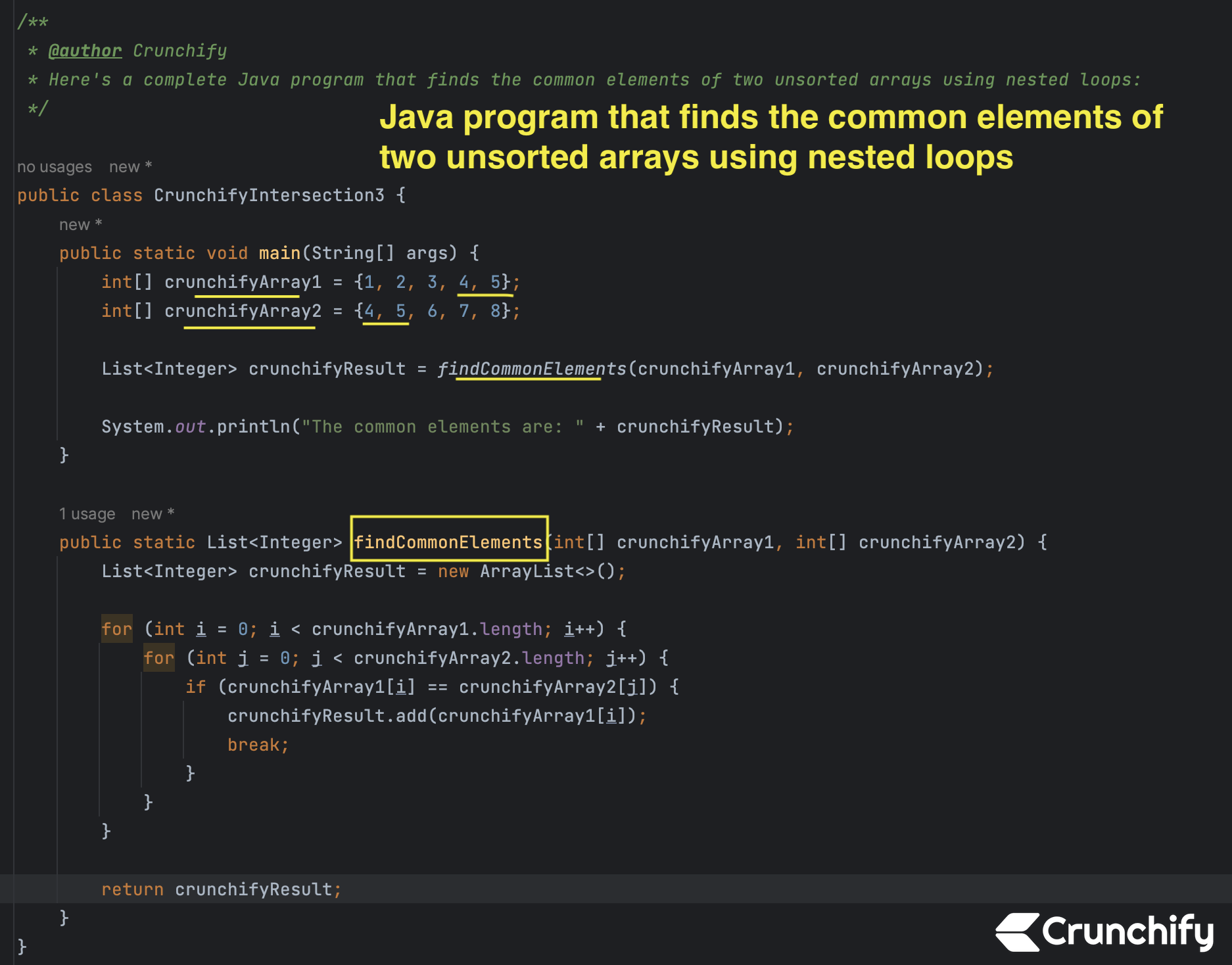
How to Find Common Elements of Two UnSorted Array? Below is a simple Java Code which shows it.
Approach-1.
- The program starts by defining two arrays
array1
andarray2
. - The
findCommonElements
method takes two arrays as arguments and returns aList
of integers that are the common elements between the two arrays. - The method uses two nested loops, the outer loop iterates through each element of
array1
, and the inner loop iterates through each element ofarray2
. - The inner loop checks if the current element of
array1
is equal to the current element ofarray2
. - If a match is found, the common element is added to the result
List
. - The method returns the result
List
which contains the common elements. - The main method calls the
findCommonElements
method and prints the result.
CrunchifyIntersection3.java
package crunchify.com.tutorials; import java.util.ArrayList; import java.util.List; /** * @author Crunchify * Here's a complete Java program that finds the common elements of two unsorted arrays using nested loops: */ public class CrunchifyIntersection3 { public static void main(String[] args) { int[] crunchifyArray1 = {1, 2, 3, 4, 5}; int[] crunchifyArray2 = {4, 5, 6, 7, 8}; List<Integer> crunchifyResult = findCommonElements(crunchifyArray1, crunchifyArray2); System.out.println("The common elements are: " + crunchifyResult); } public static List<Integer> findCommonElements(int[] crunchifyArray1, int[] crunchifyArray2) { List<Integer> crunchifyResult = new ArrayList<>(); for (int i = 0; i < crunchifyArray1.length; i++) { for (int j = 0; j < crunchifyArray2.length; j++) { if (crunchifyArray1[i] == crunchifyArray2[j]) { crunchifyResult.add(crunchifyArray1[i]); break; } } } return crunchifyResult; } }
Eclipse Console Result:
The common elements are: [4, 5] Process finished with exit code 0
Approach-2.
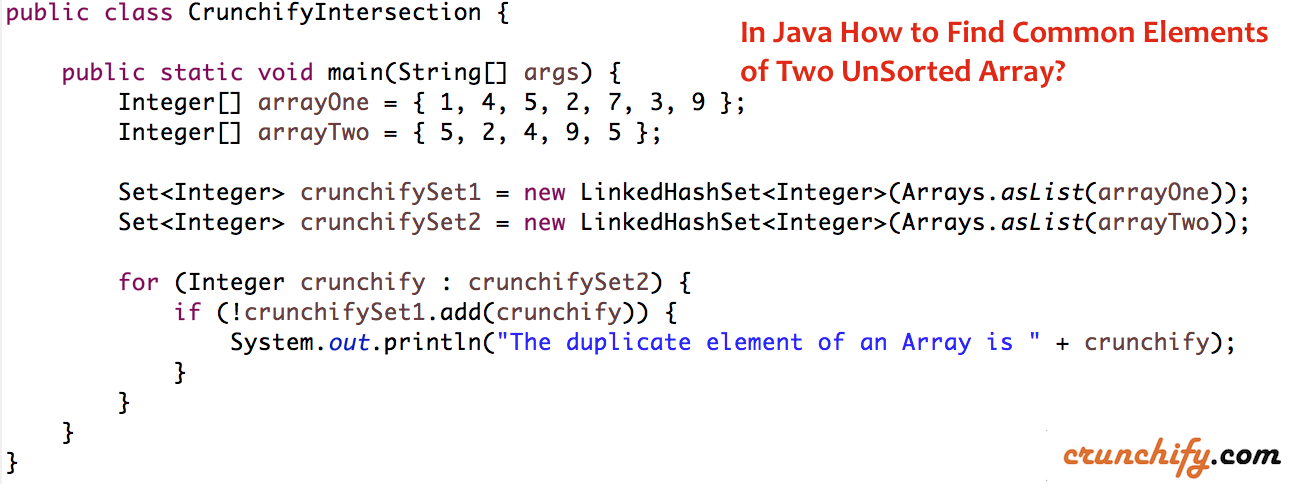
Steps:
- Find 1st small array.
- Iterate through small array and find elements in largest array.
- In case of match, add it to separate new array.
- Display final array.
package com.crunchify; import java.util.HashSet; /** * @author Crunchify.com */ public class CrunchifyIntersection { public static void main(String[] args) { Integer[ ] arrayOne = { 1, 4, 5, 2, 7, 3, 9 }; Integer[ ] arrayTwo = { 5, 2, 4, 9, 5 }; Integer[ ] common = CrunchifyIntersection.findCommon( arrayOne, arrayTwo ); System.out.print( "Common Elements Between Two Arrays: " ); for( Integer entry : common ) { System.out.print( entry + " " ); } } public static Integer[ ] findCommon( Integer[ ] arrayOne, Integer[ ] arrayTwo ) { Integer[ ] arrayToHash; Integer[ ] arrayToSearch; if( arrayOne.length < arrayTwo.length ) { arrayToHash = arrayOne; arrayToSearch = arrayTwo; } else { arrayToHash = arrayTwo; arrayToSearch = arrayOne; } HashSet<Integer> intersection = new HashSet<Integer>( ); HashSet<Integer> hashedArray = new HashSet<Integer>( ); for( Integer entry : arrayToHash ) { hashedArray.add( entry ); } for( Integer entry : arrayToSearch ) { if( hashedArray.contains( entry ) ) { intersection.add( entry ); } } return intersection.toArray( new Integer[ 0 ] ); } }
Output:
Common Elements Between Two Arrays: 2 4 5 9
Approach-3.
Below approach is suggested by our reader Maksudur rahman
as you see it in below comment section. Please find here complete example.
package crunchify.com.tutorials; import java.util.Arrays; import java.util.LinkedHashSet; import java.util.Set; /** * @author Crunchify.com * */ public class CrunchifyIntersection { public static void main(String[] args) { Integer[] arrayOne = { 1, 4, 5, 2, 7, 3, 9 }; Integer[] arrayTwo = { 5, 2, 4, 9, 5 }; Set<Integer> crunchifySet1 = new LinkedHashSet<Integer>(Arrays.asList(arrayOne)); Set<Integer> crunchifySet2 = new LinkedHashSet<Integer>(Arrays.asList(arrayTwo)); for (Integer crunchify : crunchifySet2) { if (!crunchifySet1.add(crunchify)) { System.out.println("The duplicate element of an Array is " + crunchify); } } } }
Output:
The duplicate element of an Array is 5 The duplicate element of an Array is 2 The duplicate element of an Array is 4 The duplicate element of an Array is 9
Let me know if you face any issue running this Java program.