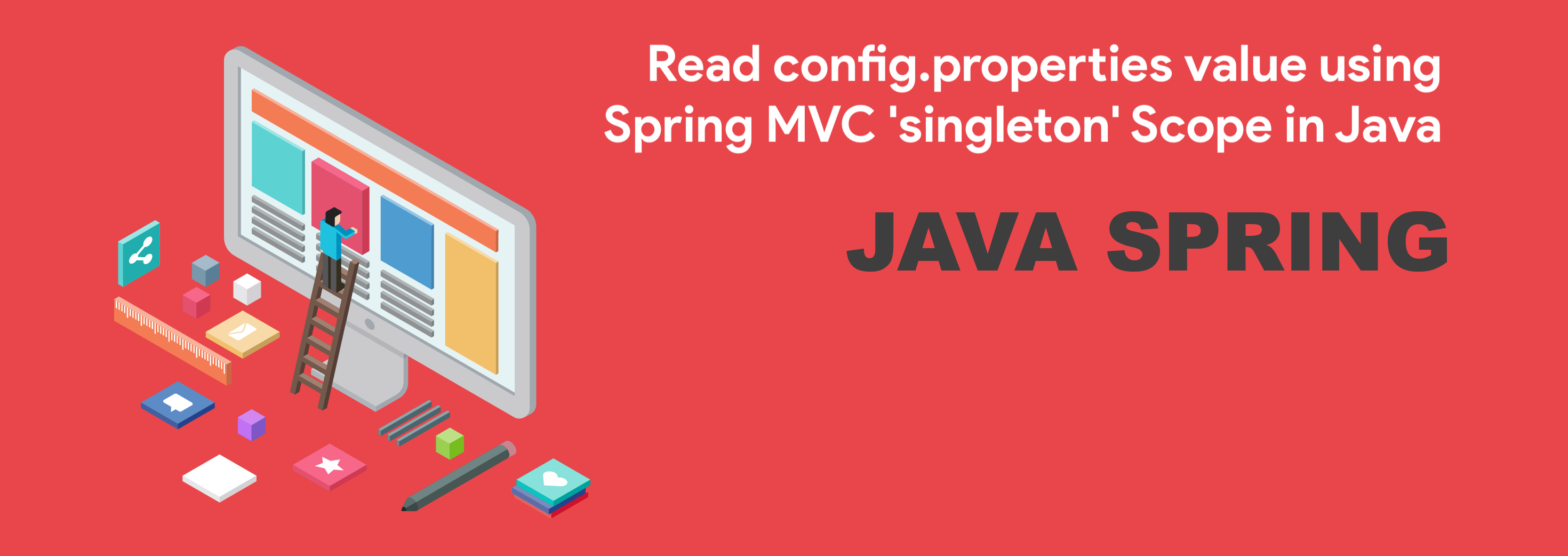
Sometime back I have written a tutorial on how to read config file in tradition way. But what if you want to load it as a Spring MVC framework?
It is most common that you may need to access some of your config.properties
values all the time in your Java class. There is a simple way to get it using Spring MVC’s singleton scope.
Kindly take a look at below complete workspace picture.
We need to create 4 files:
- CrunchifySpringExample.java under package
com.crunchify.tutorial
- config.properties file under
/refer/config/
folder - spring-bean.xml file under
/resources
folder - pom.xml file (convert project to Maven project)
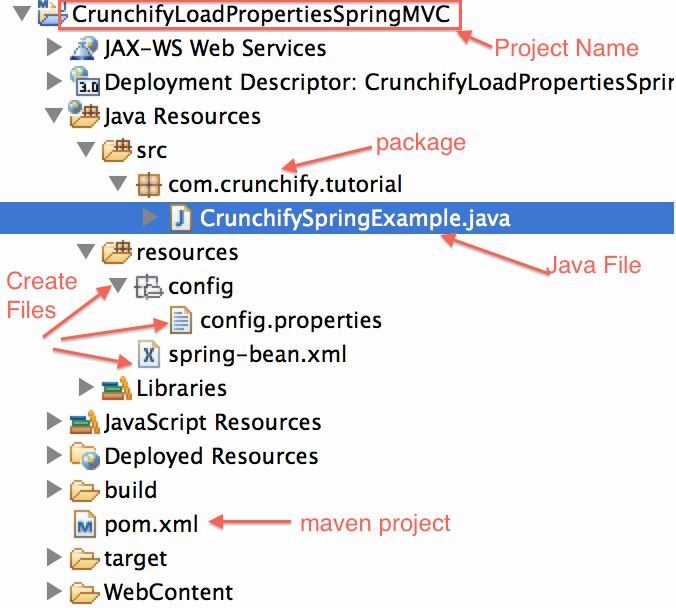
Let’s get started:
Step-1
Create Dynamic Web Project called CrunchifyLoadPropertiesSpringMVC
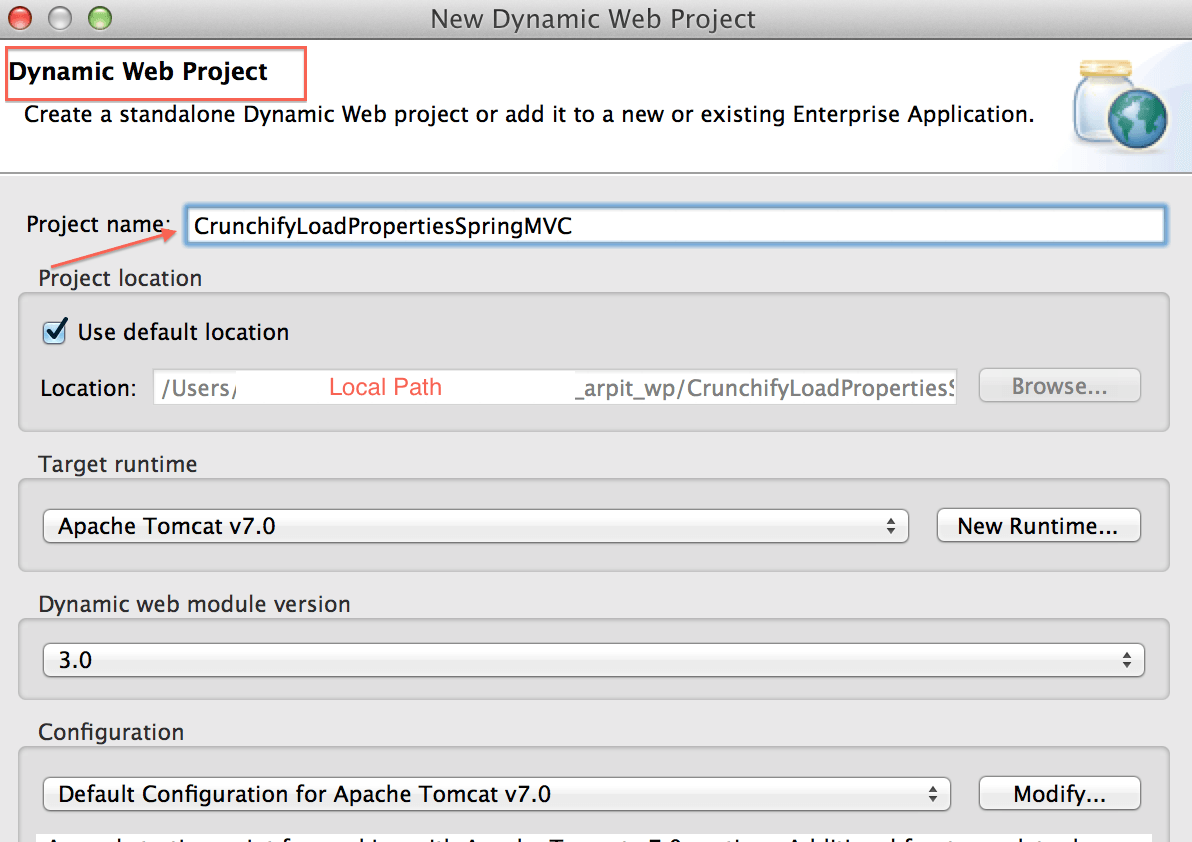
Step-2
Convert project to Maven Project.
This will create pom.xml
file and we will add Spring dependancies.
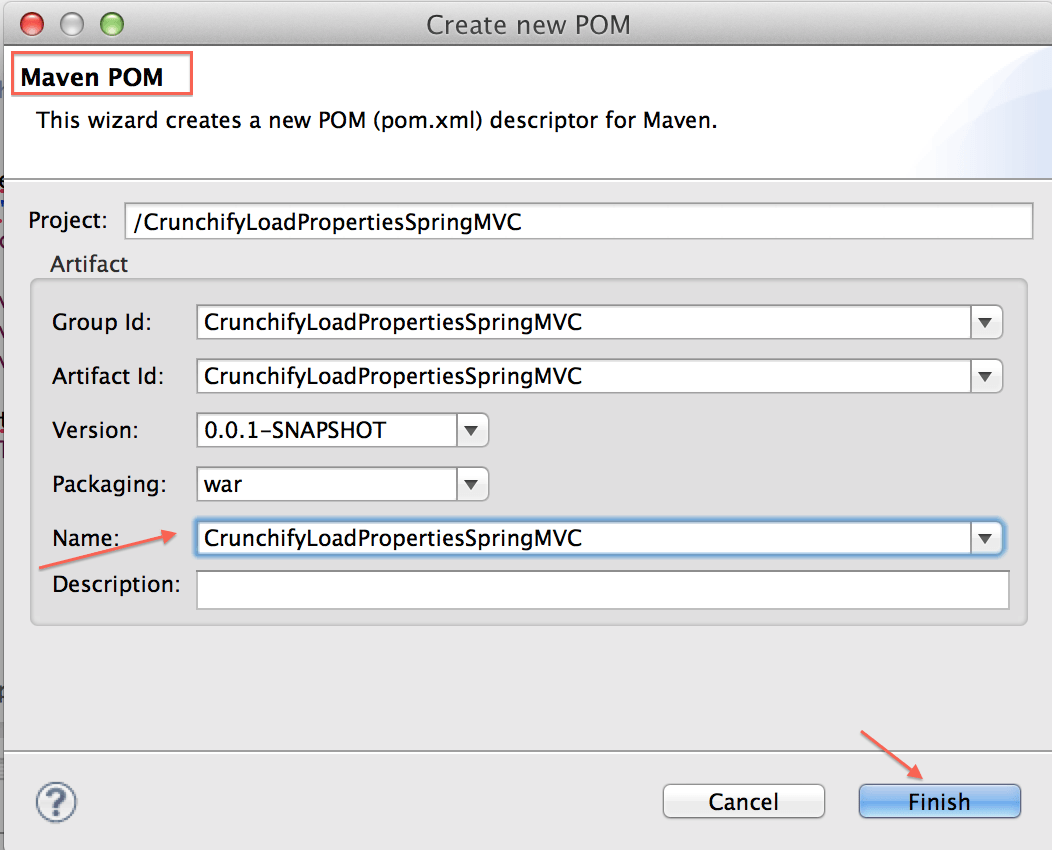
Step-3
Create file CrunchifySpringExample.java
package com.crunchify.tutorial; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Scope; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.stereotype.Component; /** * * @author Crunchify.com */ @Component("crunchifySpringExample") @Scope("singleton") public class CrunchifySpringExample { private static boolean springExample; private static String springTutorial; private static String url; @Autowired public CrunchifySpringExample(@Value("${CRUNCHIFY_URL}") String url, @Value("${SPRING_TUTORIAL}") String springTutorial, @Value("${IS_THIS_SPRING_EXAMPLE}") boolean springExample) { CrunchifySpringExample.springExample = springExample; CrunchifySpringExample.springTutorial = springTutorial; CrunchifySpringExample.url = url; } @SuppressWarnings({ "resource", "unused" }) public static void main(String[] args) { try { ClassPathXmlApplicationContext ctx = new ClassPathXmlApplicationContext(new String[] { "spring-bean.xml" }); } catch (Throwable e) { System.out.println(e); } System.out.println("\nLoading Properties from Config File during application startup: \n\nSPRING_TUTORIAL: " + springTutorial); System.out.println("IS_THIS_SPRING_EXAMPLE: " + springExample); System.out.println("CRUNCHIFY_URL: " + url); } }
Step-4
Here is my pom.xml
file.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>CrunchifyLoadPropertiesSpringMVC</groupId> <artifactId>CrunchifyLoadPropertiesSpringMVC</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>CrunchifyLoadPropertiesSpringMVC</name> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> <plugin> <artifactId>maven-war-plugin</artifactId> <version>2.3</version> <configuration> <warSourceDirectory>WebContent</warSourceDirectory> <failOnMissingWebXml>false</failOnMissingWebXml> </configuration> </plugin> </plugins> </build> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <spring.version>4.0.2.RELEASE</spring.version> </properties> <dependencies> <!-- Spring base --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring.version}</version> <exclusions> <exclusion> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> </exclusion> </exclusions> </dependency> <!-- Spring MVC --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> <version>1.1.1</version> </dependency> </dependencies> </project>
Step-5
If you don’t see “resources
” folder. create one.
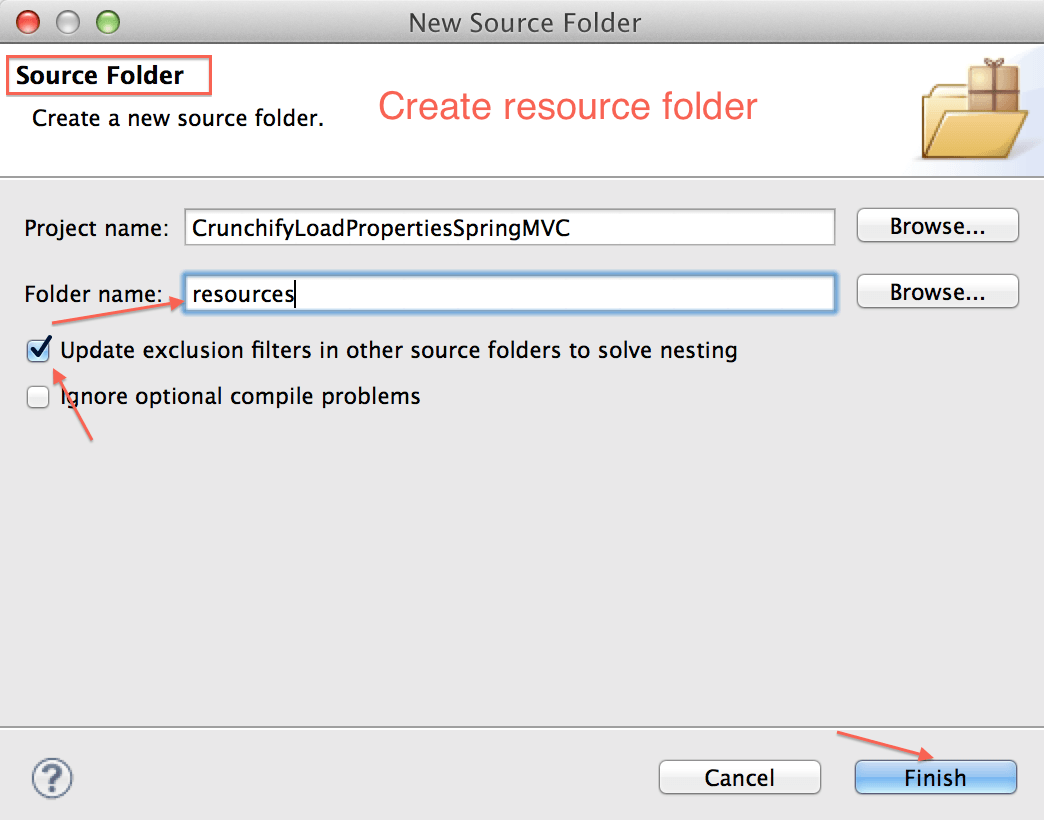
Step-6
Create config.properties
file with below contents.
# Crunchify Properties IS_THIS_SPRING_EXAMPLE=true SPRING_TUTORIAL=CRUNCHIFY_TUTORIAL CRUNCHIFY_URL=https://crunchify.com
Step-7
Content of spring-bean.xml
file
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:util="http://www.springframework.org/schema/util" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <util:properties id="nodeProperty" location="classpath:config/config.properties" /> <context:property-placeholder properties-ref="nodeProperty" /> <context:component-scan base-package="com.crunchify.tutorial" /> </beans>
Step-8
Now run CrunchifySpringExample.java
and you should see result like this.
Step-9
Output
Jul 05, 2014 3:38:52 PM org.springframework.context.support.AbstractApplicationContext prepareRefresh INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@7e3bfb66: startup date [Sat Jul 05 15:38:52 CDT 2014]; root of context hierarchy Jul 05, 2014 3:38:52 PM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions INFO: Loading XML bean definitions from class path resource [spring-bean.xml] Jul 05, 2014 3:38:52 PM org.springframework.core.io.support.PropertiesLoaderSupport loadProperties INFO: Loading properties file from class path resource [config/config.properties] Loading Properties from Config File during application startup: SPRING_TUTORIAL: CRUNCHIFY_TUTORIAL IS_THIS_SPRING_EXAMPLE: true CRUNCHIFY_URL: https://crunchify.com
Enjoy and Happy Coding..