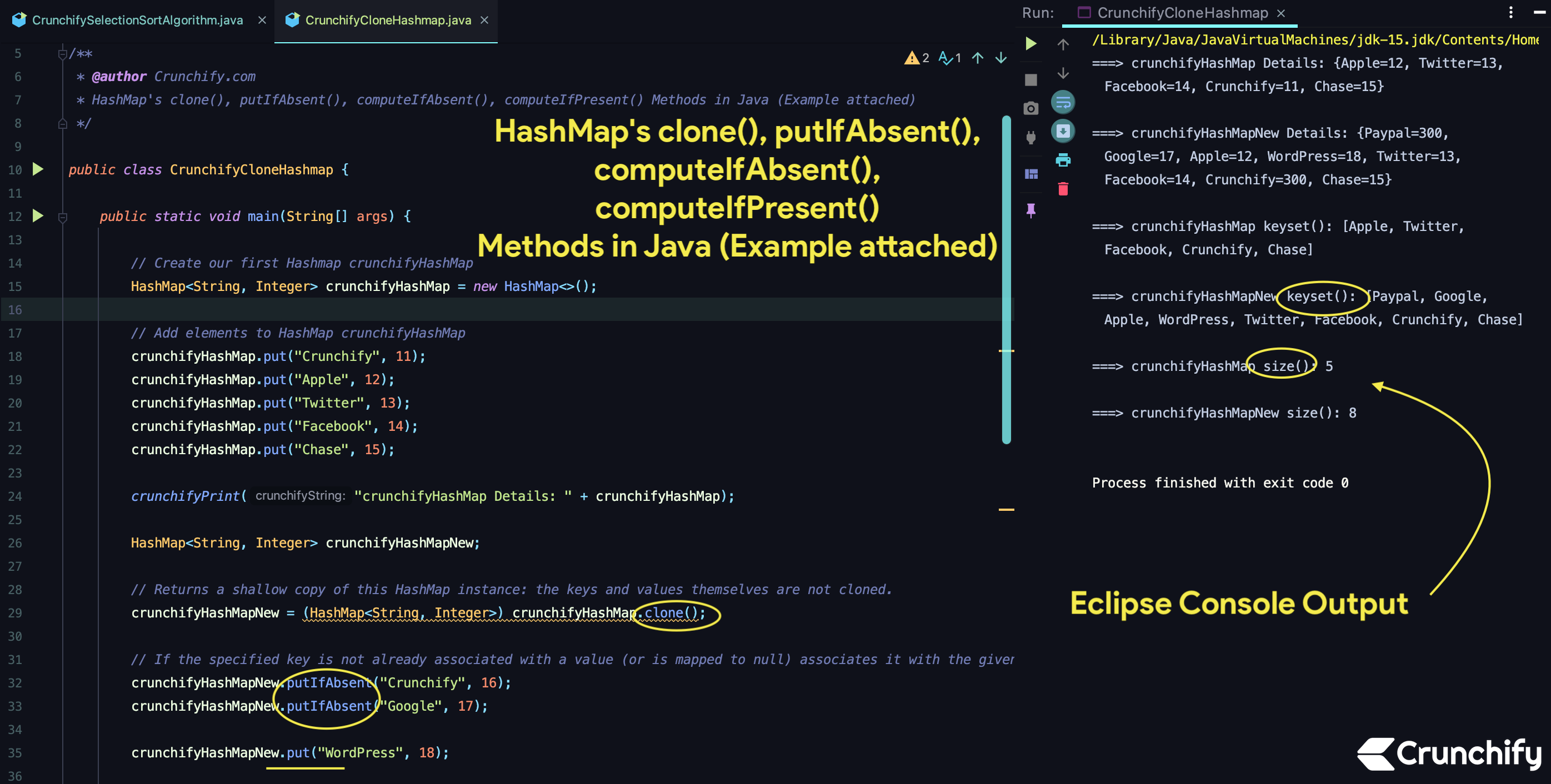
In this Java Tutorial we will go over details on how to use HashMap’s below methods:
- clone()
- putIfAbsent(K key, V value)
- computeIfAbsent(K key, java.util.function.Function mappingFunction)
- computeIfPresent(K key, java.util.function.
BiFunction
remappingFunction)
hashMap.clone():
Returns a shallow copy of this HashMap instance: the keys and values themselves are not cloned.
hashMap.putIfAbsent():
If the specified key is not already associated with a value (or is mapped to null) associates it with the given value and returns null, else returns the current value.
hashMap.computeIfAbsent():
If the specified key is not already associated with a value (or is mapped to null), attempts to compute its value using the given mapping function and enters it into this map unless null.
hashMap.computeIfPresent():
If the value for the specified key is present and non-null, attempts to compute a new mapping given the key and its current mapped value.
Let’s get started:
- Create Java class
CrunchifyCloneHashmap
.java - Put below code into it
package crunchify.com.java.tutorials; import java.util.HashMap; /** * @author Crunchify.com * HashMap's clone(), putIfAbsent(), computeIfAbsent(), computeIfPresent() Methods in Java (Example attached) */ public class CrunchifyCloneHashmap { public static void main(String[] args) { // Create our first Hashmap crunchifyHashMap HashMap<String, Integer> crunchifyHashMap = new HashMap<>(); // Add elements to HashMap crunchifyHashMap crunchifyHashMap.put("Crunchify", 11); crunchifyHashMap.put("Apple", 12); crunchifyHashMap.put("Twitter", 13); crunchifyHashMap.put("Facebook", 14); crunchifyHashMap.put("Chase", 15); crunchifyPrint("crunchifyHashMap Details: " + crunchifyHashMap); HashMap<String, Integer> crunchifyHashMapNew; // Returns a shallow copy of this HashMap instance: the keys and values themselves are not cloned. crunchifyHashMapNew = (HashMap<String, Integer>) crunchifyHashMap.clone(); // If the specified key is not already associated with a value (or is mapped to null) associates it with the given value and returns null, else returns the current value. crunchifyHashMapNew.putIfAbsent("Crunchify", 16); crunchifyHashMapNew.putIfAbsent("Google", 17); crunchifyHashMapNew.put("WordPress", 18); // If the specified key is not already associated with a value (or is mapped to null), attempts to compute its value using the given mapping function and enters it into this map unless null. //If the mapping function returns null, no mapping is recorded. If the mapping function itself throws an (unchecked) exception, the exception is rethrown, and no mapping is recorded. The most common usage is to construct a new object serving as an initial mapped value or memoized result, as in: // // map.computeIfAbsent(key, k -> new Value(f(k))); // //Or to implement a multi-value map, Map<K,Collection<V>>, supporting multiple values per key: // // map.computeIfAbsent(key, k -> new HashSet<V>()).add(v); crunchifyHashMapNew.computeIfAbsent("Paypal", k -> 100 + 200); // If the value for the specified key is present and non-null, attempts to compute a new mapping given the key and its current mapped value. //If the remapping function returns null, the mapping is removed. If the remapping function itself throws an (unchecked) exception, the exception is rethrown, and the current mapping is left unchanged. //The remapping function should not modify this map during computation. //This method will, on a best-effort basis, throw a ConcurrentModificationException if it is detected that the remapping function modifies this map during computation. crunchifyHashMapNew.computeIfPresent("Crunchify", (key, val) -> 300); crunchifyPrint("crunchifyHashMapNew Details: " + crunchifyHashMapNew); // Returns a Set view of the keys contained in this map. The set is backed by the map, so changes to the map are reflected in the set, and vice-versa. If the map is modified while an iteration over the set is in progress (except through the iterator's own remove operation), // the results of the iteration are undefined. The set supports element removal, which removes the corresponding mapping from the map, via // the Iterator.remove, Set.remove, removeAll, retainAll, and clear operations. It does not support the add or addAll operations. crunchifyPrint("crunchifyHashMap keyset(): " + crunchifyHashMap.keySet()); crunchifyPrint("crunchifyHashMapNew keyset(): " + crunchifyHashMapNew.keySet()); // Returns the number of key-value mappings in this map. crunchifyPrint("crunchifyHashMap size(): " + crunchifyHashMap.size()); crunchifyPrint("crunchifyHashMapNew size(): " + crunchifyHashMapNew.size()); } private static void crunchifyPrint(String crunchifyString) { System.out.println("===> " + crunchifyString + "\n"); } }
In above Java code, you wont see crunchifyHashMapNew.putIfAbsent(“Crunchify”, 16); added to new HashMap.
Just run above program as a Java Application in either in Eclipse IDE or IntelliJ IDEA.
You should see console result similar to this:
/Library/Java/JavaVirtualMachines/jdk-15.jdk/Contents/Home/bin/java -javaagent:/Applications/IntelliJ 5.1.3.RELEASE.jar crunchify.com.java.tutorials.CrunchifyCloneHashmap ===> crunchifyHashMap Details: {Apple=12, Twitter=13, Facebook=14, Crunchify=11, Chase=15} ===> crunchifyHashMapNew Details: {Paypal=300, Google=17, Apple=12, WordPress=18, Twitter=13, Facebook=14, Crunchify=300, Chase=15} ===> crunchifyHashMap keyset(): [Apple, Twitter, Facebook, Crunchify, Chase] ===> crunchifyHashMapNew keyset(): [Paypal, Google, Apple, WordPress, Twitter, Facebook, Crunchify, Chase] ===> crunchifyHashMap size(): 5 ===> crunchifyHashMapNew size(): 8 Process finished with exit code 0
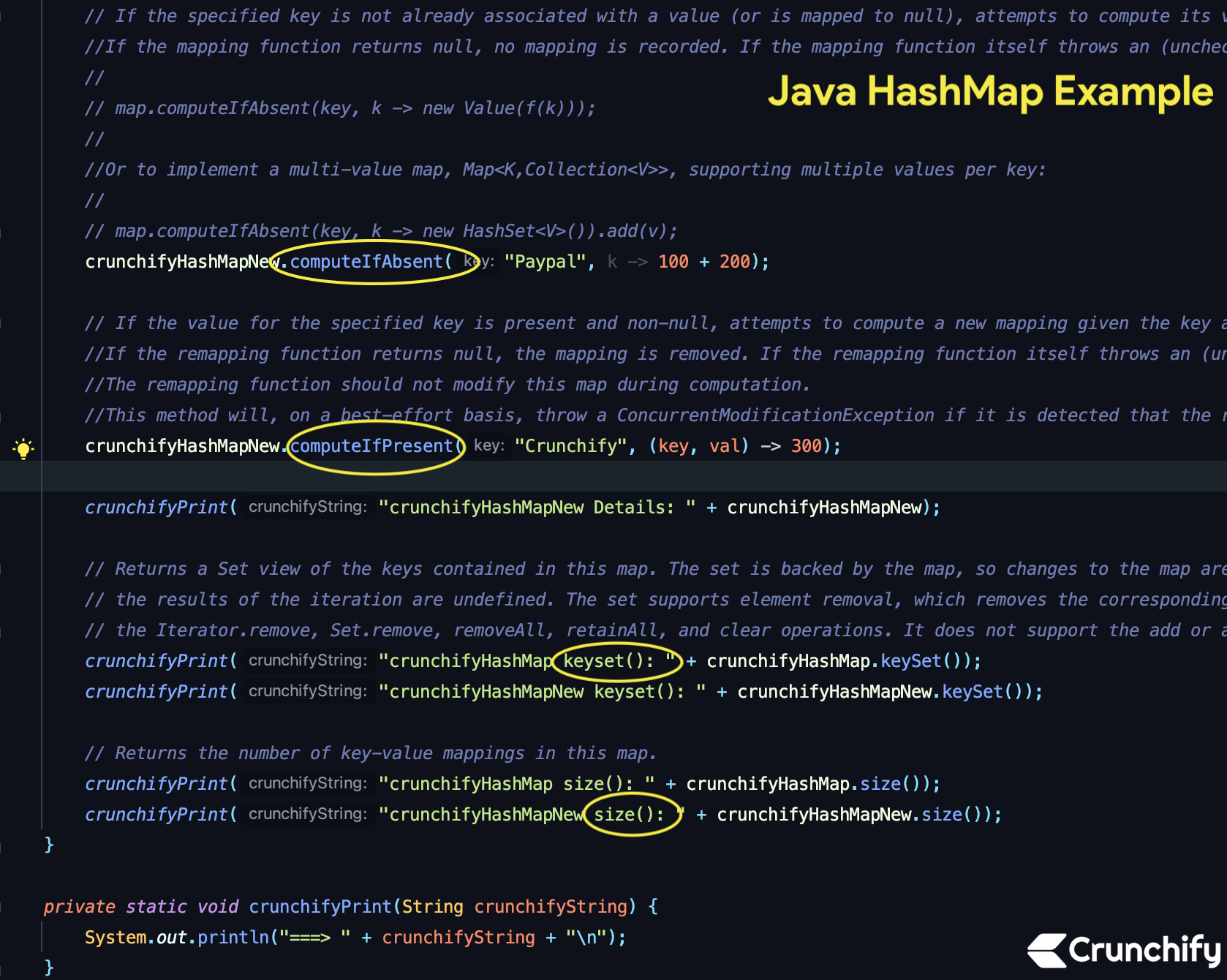
Let me know if you face any issue running code.