharsets.UTF_8); JsonReader myReader = new JsonReader(isReader); CrunchifyCompany company = gson.fromJson(myReader, CrunchifyCompany.class); crunchifyLog("Company Name: " + company.getCompanyName()); int employee = company.getEmployees(); crunchifyLog("# of Employees: " + Integer.toString(employee)); } catch (Exception e) { crunchifyLog("error load cache from file " + e.toString()); } crunchifyLog("\nCompany Data loaded successfully from file " + crunchifyFileLocation); } private static void crunchifyLog(String string) { System.out.println(string); } }
Eclipse Console Output:
Company data saved at file location: /Users/appshah/Documents/crunchify.txt Data: {"employees":4,"companyName":"Crunchify.com"} Company Name: Crunchify.com # of Employees: 4 Company Data loaded successfully from file /Users/appshah/Documents/crunchify.txt
Here is a crunchify.txt file content.
As I ran program two times
you see here JSONObject two times as we are appending value to crunchify.txt
file.
