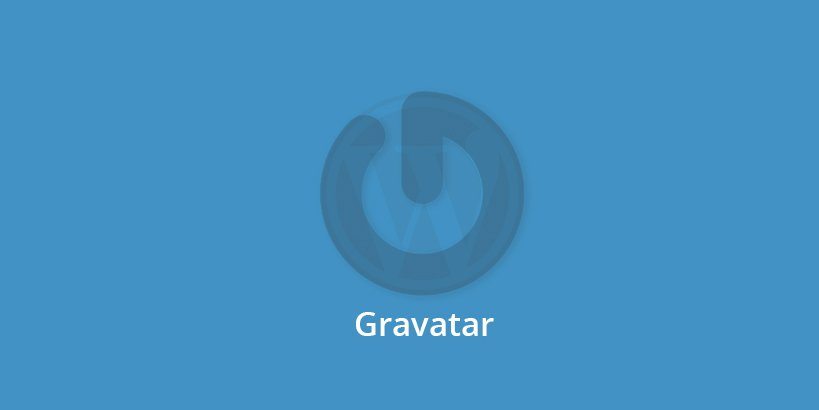
I see lots of tutorials about how to get the most recent comments in WordPress. Irrespective of how popular is your blog but it’s always good idea to show commenter’s gravatar in sidebar. Then what is the best way to show most recent comments on your WordPress blog with Gravatar?
This PHP code retrieves and displays the 10 most recent approved comments from your WordPress database, along with their associated Gravatars.
Simply put below code to your theme’s file.
<?php global $wpdb; $query = "SELECT * from $wpdb->comments WHERE comment_approved= '1' ORDER BY comment_date DESC LIMIT 0 ,10"; // This shows top 10 comments $comments = $wpdb->get_results($query); if ($comments) { echo '<ul>'; foreach ($comments as $comment) { $url = '<a href="'. get_permalink($comment->comment_post_ID).'#comment-'.$comment->comment_ID .'" title="'.$comment->comment_author .' | '.get_the_title($comment->comment_post_ID).'">'; echo '<li>'; echo '<div class="img">'; echo $url; echo get_avatar( $comment->comment_author_email, $img_w); // Get Gravatar Image echo '</a></div>'; echo '<div class="txt">Par: '; echo $url; echo $comment->comment_author; echo '</a></div>'; echo '</li>'; } echo '</ul>'; } ?>
Let’s break down what each part of the code does:
Globalizing the $wpdb
Object:
It starts by globalizing the $wpdb
(WordPress database) object, which allows you to interact with the database directly.
SQL Query:
The code constructs an SQL query to retrieve comments from the database:
$query = "SELECT * from $wpdb->comments WHERE comment_approved= '1' ORDER BY comment_date DESC LIMIT 0 ,10";
- It selects all columns (
*
) from thewp_comments
table (using$wpdb->comments
) where thecomment_approved
field is equal to ‘1’, indicating approved comments. - The comments are ordered in descending order of their
comment_date
, meaning the most recent comments will appear first. - The
LIMIT
clause restricts the query to return a maximum of 10 comments.
Executing the SQL Query:
The code then executes the SQL query and stores the results in the $comments
variable using $wpdb->get_results($query)
.
Checking for Comments:
It checks if there are any comments to display (if ($comments) {
).
Loop Through Comments:
Inside the loop, it iterates through each comment retrieved from the database and generates HTML to display the comment information:
- The comment author’s Gravatar is displayed using the
get_avatar
function:
echo get_avatar( $comment->comment_author_email, $img_w);
$comment->comment_author_email
provides the email address of the comment author.$img_w
is a variable that should be defined elsewhere in your code. It determines the size of the Gravatar image.- The comment author’s name is displayed along with a link to the comment’s corresponding post and the post’s title
$url = '<a href="'. get_permalink($comment->comment_post_ID).'#comment-'.$comment->comment_ID .'" title="'.$comment->comment_author .' | '.get_the_title($comment->comment_post_ID).'">'; echo '<li>'; echo '<div class="img">'; echo $url; echo get_avatar( $comment->comment_author_email, $img_w); // Get Gravatar Image echo '</a></div>'; echo '<div class="txt">Par: '; echo $url; echo $comment->comment_author; echo '</a></div>'; echo '</li>';
The code generates an unordered list (<ul>
) to wrap the comments and list items (<li>
) for each comment.
Closing Tags:
The code properly closes the HTML tags to ensure valid markup.
Let me know what you think about this.