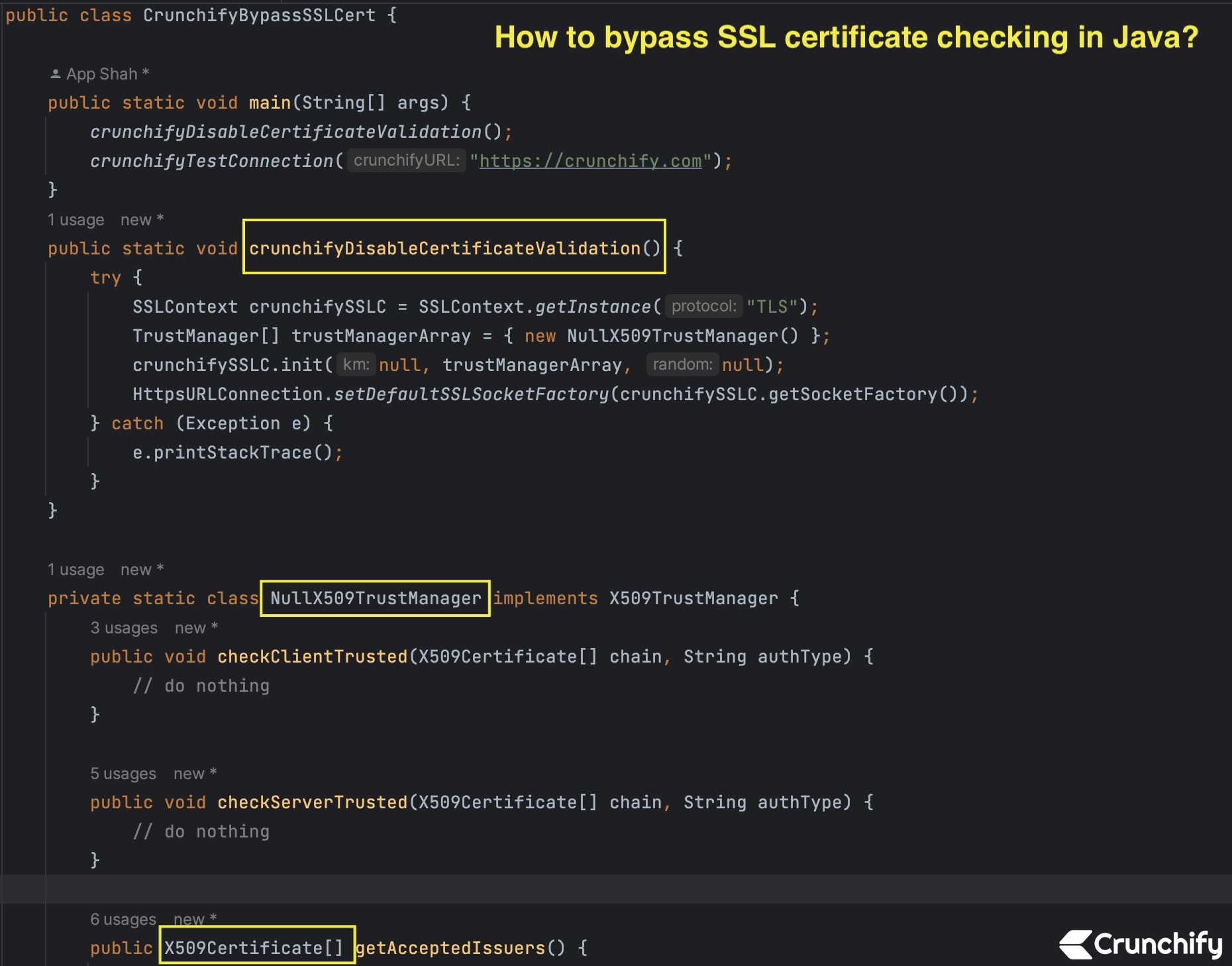
Secure Sockets Layer (SSL) and Transport Layer Security (TLS) are encryption protocols that are commonly used to secure communication over the internet. When a client (e.g. a web browser) communicates with a server using SSL/TLS, the server presents a certificate that the client can use to verify the server’s identity. If the certificate is not valid, the client will usually show a warning and refuse to communicate with the server.
However, there may be times when you need to bypass this certificate validation, for example during testing or when connecting to an internal server with a self-signed certificate. In this post, we will show you how to disable SSL certificate validation in Java.
Why bypassing SSL certificate validation is not recommended
Bypassing SSL certificate validation undermines the security of SSL/TLS encryption. It allows you to connect to a server that could be impersonating another server, which could allow an attacker to intercept and tamper with your communication. Therefore, it is not recommended to disable certificate validation in production systems.
How to bypass SSL certificate validation in Java
Here is an example code that demonstrates how to bypass SSL certificate validation in Java:
CrunchifyBypassSSLCert.java
package crunchify.com.java.tutorials; import javax.net.ssl.HttpsURLConnection; import javax.net.ssl.SSLContext; import javax.net.ssl.TrustManager; import javax.net.ssl.X509TrustManager; import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.URL; import java.security.cert.X509Certificate; /** * @author Crunchify.com * Version: 2.1 * How to bypass SSL certificate checking in Java? * */ public class CrunchifyBypassSSLCert { public static void main(String[] args) { crunchifyDisableCertificateValidation(); crunchifyTestConnection("https://crunchify.com"); } public static void crunchifyDisableCertificateValidation() { try { // SSLContext: Instances of this class represent a secure socket protocol implementation // which acts as a factory for secure socket factories or SSLEngines. // This class is initialized with an optional set of key and trust managers and source of secure random bytes. SSLContext crunchifySSLC = SSLContext.getInstance("TLS"); TrustManager[] trustManagerArray = { new NullX509TrustManager() }; crunchifySSLC.init(null, trustManagerArray, null); HttpsURLConnection.setDefaultSSLSocketFactory(crunchifySSLC.getSocketFactory()); } catch (Exception e) { e.printStackTrace(); } } private static class NullX509TrustManager implements X509TrustManager { public void checkClientTrusted(X509Certificate[] chain, String authType) { // do nothing } public void checkServerTrusted(X509Certificate[] chain, String authType) { // do nothing } public X509Certificate[] getAcceptedIssuers() { return new X509Certificate[0]; } } public static void crunchifyTestConnection(String crunchifyURL) { try { URL crunchifySiteURL = new URL(crunchifyURL); // HttpsURLConnection extends HttpURLConnection with support for https-specific features. HttpsURLConnection connection = (HttpsURLConnection) crunchifySiteURL.openConnection(); connection.connect(); // Abstract class for X.509 certificates. // This provides a standard way to access all the attributes of an X.509 certificate. X509Certificate[] certs = (X509Certificate[]) connection.getServerCertificates(); for (X509Certificate cert : certs) { System.out.println("Here is a Certificate: " + cert.toString()); } BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream())); String inputLine; System.out.println("Crunchify Response Starts: "); while ((inputLine = in.readLine()) != null) { System.out.println(inputLine); } in.close(); System.out.println("Crunchify Response Code: " + connection.getResponseCode()); } catch (Exception e) { e.printStackTrace(); } } }
The code creates a custom TrustManager
implementation called NullX509TrustManager
that simply returns an empty array of accepted issuers.
The disableCertificateValidation
method sets this custom trust manager as the default for the HttpsURLConnection
class.
IntelliJ IDEA Console Result:
Just run above program as a Java Application and you will see result as below. Here I’m printing Certificate for site https://crunchify.com
Here is a Certificate: [ [ Version: V3 Subject: CN=crunchify.com, O="Cloudflare, Inc.", L=San Francisco, ST=California, C=US Signature Algorithm: SHA256withECDSA, OID = 1.2.840.10045.4.3.2 Key: Sun EC public key, 256 bits public x coord: 38529685942248262624712688939942529436235652555283274298255011250522606614918 public y coord: 29334354097022760577193442773858953165938409689794173149697689580356278546401 parameters: secp256r1 [NIST P-256,X9.62 prime256v1] (1.2.840.10045.3.1.7) Validity: [From: Fri Jul 22 19:00:00 CDT 2022, To: Sun Jul 23 18:59:59 CDT 2023] Issuer: CN=Cloudflare Inc ECC CA-3, O="Cloudflare, Inc.", C=US SerialNumber: [ 03c5734c 15f2a24f a8554c49 a0935d45] Certificate Extensions: 10 [1]: ObjectId: 1.3.6.1.4.1.11129.2.4.2 Criticality=false Extension unknown: DER encoded OCTET string = 0000: 04 82 01 6E 04 82 01 6A 01 68 00 76 00 AD F7 BE ...n...j.h.v.... 0010: FA 7C FF 10 C8 8B 9D 3D 9C 1E 3E 18 6A B4 67 29 .......=..>.j.g) 0020: 5D CF B1 0C 24 CA 85 86 34 EB DC 82 8A 00 00 01 ]...$...4....... 0030: 82 28 D4 6A 70 00 00 04 03 00 47 30 45 02 20 19 .(.jp.....G0E. . 0040: 31 3C 0C 39 7A A9 8E 78 B6 0B B2 37 25 FE 62 23 1<.9z..x...7%.b# 0050: 53 4F 27 75 17 A7 B8 01 FB C4 E6 91 E1 CB 31 02 SO'u..........1. 0060: 21 00 B2 A2 7C 06 26 8E 72 83 02 9A 58 52 0E 31 !.....&.r...XR.1 0070: 99 6F 2C 2A 0C 34 0A B6 34 45 F1 A9 79 A2 4F B7 .o,*.4..4E..y.O. 0080: 8A DB 00 76 00 35 CF 19 1B BF B1 6C 57 BF 0F AD ...v.5.....lW... 0090: 4C 6D 42 CB BB B6 27 20 26 51 EA 3F E1 2A EF A8 LmB...' &Q.?.*.. 00A0: 03 C3 3B D6 4C 00 00 01 82 28 D4 6A 57 00 00 04 ..;.L....(.jW... 00B0: 03 00 47 30 45 02 20 5F D6 85 1B 65 51 68 C7 BE ..G0E. _...eQh.. 00C0: 5A 77 12 47 98 93 7D F6 18 BB 5E 74 12 62 42 A5 Zw.G......^t.bB. 00D0: 38 95 64 F4 F1 8F CA 02 21 00 DD BD 79 13 78 A7 8.d.....!...y.x. 00E0: E4 A7 A1 9C 40 C2 2E E0 3E DF 16 9F 70 70 E7 A1 ....@...>...pp.. 00F0: 78 01 16 3D D1 7B 1C E0 60 1E 00 76 00 B7 3E FB x..=....`..v..>. 0100: 24 DF 9C 4D BA 75 F2 39 C5 BA 58 F4 6C 5D FC 42 $..M.u.9..X.l].B 0110: CF 7A 9F 35 C4 9E 1D 09 81 25 ED B4 99 00 00 01 .z.5.....%...... 0120: 82 28 D4 6A 5B 00 00 04 03 00 47 30 45 02 21 00 .(.j[.....G0E.!. 0130: AA 7C E3 08 41 E7 3B 84 1B B8 FE 06 4B 7C 83 27 ....A.;.....K..' 0140: CF 4A 81 3F 07 D4 32 FC 69 80 22 D5 DC 06 9B D5 .J.?..2.i."..... 0150: 02 20 0B 50 38 88 53 20 A0 A6 CD 6D 88 37 7C 6D . .P8.S ...m.7.m 0160: E2 E2 8E D9 B1 B7 16 1E A6 EA 38 C2 D3 D7 9B 48 ..........8....H 0170: 34 3C 4< [2]: ObjectId: 1.3.6.1.5.5.7.1.1 Criticality=false AuthorityInfoAccess [ [ accessMethod: ocsp accessLocation: URIName: http://ocsp.digicert.com , accessMethod: caIssuers accessLocation: URIName: http://cacerts.digicert.com/CloudflareIncECCCA-3.crt ] ] [3]: ObjectId: 2.5.29.35 Criticality=false AuthorityKeyIdentifier [ KeyIdentifier [ 0000: A5 CE 37 EA EB B0 75 0E 94 67 88 B4 45 FA D9 24 ..7...u..g..E..$ 0010: 10 87 96 1F .... ] ] [4]: ObjectId: 2.5.29.19 Criticality=true BasicConstraints:[ CA:false PathLen: undefined ] [5]: ObjectId: 2.5.29.31 Criticality=false CRLDistributionPoints [ [DistributionPoint: [URIName: http://crl3.digicert.com/CloudflareIncECCCA-3.crl] , DistributionPoint: [URIName: http://crl4.digicert.com/CloudflareIncECCCA-3.crl] ]] [6]: ObjectId: 2.5.29.32 Criticality=false CertificatePolicies [ [CertificatePolicyId: [2.23.140.1.2.2] [PolicyQualifierInfo: [ qualifierID: 1.3.6.1.5.5.7.2.1 qualifier: 0000: 16 1B 68 74 74 70 3A 2F 2F 77 77 77 2E 64 69 67 ..http://www.dig 0010: 69 63 65 72 74 2E 63 6F 6D 2F 43 50 53 icert.com/CPS ]] ] ] [7]: ObjectId: 2.5.29.37 Criticality=false ExtendedKeyUsages [ serverAuth clientAuth ] [8]: ObjectId: 2.5.29.15 Criticality=true KeyUsage [ DigitalSignature ] [9]: ObjectId: 2.5.29.17 Criticality=false SubjectAlternativeName [ DNSName: *.crunchify.com DNSName: crunchify.com ] [10]: ObjectId: 2.5.29.14 Criticality=false SubjectKeyIdentifier [ KeyIdentifier [ 0000: CF 6E 31 8C 88 C5 ED 09 6D F6 97 57 CE D4 F0 EC .n1.....m..W.... 0010: B5 FD 99 1A .... ] ] ] Algorithm: [SHA256withECDSA] Signature: 0000: 30 45 02 20 48 32 9D F1 A0 BA 7A 89 0A 69 0E 5A 0E. H2....z..i.Z 0010: D6 50 58 9F B4 89 A8 FF BE A3 BF 3F 80 6E DD F2 .PX........?.n.. 0020: C9 1E BB 04 02 21 00 D3 A8 8F E6 0F 23 E3 59 D7 .....!......#.Y. 0030: 60 3C 74 A7 BF F1 4D 8E 99 0D B9 85 75 15 14 DE `<t...M.....u... 0040: 6D B4 B4 01 BB E2 6F m.....o ] Here is a Certificate: [ [ Version: V3 Subject: CN=Cloudflare Inc ECC CA-3, O="Cloudflare, Inc.", C=US Signature Algorithm: SHA256withRSA, OID = 1.2.840.113549.1.1.11 Key: Sun EC public key, 256 bits public x coord: 83984075730615231530440956498748499276900957075036316089284983112230089232319 public y coord: 84720202049003273739269829519636180374924996951868121119946393481023066512343 parameters: secp256r1 [NIST P-256,X9.62 prime256v1] (1.2.840.10045.3.1.7) Validity: [From: Mon Jan 27 06:48:08 CST 2020, To: Tue Dec 31 17:59:59 CST 2024] Issuer: CN=Baltimore CyberTrust Root, OU=CyberTrust, O=Baltimore, C=IE SerialNumber: [ 0a378764 5e5fb48c 224efd1b ed140c3c] Certificate Extensions: 8 [1]: ObjectId: 1.3.6.1.5.5.7.1.1 Criticality=false AuthorityInfoAccess [ [ accessMethod: ocsp accessLocation: URIName: http://ocsp.digicert.com ] ] [2]: ObjectId: 2.5.29.35 Criticality=false AuthorityKeyIdentifier [ KeyIdentifier [ 0000: E5 9D 59 30 82 47 58 CC AC FA 08 54 36 86 7B 3A ..Y0.GX....T6..: 0010: B5 04 4D F0 ..M. ] ] [3]: ObjectId: 2.5.29.19 Criticality=true BasicConstraints:[ CA:true PathLen:0 ] [4]: ObjectId: 2.5.29.31 Criticality=false CRLDistributionPoints [ [DistributionPoint: [URIName: http://crl3.digicert.com/Omniroot2025.crl] ]] [5]: ObjectId: 2.5.29.32 Criticality=false CertificatePolicies [ [CertificatePolicyId: [2.16.840.1.114412.1.1] [PolicyQualifierInfo: [ qualifierID: 1.3.6.1.5.5.7.2.1 qualifier: 0000: 16 1C 68 74 74 70 73 3A 2F 2F 77 77 77 2E 64 69 ..https://www.di 0010: 67 69 63 65 72 74 2E 63 6F 6D 2F 43 50 53 gicert.com/CPS ]] ] [CertificatePolicyId: [2.16.840.1.114412.1.2] [] ] [CertificatePolicyId: [2.23.140.1.2.1] [] ] [CertificatePolicyId: [2.23.140.1.2.2] [] ] [CertificatePolicyId: [2.23.140.1.2.3] [] ] ] [6]: ObjectId: 2.5.29.37 Criticality=false ExtendedKeyUsages [ serverAuth clientAuth ] [7]: ObjectId: 2.5.29.15 Criticality=true KeyUsage [ DigitalSignature Key_CertSign Crl_Sign ] [8]: ObjectId: 2.5.29.14 Criticality=false SubjectKeyIdentifier [ KeyIdentifier [ 0000: A5 CE 37 EA EB B0 75 0E 94 67 88 B4 45 FA D9 24 ..7...u..g..E..$ 0010: 10 87 96 1F .... ] ] ] Algorithm: [SHA256withRSA] Signature: 0000: 05 24 1D DD 1B B0 2A EB 98 D6 85 E3 39 4D 5E 6B .$....*.....9M^k 0010: 57 9D 82 57 FC EB E8 31 A2 57 90 65 05 BE 16 44 W..W...1.W.e...D 0020: 38 5A 77 02 B9 CF 10 42 C6 E1 92 A4 E3 45 27 F8 8Zw....B.....E'. 0030: 00 47 2C 68 A8 56 99 53 54 8F AD 9E 40 C1 D0 0F .G,h.V.ST...@... 0040: B6 D7 0D 0B 38 48 6C 50 2C 49 90 06 5B 64 1D 8B ....8HlP,I..[d.. 0050: CC 48 30 2E DE 08 E2 9B 49 22 C0 92 0C 11 5E 96 .H0.....I"....^. 0060: 92 94 D5 FC 20 DC 56 6C E5 92 93 BF 7A 1C C0 37 .... .Vl....z..7 0070: E3 85 49 15 FA 2B E1 74 39 18 0F B7 DA F3 A2 57 ..I..+.t9......W 0080: 58 60 4F CC 8E 94 00 FC 46 7B 34 31 3E 4D 47 82 X`O.....F.41>MG. 0090: 81 3A CB F4 89 5D 0E EF 4D 0D 6E 9C 1B 82 24 DD .:...]..M.n...$. 00A0: 32 25 5D 11 78 51 10 3D A0 35 23 04 2F 65 6F 9C 2%].xQ.=.5#./eo. 00B0: C1 D1 43 D7 D0 1E F3 31 67 59 27 DD 6B D2 75 09 ..C....1gY'.k.u. 00C0: 93 11 24 24 14 CF 29 BE E6 23 C3 B8 8F 72 3F E9 ..$$..)..#...r?. 00D0: 07 C8 24 44 53 7A B3 B9 61 65 A1 4C 0E C6 48 00 ..$DSz..ae.L..H. 00E0: C9 75 63 05 87 70 45 52 83 D3 95 9D 45 EA F0 E8 .uc..pER....E... 00F0: 31 1D 7E 09 1F 0A FE 3E DD AA 3C 5E 74 D2 AC B1 1......>..<^t... ]
Bypassing SSL certificate validation is not recommended for production systems because it undermines the security of SSL/TLS encryption.
However, it may be necessary for testing purposes.
The code shown in this post demonstrates how to disable certificate validation in Java, but keep in mind that this should only be used for testing purposes and not in production systems.
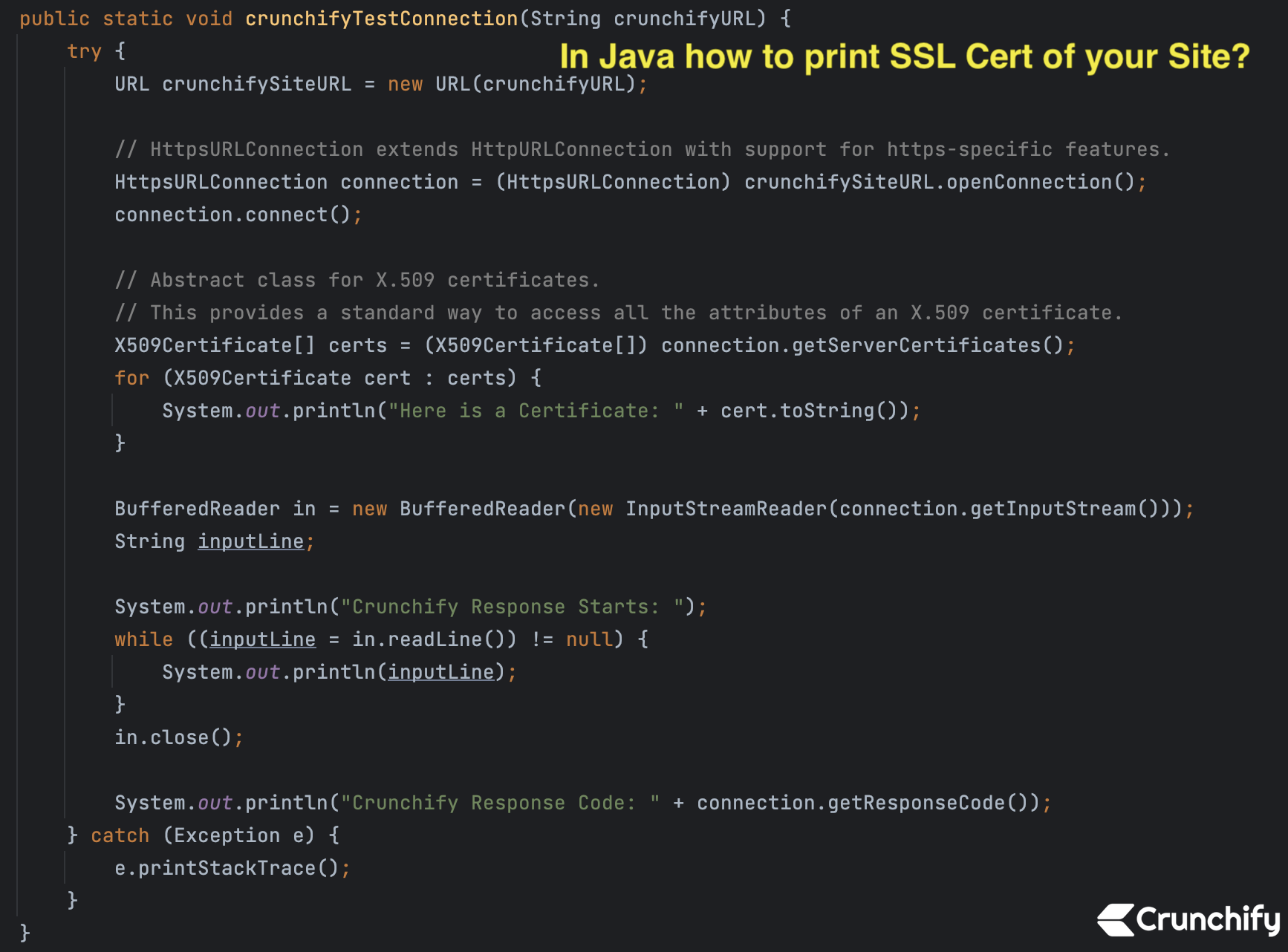
Let me know if you face any issue running this code.