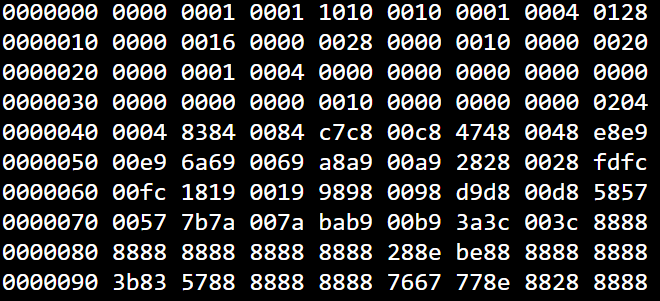
Efficient programming often involves optimizing code for speed and memory usage. One powerful but underutilized technique is leveraging binary notation to optimize operations at a low level. Binary operations can lead to significant performance improvements, especially in systems programming, embedded development, and high-performance applications.
Why Use Binary Notation?
Binary notation allows for more efficient computations, reducing reliance on arithmetic operations that consume extra processing power. Many modern processors handle bitwise operations faster than traditional arithmetic calculations.
Common Binary Operations for Optimization
1. Bitwise AND (&
) and OR (|
) for Conditional Checks
Instead of using multiple conditional statements, you can optimize checks with bitwise operations.
Example:
// Check if a number is even if (num & 1 == 0) { printf("Even number"); }
This avoids modulus (%
) operations, which are generally more expensive than bitwise operations.
2. Bitwise Shift (<<
, >>
) for Fast Multiplication and Division
Bitwise shifts provide a faster way to multiply or divide by powers of two.
Example:
int multiplyBy8 = num << 3; // Equivalent to num * 8 int divideBy4 = num >> 2; // Equivalent to num / 4
This is much faster than using *
or /
, as shifting only requires a single CPU cycle.
3. Using XOR (^
) for Swapping Variables Without a Temp Variable
XOR can be used to swap two variables without needing extra memory.
Example:
x = x ^ y; y = x ^ y; x = x ^ y;
This can be useful in constrained environments like embedded systems.
4. Checking if a Number is a Power of Two
A quick way to determine if a number is a power of two is using the following condition:
Example:
if ((num & (num - 1)) == 0 && num != 0) { printf("Power of two"); }
This takes advantage of the binary representation of powers of two, where only a single bit is set.
Conclusion
Utilizing binary notation and bitwise operations can significantly optimize code performance. These techniques are especially useful in low-level programming, graphics rendering, cryptography, and embedded systems. By understanding and applying binary optimizations, developers can write faster and more efficient code.
Full Java program:
public class CrunchifyBinaryOptimization { public static void main(String[] args) { int crunchifyNum = 8; // Check if a number is even using bitwise AND if ((crunchifyNum & 1) == 0) { System.out.println(crunchifyNum + " is even"); } else { System.out.println(crunchifyNum + " is odd"); } // Multiply and divide using bitwise shift int crunchifyMultiplyBy8 = crunchifyNum << 3; // num * 8 int crunchifyDivideBy4 = crunchifyNum >> 2; // num / 4 System.out.println("Multiply by 8: " + crunchifyMultiplyBy8); System.out.println("Divide by 4: " + crunchifyDivideBy4); // Swap two numbers using XOR int crunchifyA = 5, crunchifyB = 3; System.out.println("Before swap: a = " + crunchifyA + ", b = " + crunchifyB); crunchifyA = crunchifyA ^ crunchifyB; crunchifyB = crunchifyA ^ crunchifyB; crunchifyA = crunchifyA ^ crunchifyB; System.out.println("After swap: a = " + crunchifyA + ", b = " + crunchifyB); // Check if a number is a power of two if ((crunchifyNum & (crunchifyNum - 1)) == 0 && crunchifyNum != 0) { System.out.println(crunchifyNum + " is a power of two"); } else { System.out.println(crunchifyNum + " is not a power of two"); } } }
Console Result:
8 is even Multiply by 8: 64 Divide by 4: 2 Before swap: a = 5, b = 3 After swap: a = 3, b = 5 8 is a power of two