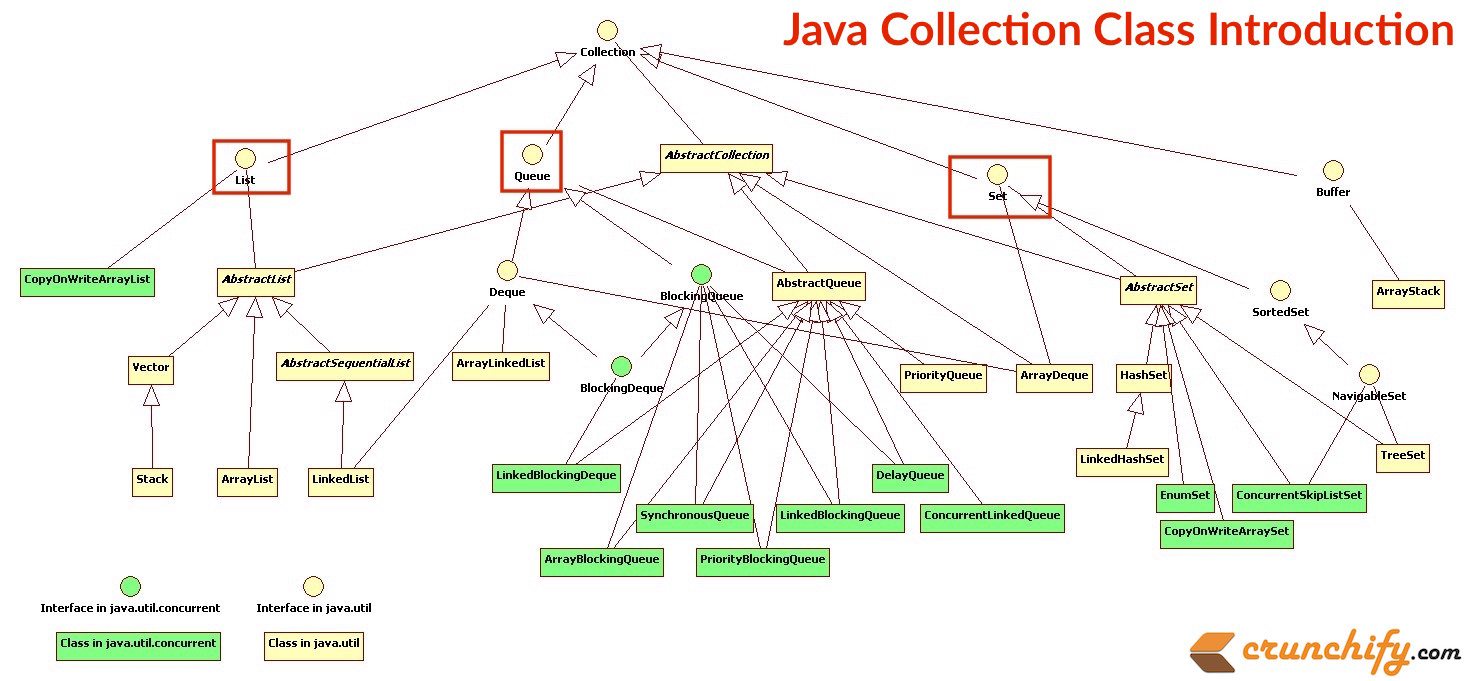
Here is one way to implement the union of two arrays using the Java Collection class.
In this example I’m using Java Collection Class TreeSet. This class implements the Set interface, backed by a TreeMap instance. This class guarantees that the sorted set will be in ascending element order, sorted according to the natural order of the elements (see Comparable), or by the comparator provided at set creation time, depending on which constructor is used.
If are you looking for Union of Two Arrays using Primitive Data Types
then visit this.
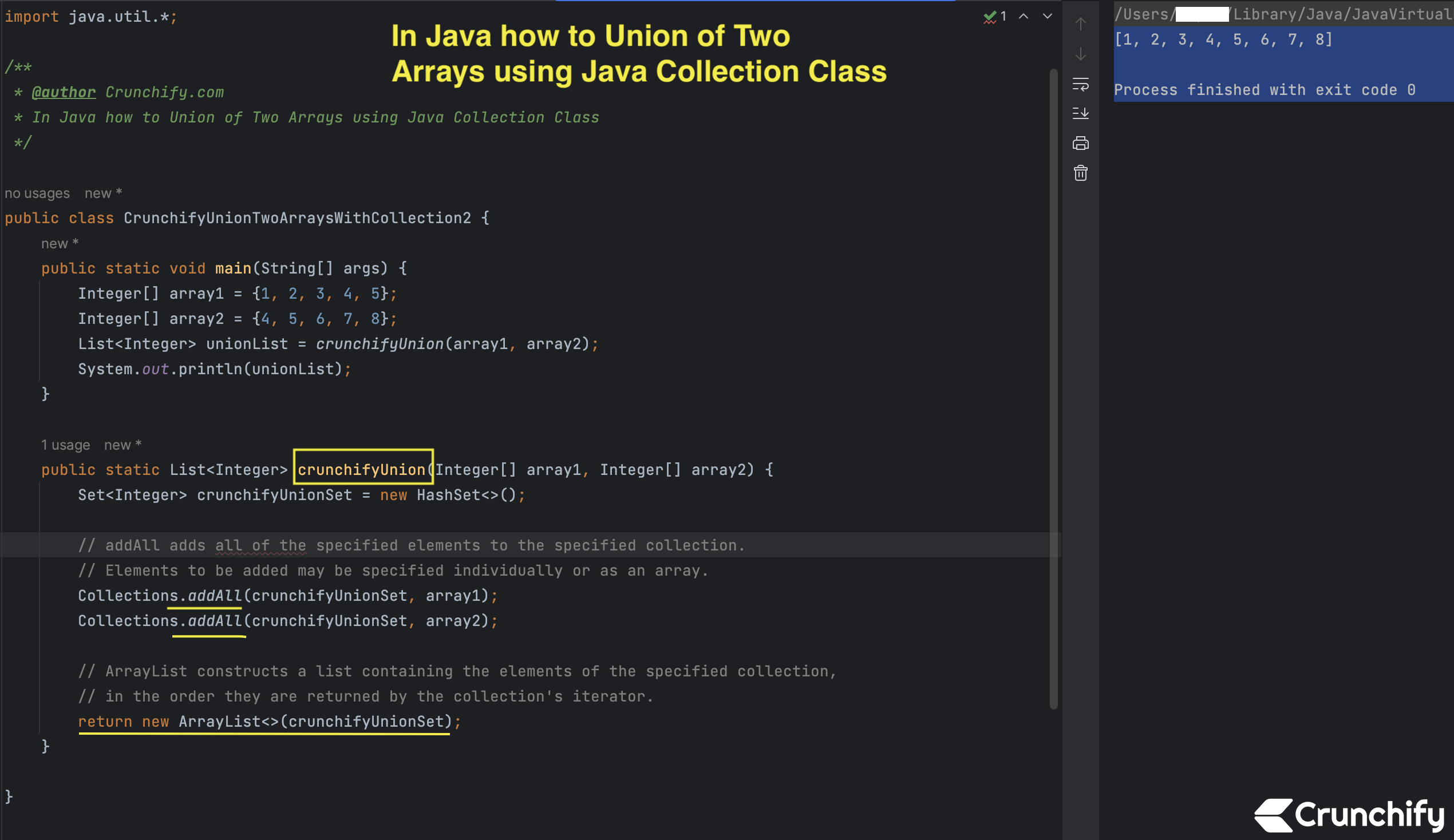
Java Code:
Method-1. CrunchifyUnionTwoArraysWithCollection2.java
package crunchify.com.tutorials; import java.util.*; /** * @author Crunchify.com * In Java how to Union of Two Arrays using Java Collection Class */ public class CrunchifyUnionTwoArraysWithCollection2 { public static void main(String[] args) { Integer[] array1 = {1, 2, 3, 4, 5}; Integer[] array2 = {4, 5, 6, 7, 8}; List<Integer> unionList = crunchifyUnion(array1, array2); System.out.println(unionList); } public static List<Integer> crunchifyUnion(Integer[] array1, Integer[] array2) { Set<Integer> crunchifyUnionSet = new HashSet<>(); // addAll adds all of the specified elements to the specified collection. // Elements to be added may be specified individually or as an array. Collections.addAll(crunchifyUnionSet, array1); Collections.addAll(crunchifyUnionSet, array2); // ArrayList constructs a list containing the elements of the specified collection, // in the order they are returned by the collection's iterator. return new ArrayList<>(crunchifyUnionSet); } }
IntelliJ IDEA console result:
[1, 2, 3, 4, 5, 6, 7, 8] Process finished with exit code 0
This program creates two arrays of integers and passes them to the union
method, which creates a HashSet
and adds all elements from both arrays to the set.
Since sets only allow unique elements, the resulting set will contain the union of the two arrays, with duplicates removed.
Finally, the set is converted to a list and returned. The main method prints out the resulting list, which should contain all elements from both arrays with duplicates removed.
Method-2. CrunchifyUnionTwoArraysWithCollection.java
package com.crunchify.tutorials; /** * @author Crunchify.com */ import java.util.TreeSet; public class CrunchifyUnionTwoArraysWithCollection { public static void main(String[] args) { Integer[] arrayOne = { 4, 11, 2, 1, 3, 3, 5, 7 }; Integer[] arrayTwo = { 5, 2, 3, 15, 1, 0, 9 }; Integer[] union = findUnion(arrayOne, arrayTwo); System.out.println("\nUnion of Two Arrays: "); for (Integer entry : union) { System.out.print(entry + " "); } } public static Integer[] findUnion(Integer[] arrayOne, Integer[] arrayTwo) { // TreeSet<Integer> hashedArray = new TreeSet<Integer>(); for (Integer entry : arrayOne) { hashedArray.add(entry); } for (Integer entry : arrayTwo) { hashedArray.add(entry); } return hashedArray.toArray(new Integer[0]); } }
This implementation provides guaranteed log(n) time
cost for the basic operations (add, remove and contains).
Note that the ordering maintained by a set
(whether or not an explicit comparator is provided) must be consistent with equals if it is to correctly implement the Set interface (See Comparable or Comparator for a precise definition of consistent with equals.)
This is so because the Set interface is defined in terms of the equals operation, but a TreeSet instance performs all key comparisons using its compareTo (or compare) method, so two keys that are deemed equal by this method are, from the standpoint of the set, equal. The behavior of a set is well-defined even if its ordering is inconsistent with equals; it just fails to obey the general contract of the Set interface.
IntelliJ IDEA console result:
Union of Two Arrays: 0 1 2 3 4 5 7 9 11 15