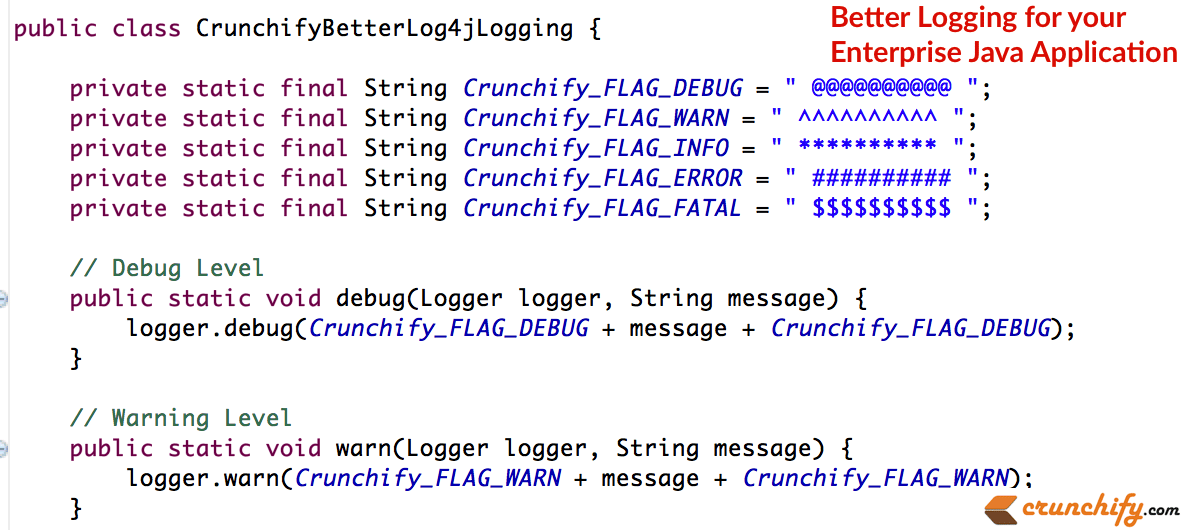
If you are developing Java Application where you need to use the logger functionality, there are number of ways you could enhance the Logger Utility.
To make sure that logging can be left in a production program, the Java logger API is designed to make logging as inexpensive as possible. To let code produce fine-grained logging when needed but not slow the application in normal production use, the API provides mechanisms to dynamically change what log messages are produced, so that the impact of the logging code is minimized during normal operation.
Now let’s start writing simple Logger Utility which will enhance your overall production logging quality.
Update Log4j to latest version
CVE-2021-44228: Apache Log4j2 <=2.14.1 JNDI features used in configuration, log messages, and parameters do not protect against attacker controlled LDAP and other JNDI related endpoints.
From log4j 2.16.0, this behavior has been disabled by default.
Step-1:
Create file CrunchifyBetterLog4jLogging.java
package com.crunchify.tutorial; import org.apache.log4j.Logger; /** * @author Crunchify.com * */ public class CrunchifyBetterLog4jLogging { private static final String Crunchify_FLAG_DEBUG = " ---------- "; private static final String Crunchify_FLAG_WARN = " ^^^^^^^^^^ "; private static final String Crunchify_FLAG_INFO = " ********** "; private static final String Crunchify_FLAG_ERROR = " ########## "; private static final String Crunchify_FLAG_FATAL = " $$$$$ "; // Debug Level public static void debug(Logger logger, String message) { logger.debug(Crunchify_FLAG_DEBUG + message + Crunchify_FLAG_DEBUG); } // Warning Level public static void warn(Logger logger, String message) { logger.warn(Crunchify_FLAG_WARN + message + Crunchify_FLAG_WARN); } // Info Level public static void info(Logger logger, String message) { logger.info(Crunchify_FLAG_INFO + message + Crunchify_FLAG_INFO); } // Error Level public static void error(Logger Logger, String message) { Logger.error(Crunchify_FLAG_ERROR + message + Crunchify_FLAG_ERROR); } // Fatal Level public static void fatal(Logger Logger, String message) { Logger.fatal(Crunchify_FLAG_FATAL + message + Crunchify_FLAG_FATAL); } }
Basically what we are doing is, when in your Java program you say log.info
or log.error
or log.fatal
, code will automatically add special characters as you see above.
Step-2
Testcase (Sample Java Program) – CrunchifyBetterLog4jLoggingTest.java
package com.crunchify.tutorial; import org.apache.log4j.Logger; public class CrunchifyBetterLog4jLoggingTest { private static Logger logger = Logger.getLogger(CrunchifyBetterLog4jLoggingTest.class); public static void main(String[] args) { int a = 20; int b = 30; int c = 3; int d = 4; CrunchifyBetterLog4jLoggingTest.SwapVairablesMethod1(a, b); CrunchifyBetterLog4jLoggingTest.SwapVairablesMethod2(c, d); } public static void SwapVairablesMethod1(int a, int b) { CrunchifyBetterLog4jLogging.debug(logger, "value of a and b before swapping, a: " + a + " b: " + b); // swapping value of two numbers without using temp variable a = a + b; // now a is 50 and b is 20 b = a - b; // now a is 50 but b is 20 (original value of a) a = a - b; // now a is 30 and b is 20, numbers are swapped CrunchifyBetterLog4jLogging.warn(logger, "Result Method1 => a: " + a + " b: " + b); } public static void SwapVairablesMethod2(int c, int d) { CrunchifyBetterLog4jLogging.info(logger, "value of c and d before swapping, c: " + c + " d: " + d); // swapping value of two numbers without using temp variable using // multiplication and division c = c * d; d = c / d; c = c / d; CrunchifyBetterLog4jLogging.error(logger, "Result Method2 => c: " + c + " d: " + d); } }
Here we are printing different types of logging result.
Result:
2014-06-21 00:23:19,463 DEBUG [CrunchifyBetterLog4jLoggingTest] @@@@@@@@@@ value of a and b before swapping, a: 20 b: 30 @@@@@@@@@@ 2014-06-21 00:23:19,464 WARN [CrunchifyBetterLog4jLoggingTest] ^^^^^^^^^^ Result Method1 => a: 30 b: 20 ^^^^^^^^^^ 2014-06-21 00:23:19,464 INFO [CrunchifyBetterLog4jLoggingTest] ********** value of c and d before swapping, c: 3 d: 4 ********** 2014-06-21 00:23:19,464 ERROR [CrunchifyBetterLog4jLoggingTest] ########## Result Method2 => c: 4 d: 3 ##########