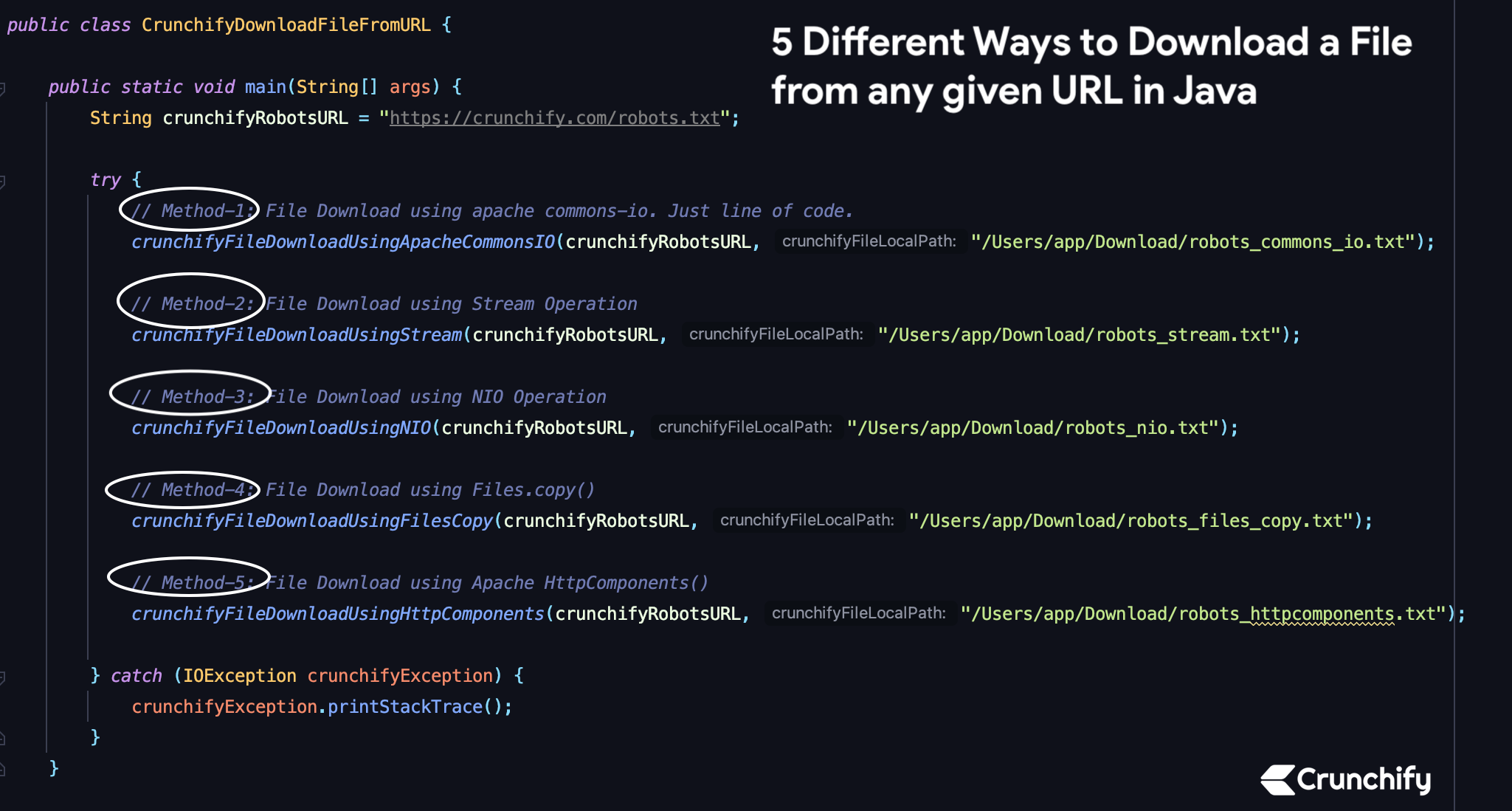
In Java how to download files from any given URL? This tutorial works if you have any of below questions:
- Download a file from a URL in Java.
- How to Download a File from a URL in Java
- How to download and save a file from Internet using Java
- java download file from url Code Example
There are 5 different ways you could download files from any given URL in Java.
- File Download using apache commons-io. Single line of code.
- File Download using Stream Operation
- File Download using NIO Operation
- File Download using Files.copy()
- File Download using Apache HttpComponents()
Create java class: CrunchifyDownloadFileFromURL.java
package crunchify.com.tutorial; import org.apache.commons.io.FileUtils; import org.apache.http.HttpEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import java.io.*; import java.net.URI; import java.net.URL; import java.nio.channels.Channels; import java.nio.channels.ReadableByteChannel; import java.nio.file.Files; import java.nio.file.Paths; import java.nio.file.StandardCopyOption; /** * @author Crunchify.com * <p> * In Java How to Download file from any given URL? * <p> * 5 different ways: * 1. File Download using apache commons-io. Just line of code * 2. File Download using Stream Operation * 3. File Download using NIO Operation * 4. File Download using Files.copy() * 5. File Download using Apache HttpComponents() */ public class CrunchifyDownloadFileFromURL { public static void main(String[] args) { String crunchifyRobotsURL = "https://crunchify.com/robots.txt"; try { // Method-1: File Download using apache commons-io. Just line of code. crunchifyFileDownloadUsingApacheCommonsIO(crunchifyRobotsURL, "/Users/app/Download/robots_commons_io.txt"); // Method-2: File Download using Stream Operation crunchifyFileDownloadUsingStream(crunchifyRobotsURL, "/Users/app/Download/robots_stream.txt"); // Method-3: File Download using NIO Operation crunchifyFileDownloadUsingNIO(crunchifyRobotsURL, "/Users/app/Download/robots_nio.txt"); // Method-4: File Download using Files.copy() crunchifyFileDownloadUsingFilesCopy(crunchifyRobotsURL, "/Users/app/Download/robots_files_copy.txt"); // Method-5: File Download using Apache HttpComponents() crunchifyFileDownloadUsingHttpComponents(crunchifyRobotsURL, "/Users/app/Download/robots_httpcomponents.txt"); } catch (IOException crunchifyException) { crunchifyException.printStackTrace(); } } // Method-1: Using apache commons-io. Just single line of code. // FileUtils.copyURLToFile(URL, File); private static void crunchifyFileDownloadUsingApacheCommonsIO(String crunchifyURL, String crunchifyFileLocalPath) { URL crunchifyRobotsURL = null; try { crunchifyRobotsURL = new URL(crunchifyURL); FileUtils.copyURLToFile(crunchifyRobotsURL, new File(crunchifyFileLocalPath)); } catch (IOException e) { e.printStackTrace(); } printResult("File Downloaded Successfully with apache commons-io Operation \n"); } // Method-2: File Download using Stream Operation private static void crunchifyFileDownloadUsingStream(String crunchifyURL, String crunchifyFileLocalPath) throws IOException { URL crunchifyRobotsURL = new URL(crunchifyURL); // BufferedInputStream(): Creates a BufferedInputStream and saves its argument, the input stream in, for later use. An internal buffer array is created and stored in buf. BufferedInputStream crunchifyInputStream = new BufferedInputStream(crunchifyRobotsURL.openStream()); FileOutputStream crunchifyOutputStream = new FileOutputStream(crunchifyFileLocalPath); byte[] crunchifySpace = new byte[2048]; int crunchifyCounter = 0; while ((crunchifyCounter = crunchifyInputStream.read(crunchifySpace, 0, 1024)) != -1) { crunchifyOutputStream.write(crunchifySpace, 0, crunchifyCounter); } crunchifyOutputStream.close(); crunchifyInputStream.close(); printResult("File Downloaded Successfully with Java Stream Operation \n"); } // Method-3: File Download using NIO Operation private static void crunchifyFileDownloadUsingNIO(String crunchifyURL, String crunchifyFileLocalPath) throws IOException { URL crunchifyRobotsURL = new URL(crunchifyURL); // ReadableByteChannel(): A channel that can read bytes. Only one read operation upon a readable channel may be in progress at any given time. If one thread initiates a read operation upon a channel then any other thread that attempts to initiate another read operation will block until the first operation is complete. // Whether or not other kinds of I/O operations may proceed concurrently with a read operation depends upon the type of the channel. // openStream(): Opens a connection to this URL and returns an InputStream for reading from that connection. ReadableByteChannel crunchifyByteChannel = Channels.newChannel(crunchifyRobotsURL.openStream()); // FileOutputStream(): A file output stream is an output stream for writing data to a File or to a FileDescriptor. Whether or not a file is available or may be created depends upon the underlying platform. Some platforms, in particular, allow a file to be opened for writing by only one FileOutputStream (or other file-writing object) at a time. // In such situations the constructors in this class will fail if the file involved is already open. FileOutputStream crunchifyOutputStream = new FileOutputStream(crunchifyFileLocalPath); // getChannel(): Returns the unique FileChannel object associated with this file output stream. // transferFrom(): Transfers bytes into this channel's file from the given readable byte channel. // An attempt is made to read up to count bytes from the source channel and write them to this channel's file starting at the given position. An invocation of this method may or may not transfer all of the requested bytes; whether or not it does so depends upon the natures and states of the channels. // Fewer than the requested number of bytes will be transferred if the source channel has fewer than count bytes remaining, or if the source channel is non-blocking and has fewer than count bytes immediately available in its input buffer. crunchifyOutputStream.getChannel().transferFrom(crunchifyByteChannel, 0, Long.MAX_VALUE); // A constant holding the maximum value a long can have. // Closes this file output stream and releases any system resources associated with this stream. This file output stream may no longer be used for writing bytes. crunchifyOutputStream.close(); // Closes this channel. After a channel is closed, any further attempt to invoke I/O operations upon it will cause a ClosedChannelException to be thrown. crunchifyByteChannel.close(); printResult("File Downloaded Successfully with Java NIO ReadableByteChannel Operation \n"); } // Method-4: File Download using Files.copy() private static void crunchifyFileDownloadUsingFilesCopy(String crunchifyURL, String crunchifyFileLocalPath) { // URI Creates a URI by parsing the given string. // This convenience factory method works as if by invoking the URI(String) constructor; // any URISyntaxException thrown by the constructor is caught and wrapped in a new IllegalArgumentException object, which is then thrown. try (InputStream crunchifyInputStream = URI.create(crunchifyURL).toURL().openStream()) { // Files: This class consists exclusively of static methods that operate on files, directories, or other types of files. // copy() Copies all bytes from an input stream to a file. On return, the input stream will be at end of stream. // Paths: This class consists exclusively of static methods that return a Path by converting a path string or URI. // Paths.get() Converts a path string, or a sequence of strings that when joined form a path string, to a Path. Files.copy(crunchifyInputStream, Paths.get(crunchifyFileLocalPath), StandardCopyOption.REPLACE_EXISTING); } catch (IOException e) { // printStackTrace: Prints this throwable and its backtrace to the standard error stream. e.printStackTrace(); } printResult("File Downloaded Successfully with Files.copy() Operation \n"); } // Method-5: File Download using Apache HttpComponents() private static void crunchifyFileDownloadUsingHttpComponents(String crunchifyURL, String crunchifyFileLocalPath) { CloseableHttpClient crunchifyHTTPClient = HttpClients.createDefault(); HttpGet crunchifyHTTPGet = new HttpGet(crunchifyURL); CloseableHttpResponse crunchifyHTTPResponse = null; try { crunchifyHTTPResponse = crunchifyHTTPClient.execute(crunchifyHTTPGet); HttpEntity crunchifyHttpEntity = crunchifyHTTPResponse.getEntity(); if (crunchifyHttpEntity != null) { FileUtils.copyInputStreamToFile(crunchifyHttpEntity.getContent(), new File(crunchifyFileLocalPath)); } } catch (IOException e) { // IOException: Signals that an I/O exception of some sort has occurred. // This class is the general class of exceptions produced by failed or interrupted I/O operations. e.printStackTrace(); } printResult("File Downloaded Successfully with Apache HttpComponents() Operation \n"); } // Simple Crunchify Print Utility private static void printResult(String crunchifyResult) { System.out.println(crunchifyResult); } }
Just run above program and you will result as below.
IntelliJ IDEA Console result.
File Downloaded Successfully with apache commons-io Operation File Downloaded Successfully with Java Stream Operation File Downloaded Successfully with Java NIO ReadableByteChannel Operation File Downloaded Successfully with Files.copy() Operation File Downloaded Successfully with Apache HttpComponents() Operation Process finished with exit code 0
Method-1) File Download using apache commons-io
Please make sure to add below maven dependency into your pom.xml file.
<dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.10.0</version> </dependency>
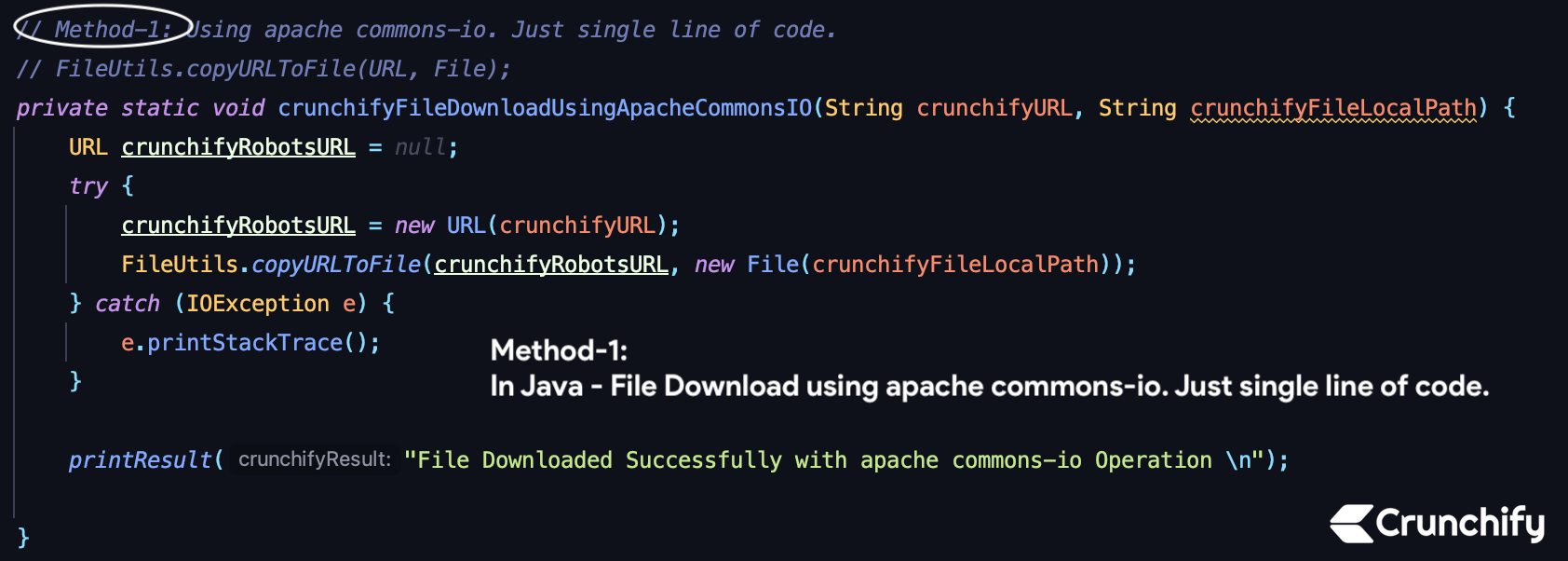
Method-2) File Download using Stream Operation
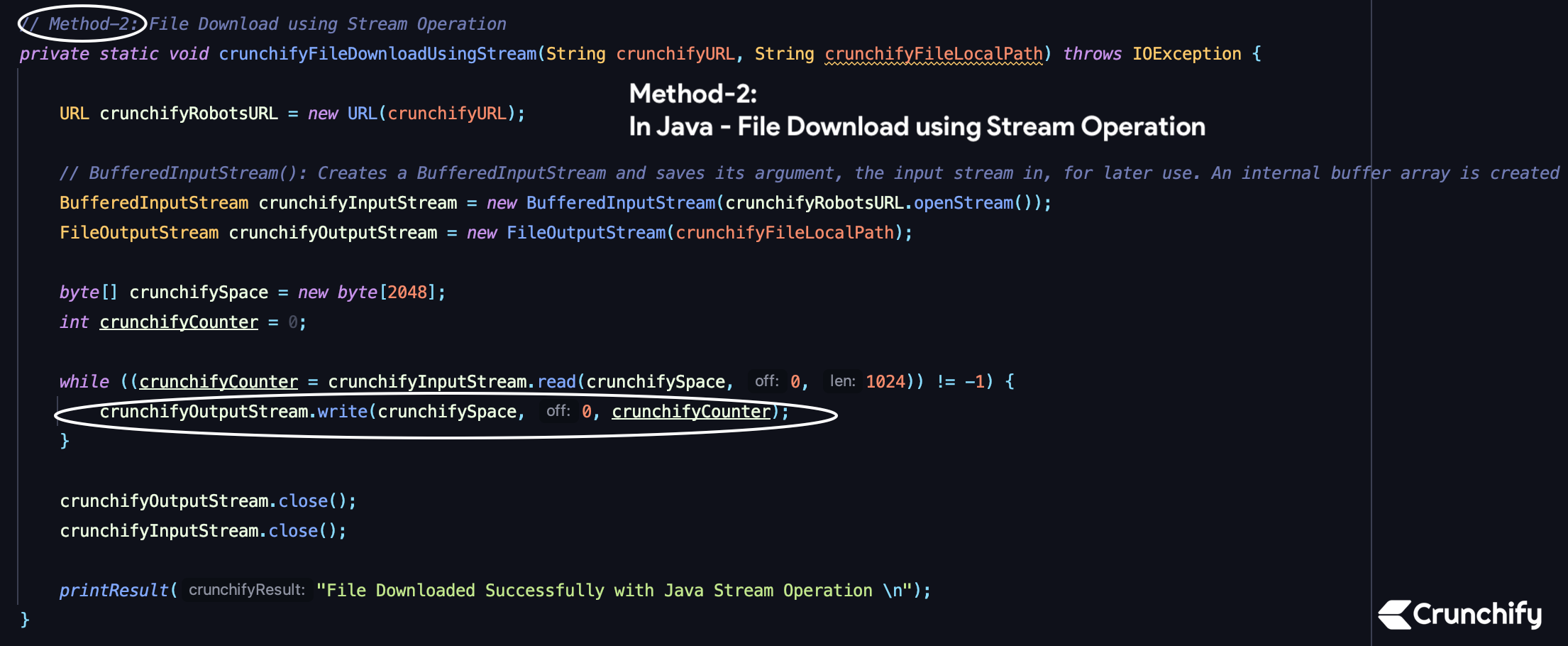
Method-3) File Download using NIO Operation
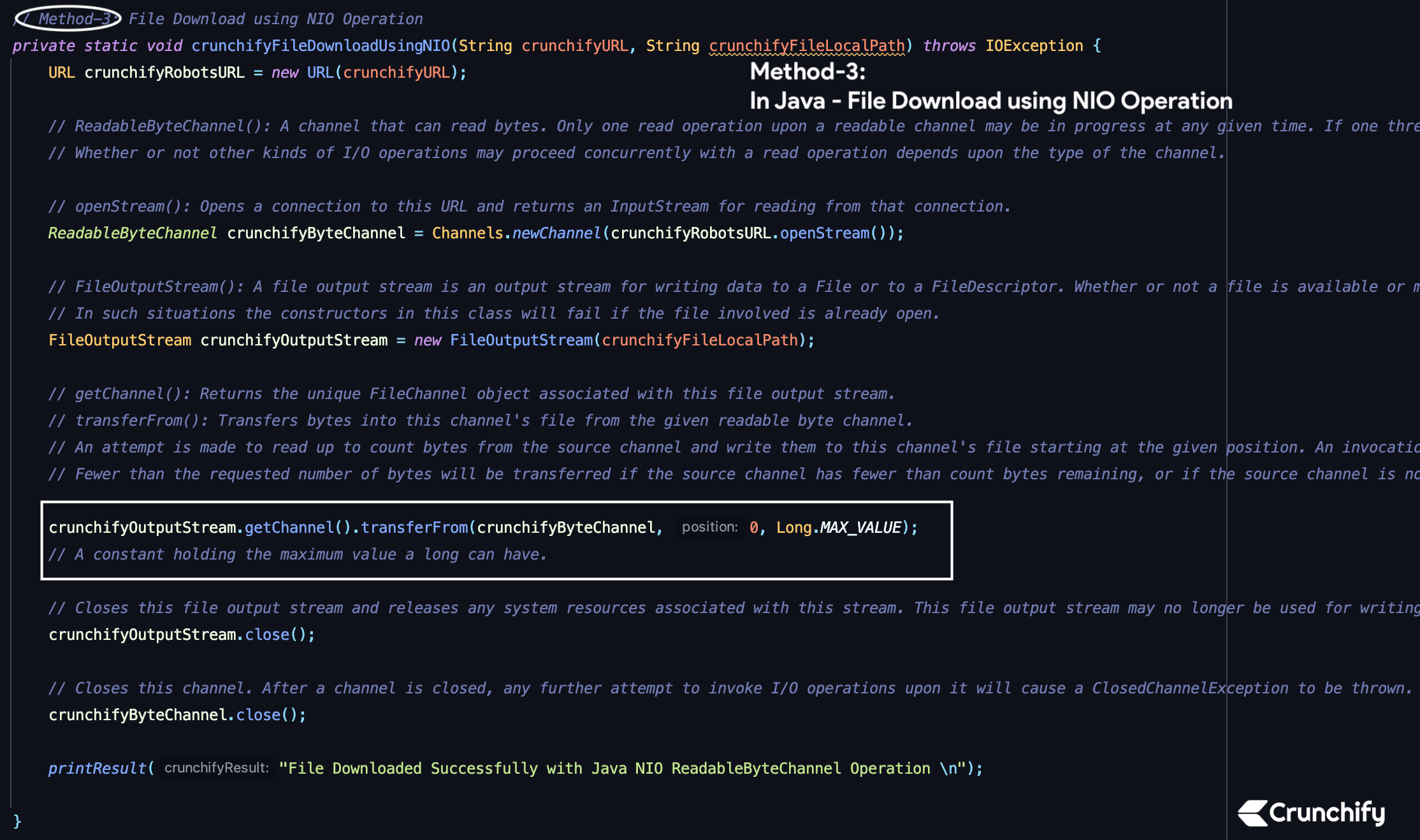
Method-4) File Download using Files.copy()
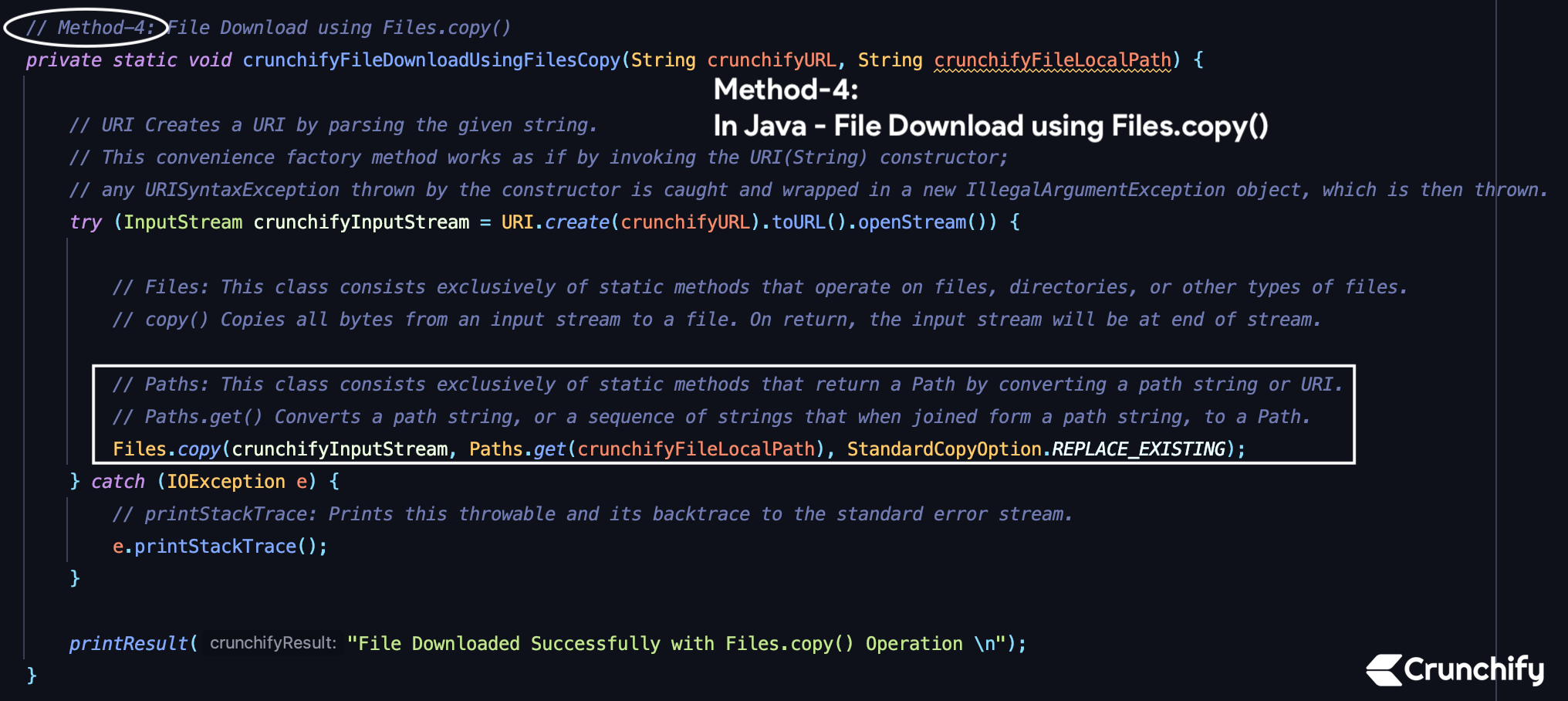
Method-5) File Download using Apache HttpComponents()
Please make sure to add below maven dependency into your pom.xml file.
<dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>com.springsource.org.apache.httpcomponents.httpclient</artifactId> <version>4.2.1</version> </dependency>
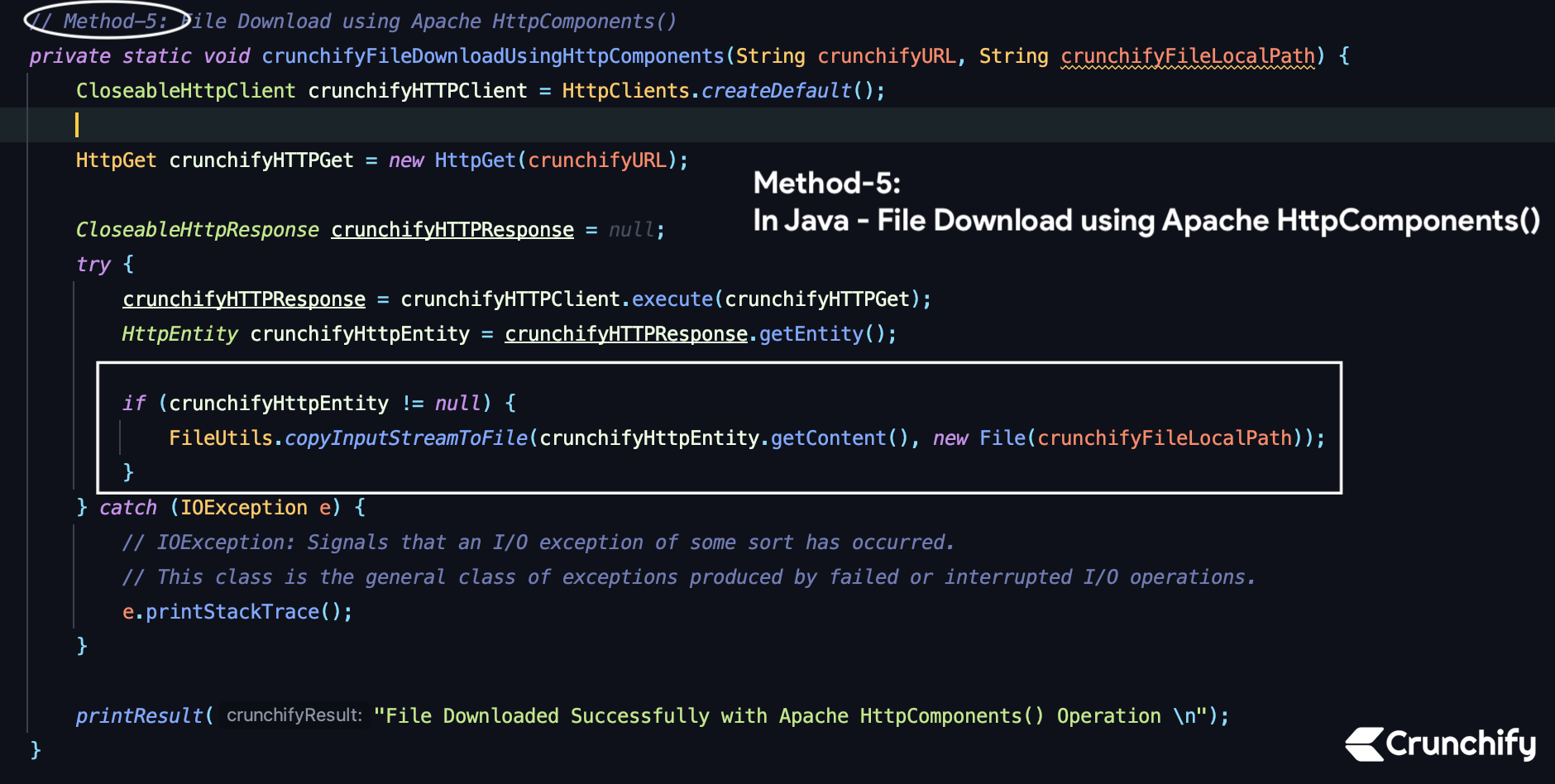
Let me know if you face any issue or exception running above Java Program.