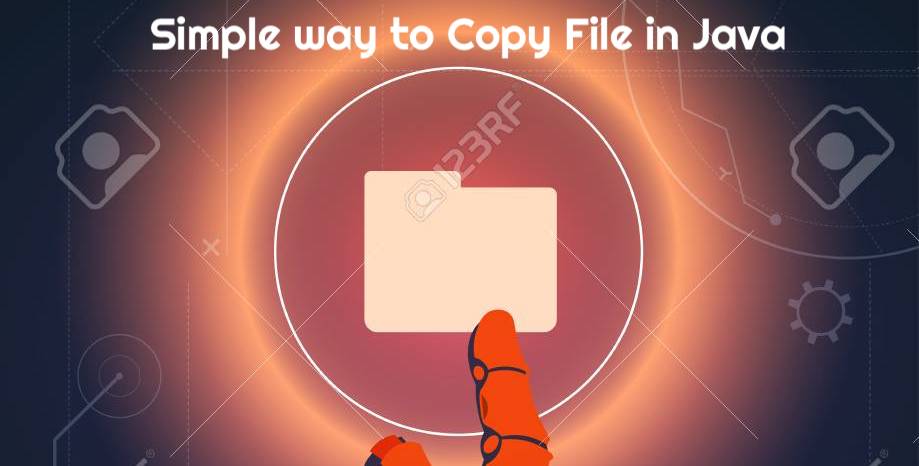
This example illustrates how to copy contents from one file to another file. This topic is related to the I/O (input/output) of java.io.File package.
Java.io class represents a file name of the host file system.
The file name can be relative or absolute. It must use the file name conventions of the host platform.
The intention is to provide an abstraction that deals with most of the system-dependent file name features such as the separator character, root, device name, etc.
Note that whenever a file name or path is used it is assumed that the host’s file name conventions are used.
Here is a complete Java file:
package com.crunchify; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; /** * @author Crunchify.com */ public class CrunchifyJavaCopyFunction { public static void main(String[] args) { InputStream inStream = null; OutputStream outStream = null; try{ File file1 =new File("/Users/<username>/Documents/file1.txt"); File file2 =new File("/Users/<username>/Documents/file2.txt"); inStream = new FileInputStream(file1); outStream = new FileOutputStream(file2); // for override file content //outStream = new FileOutputStream(file2,true); // for append file content byte[] buffer = new byte[1024]; int length; while ((length = inStream.read(buffer)) > 0){ outStream.write(buffer, 0, length); } if (inStream != null)inStream.close(); if (outStream != null)outStream.close(); System.out.println("File Copied.."); }catch(IOException e){ e.printStackTrace(); } } }
Console output result:
File Copied.. Process finished with exit code 0