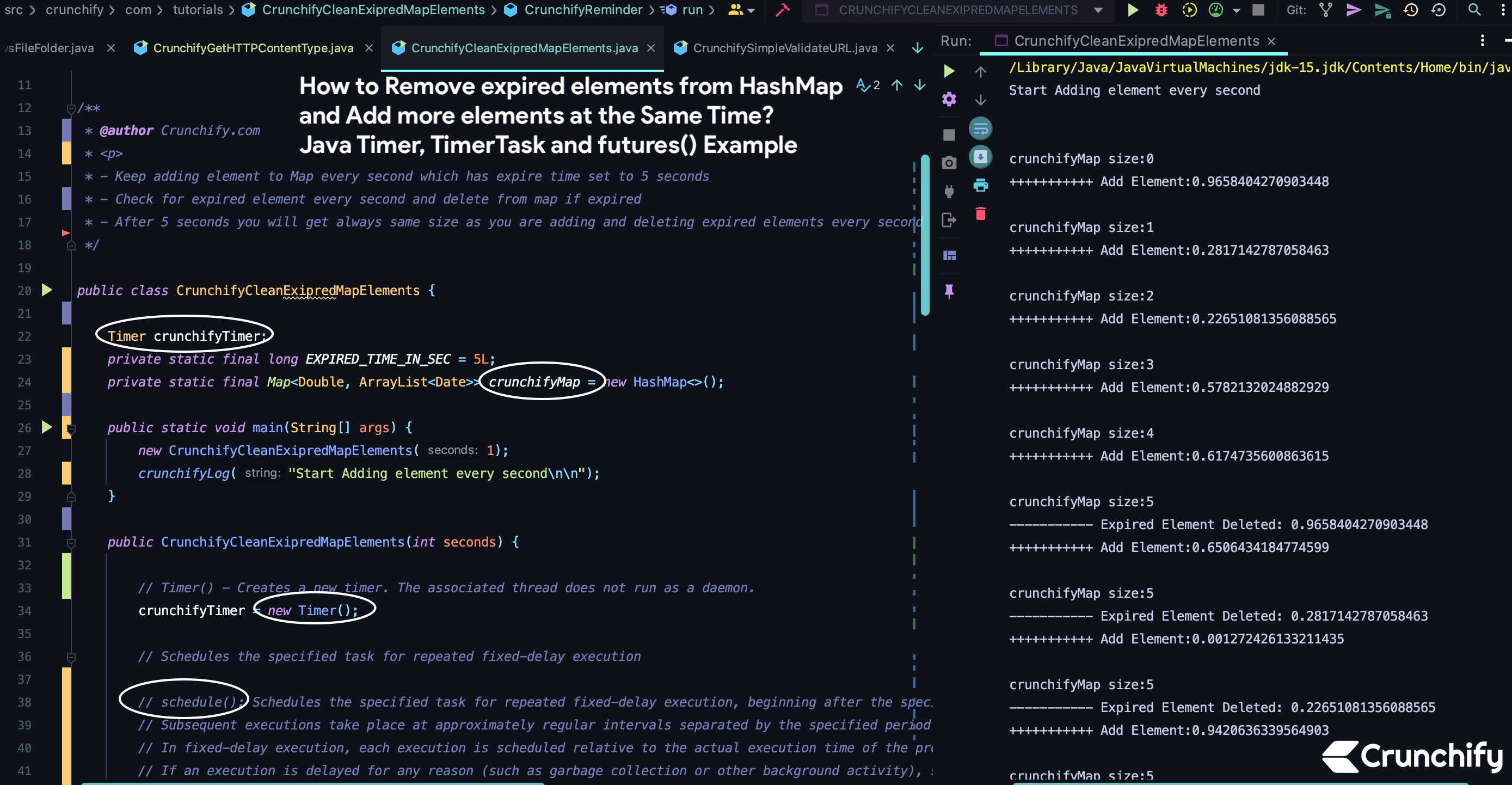
Hashmap, ArrayList, Static Map, Vectors, etc are the most used Java collection framework elements. There are infinite number of scenarios you could use this as per your need.
This example is very interesting Java Example. We are going to perform below operation on single HashMap().
- Create crunchifyMap Object
- Keep adding element to Map every second which has expire time set to
5 seconds
Check
for expired element like cache every second anddelete from map if expired
- After 5 seconds you will get
always same size
andalways 5 elements
as you are adding and deleting expired elements every second
Also if you have below questions
then you are at right place:
- What is Passive Expiring Map Example
- Concurrent Map with timed out element
- Java Cache Map Example
- Java TimerTask Example
- Evictor – a Java Concurrent Map Example
Let’s get started:
Point-1
- Create Timer element
crunchifyTimer
- Schedules the specified task
CrunchifyReminder()
for repeated fixed-delay execution which is 1 second - In scheduled task
add
element into crunchifyMap- check for expired element from crunchifyMap and delete
Point-2
- During
addElement()
operation- We are associating
current time
for each element
- We are associating
- During
crunchifyClearExipredElementsFromMap()
operation- We are checking for current time with element’s time
- If time difference is more than 5 seconds then just delete element from crunchifyMap
Point-3
- During add and remove operation print element on Eclipse console
- 1st added element will be removed 1st and so on
- Please check Eclipse console output for result
Here is a Java program:
package crunchify.com.tutorials; import java.util.ArrayList; import java.util.Date; import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Map.Entry; import java.util.Timer; import java.util.TimerTask; /** * @author Crunchify.com * <p> * - Keep adding element to Map every second which has expire time set to 5 seconds * - Check for expired element every second and delete from map if expired * - After 5 seconds you will get always same size as you are adding and deleting expired elements every second */ public class CrunchifyCleanExipredMapElements { Timer crunchifyTimer; private static final long EXPIRED_TIME_IN_SEC = 5L; private static final Map<Double, ArrayList<Date>> crunchifyMap = new HashMap<>(); public static void main(String[] args) { new CrunchifyCleanExipredMapElements(1); crunchifyLog("Start Adding element every second\n\n"); } public CrunchifyCleanExipredMapElements(int seconds) { // Timer() - Creates a new timer. The associated thread does not run as a daemon. crunchifyTimer = new Timer(); // Schedules the specified task for repeated fixed-delay execution // schedule(): Schedules the specified task for repeated fixed-delay execution, beginning after the specified delay. // Subsequent executions take place at approximately regular intervals separated by the specified period. // In fixed-delay execution, each execution is scheduled relative to the actual execution time of the previous execution. // If an execution is delayed for any reason (such as garbage collection or other background activity), subsequent executions will be delayed as well. // In the long run, the frequency of execution will generally be slightly lower than the reciprocal of the specified period (assuming the system clock underlying Object.wait(long) is accurate). crunchifyTimer.schedule(new CrunchifyReminder(), 0, seconds * 1000L); } // TimeTask: A task that can be scheduled for one-time or repeated execution by a Timer. // A timer task is not reusable. Once a task has been scheduled for execution on a Timer or cancelled, // subsequent attempts to schedule it for execution will throw IllegalStateException. class CrunchifyReminder extends TimerTask { public void run() { // We are checking for expired element from map every second crunchifyClearExpiredElementsFromMap(); // We are adding element every second addElement(); } } public void addElement() { crunchifyAddElementToMap(Math.random()); } // Check for element's expired time. If element is > 5 seconds old then remove it private static void crunchifyClearExpiredElementsFromMap() { // Date() - Allocates a Date object and initializes it so that it represents the time at which it was allocated, measured to the nearest millisecond. Date currentTime = new Date(); Date actualExpiredTime = new Date(); // if element time stamp and current time stamp difference is 5 second then delete element actualExpiredTime.setTime(currentTime.getTime() - EXPIRED_TIME_IN_SEC * 1000L); // size() - Returns the number of key-value mappings in this map. If the map contains more than Integer.MAX_VALUE elements, returns Integer.MAX_VALUE. System.out.println("crunchifyMap size:" + CrunchifyCleanExipredMapElements.crunchifyMap.size()); Iterator<Entry<Double, ArrayList<Date>>> crunchifyIterator = CrunchifyCleanExipredMapElements.crunchifyMap.entrySet().iterator(); // hasNext() - Returns true if the iteration has more elements. (In other words, returns true if next would return an element rather than throwing an exception.) while (crunchifyIterator.hasNext()) { // next() - Returns the next element in the iteration. Entry<Double, ArrayList<Date>> entry = crunchifyIterator.next(); // getValue() - Returns the value corresponding to this entry. // If the mapping has been removed from the backing map (by the iterator's remove operation), the results of this call are undefined. ArrayList<Date> crunchifyElement = entry.getValue(); while (crunchifyElement.size() > 0 && crunchifyElement.get(0).compareTo(actualExpiredTime) < 0) { crunchifyLog("----------- Expired Element Deleted: " + entry.getKey()); crunchifyElement.remove(0); } if (crunchifyElement.size() == 0) { // remove() - Removes from the underlying collection the last element returned by this iterator (optional operation). // This method can be called only once per call to next. crunchifyIterator.remove(); } } } // Adding new element to map with current timestamp private static void crunchifyAddElementToMap(Double digit) { ArrayList<Date> crunchifyList = new ArrayList<>(); CrunchifyCleanExipredMapElements.crunchifyMap.put(digit, crunchifyList); // add() - Appends the specified element to the end of this list. crunchifyList.add(new Date()); crunchifyLog("+++++++++++ Add Element:" + digit + "\n"); } private static void crunchifyLog(String string) { System.out.println(string); } }
Eclipse Console Output:
Just run above program and you should see result as below.
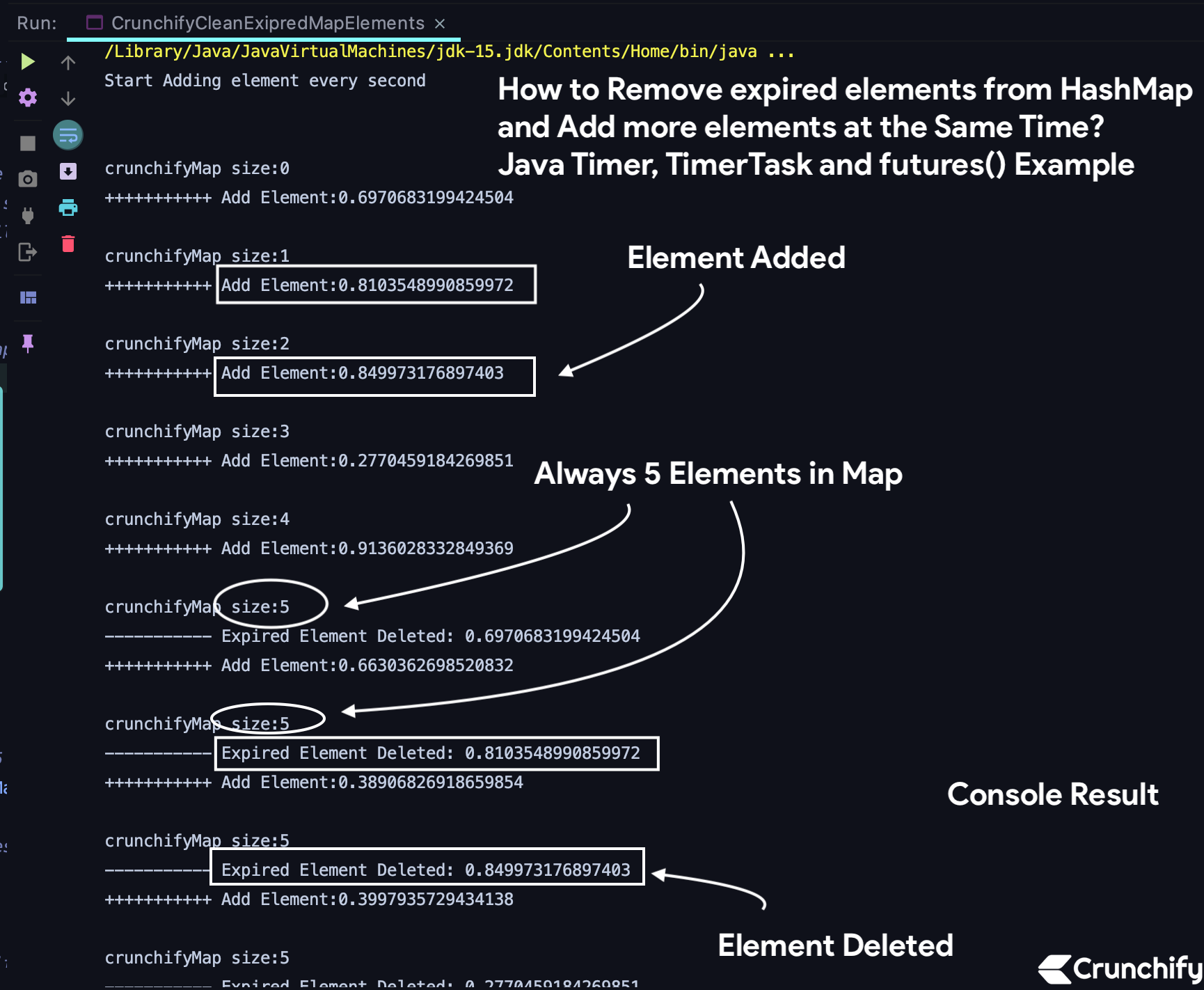
Start Adding element every second crunchifyMap size:0 +++++++++++ Add Element:0.6970683199424504 crunchifyMap size:1 +++++++++++ Add Element:0.8103548990859972 crunchifyMap size:2 +++++++++++ Add Element:0.849973176897403 crunchifyMap size:3 +++++++++++ Add Element:0.2770459184269851 crunchifyMap size:4 +++++++++++ Add Element:0.9136028332849369 crunchifyMap size:5 ----------- Expired Element Deleted: 0.6970683199424504 +++++++++++ Add Element:0.6630362698520832 crunchifyMap size:5 ----------- Expired Element Deleted: 0.8103548990859972 +++++++++++ Add Element:0.38906826918659854 crunchifyMap size:5 ----------- Expired Element Deleted: 0.849973176897403 +++++++++++ Add Element:0.3997935729434138 crunchifyMap size:5 ----------- Expired Element Deleted: 0.2770459184269851 +++++++++++ Add Element:0.20373441843273887 Process finished with exit code 130 (interrupted by signal 2: SIGINT)
Let me know if you face any issue running above Java program.