Scan this: You will be redirected to https://crunchify.com
QR code
(abbreviated from Quick Response Code
) is the trademark for a type of matrix barcode (or two-dimensional barcode) first designed for the automotive industry in Japan. Bar codes are optical machine-readable labels attached to items that record information related to the item. Initially patented, its patent holder has chosen not to exercise those rights. Recently, the QR Code system has become popular outside the automotive industry due to its fast readability and greater storage capacity compared to standard UPC barcodes.
The code consists of black modules (square dots) arranged in a square grid on a white background. ZXING
is a Multi-format 1D/2D barcode image processing library with clients for Android, Java. It is an open-source, multi-format 1D/2D barcode image processing library implemented in Java, with ports to other languages.
Checkout live example:
Our focus is on using the built-in camera on mobile phones to scan and decode barcodes on the device, without communicating with a server. However the project can be used to encode and decode barcodes on desktops and servers as well.
Here is a simple Java Code which generates QR code for you.
package crunchify.com.tutorials; import com.google.zxing.BarcodeFormat; import com.google.zxing.EncodeHintType; import com.google.zxing.WriterException; import com.google.zxing.common.BitMatrix; import com.google.zxing.qrcode.QRCodeWriter; import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel; import javax.imageio.ImageIO; import java.awt.*; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import java.util.EnumMap; import java.util.Map; /** * @author Crunchify.com * Simple QR Code Generator Example - Create QR codes for free. */ public class CrunchifyQRCodeGenerator { public static void main(String[] args) { String myCodeText = "https://crunchify.com"; String filePath = "/Users/app/Document/Crunchify.com-QRCode.png"; int size = 512; String crunchifyFileType = "png"; File crunchifyFile = new File(filePath); try { Map<EncodeHintType, Object> crunchifyHintType = new EnumMap<EncodeHintType, Object>(EncodeHintType.class); crunchifyHintType.put(EncodeHintType.CHARACTER_SET, "UTF-8"); // Now with version 3.4.1 you could change margin (white border size) crunchifyHintType.put(EncodeHintType.MARGIN, 1); /* default = 4 */ Object put = crunchifyHintType.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H); QRCodeWriter mYQRCodeWriter = new QRCodeWriter(); // throws com.google.zxing.WriterException BitMatrix crunchifyBitMatrix = mYQRCodeWriter.encode(myCodeText, BarcodeFormat.QR_CODE, size, size, crunchifyHintType); int CrunchifyWidth = crunchifyBitMatrix.getWidth(); // The BufferedImage subclass describes an Image with an accessible buffer of crunchifyImage data. BufferedImage crunchifyImage = new BufferedImage(CrunchifyWidth, CrunchifyWidth, BufferedImage.TYPE_INT_RGB); // Creates a Graphics2D, which can be used to draw into this BufferedImage. crunchifyImage.createGraphics(); // This Graphics2D class extends the Graphics class to provide more sophisticated control over geometry, coordinate transformations, color management, and text layout. // This is the fundamental class for rendering 2-dimensional shapes, text and images on the Java(tm) platform. Graphics2D crunchifyGraphics = (Graphics2D) crunchifyImage.getGraphics(); // setColor() sets this graphics context's current color to the specified color. // All subsequent graphics operations using this graphics context use this specified color. crunchifyGraphics.setColor(Color.white); // fillRect() fills the specified rectangle. The left and right edges of the rectangle are at x and x + width - 1. crunchifyGraphics.fillRect(0, 0, CrunchifyWidth, CrunchifyWidth); // TODO: Please change this color as per your need crunchifyGraphics.setColor(Color.BLUE); for (int i = 0; i < CrunchifyWidth; i++) { for (int j = 0; j < CrunchifyWidth; j++) { if (crunchifyBitMatrix.get(i, j)) { crunchifyGraphics.fillRect(i, j, 1, 1); } } } // A class containing static convenience methods for locating // ImageReaders and ImageWriters, and performing simple encoding and decoding. ImageIO.write(crunchifyImage, crunchifyFileType, crunchifyFile); System.out.println("\nCongratulation.. You have successfully created QR Code.. \n" + "Check your code here: " + filePath); } catch (WriterException e) { System.out.println("\nSorry.. Something went wrong...\n"); e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Output: (Scan it by yourself)
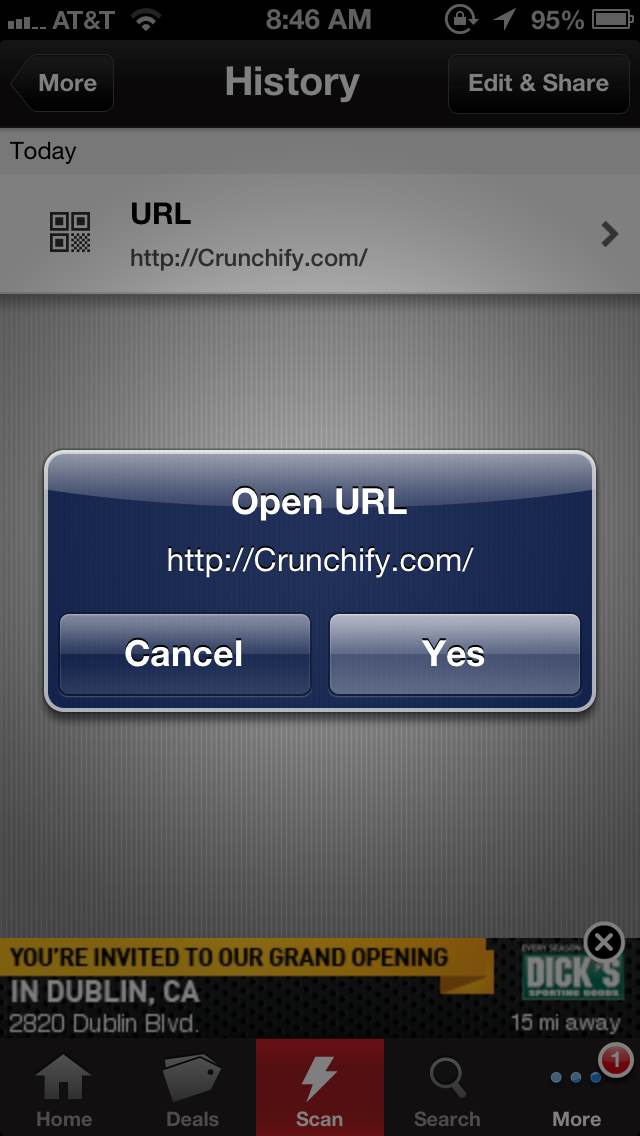
Which library do I have to download?
If you have Maven project then include this dependency to your pom.xml file.
<dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.4.1</version> </dependency>
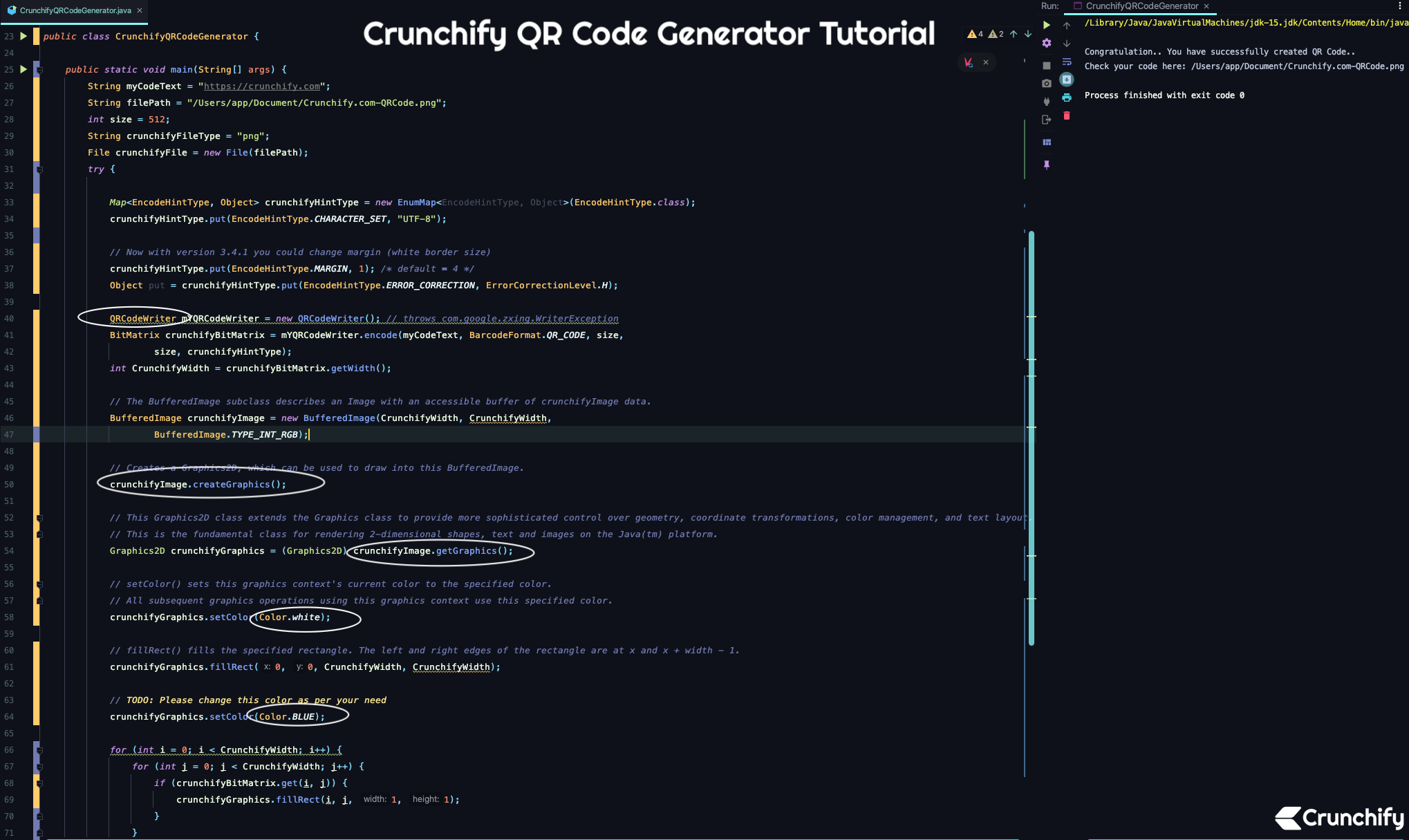
OR Download .jar file manually
Step-1:
Download link.
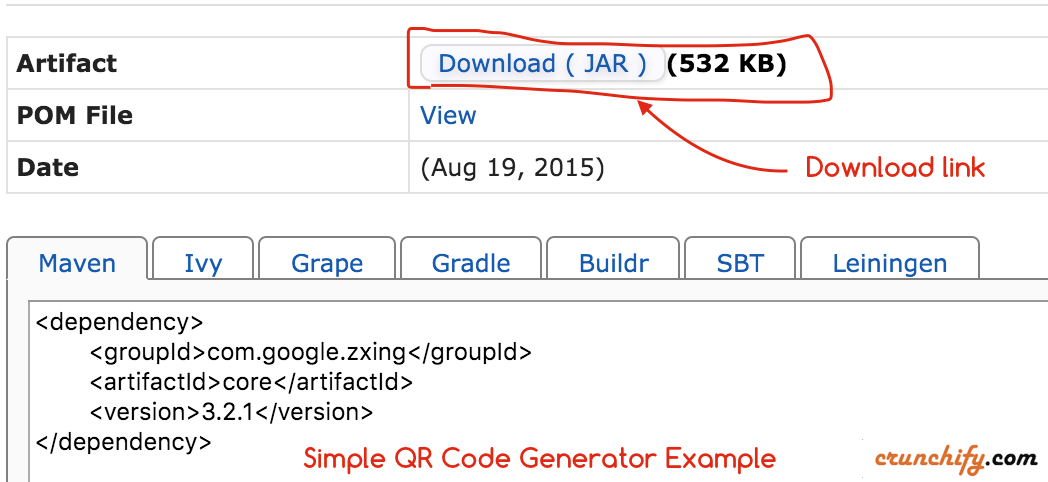
Step-2
Once you download it you have to include it into your project’s classpath. How to add .jar file to Project Build Path in Eclipse.
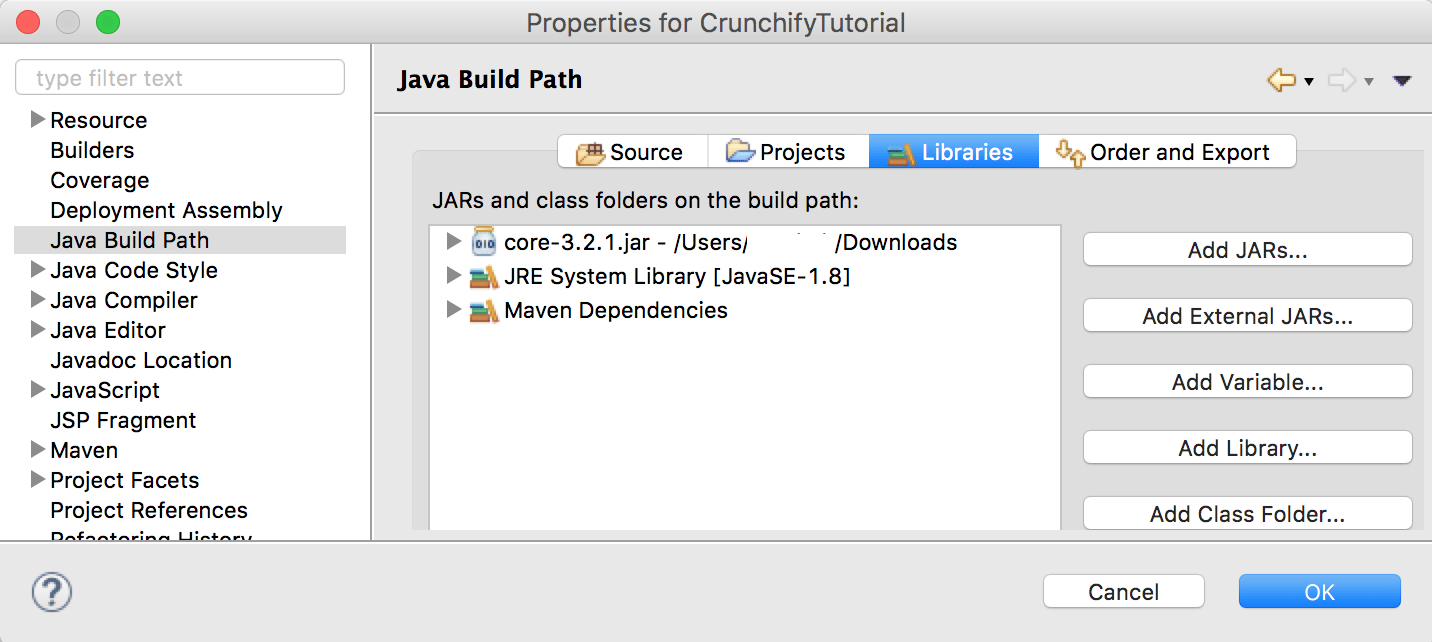
Let me know if you face any issue generating QR code.