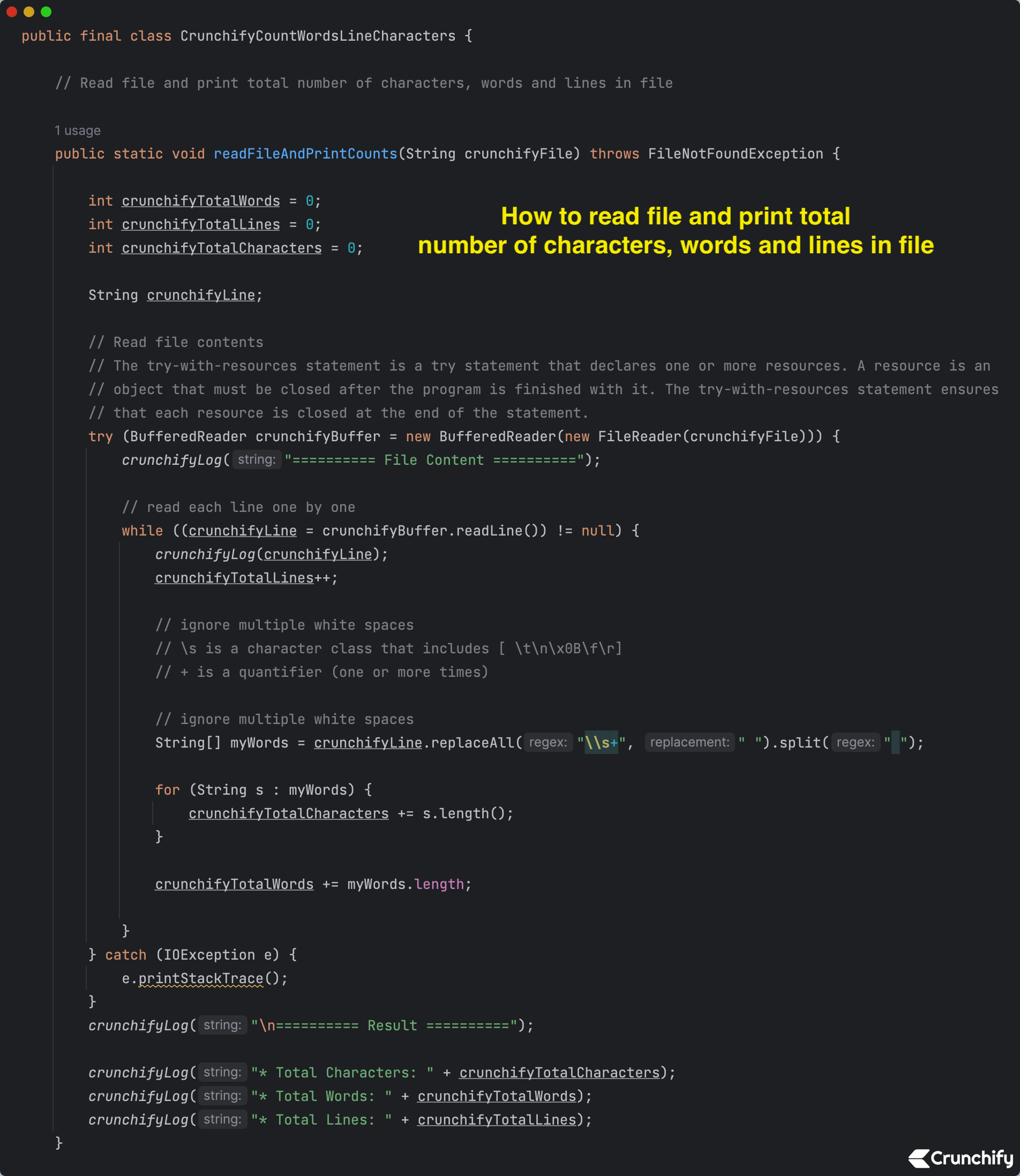
Master the art of efficient text file analysis in Java with our insightful guide. Learn how to count characters, words, and lines using built-in features. Optimize your file processing techniques and elevate your Java development skills.
Recently I got an email asking for “Can I have a tutorial on counting total number of words
, lines
and characters
from file?”
Some time back I’ve written an article on How to Read a File Line by Line in Reverse Order which doesn’t print above requested metrics. In this tutorial we will go over steps on how to print total number of Characters, Words and Lines for a given file.
Let’s get started:
- We will read file
crunchify.txt
first located at/Users/appshah/Downloads/
folder. I’m running program on my Macbook Pro so replace username fromappshah
with your actual name. - We will read each line and will remove multiple whitespace using regex
\\s
- Print file and results on console
If you need more information on REGEX pattern in java then follow below tutorials:
- Matcher (java.util.regex.Matcher) Tutorial
- What is RegEx Pattern (Regular Expression)? How to use it in Java?
File we are using:
<?php // Silence is golden. This is Crunchify's tutorial which counts total number of char, words and lines... ?>
Detailed Explanation:
Program is written to read a text file, analyze its content, and then output the total number of characters, words, and lines in the file. It uses a buffered reader to read the file line by line, splits each line into words, and performs the necessary calculations to determine the counts.
- Import Statements: The code includes necessary import statements to use classes for file reading and exception handling.
- Class Definition and Main Method:
CrunchifyCountWordsLineCharacters
is the main class that contains the functionality to count characters, words, and lines in a text file.- The
main
method is the entry point of the program. It reads a specific file (crunchifyFile
) and calls thereadFileAndPrintCounts
method.
readFileAndPrintCounts
Method:- This method takes a file path as a parameter and performs the following steps:
- Initializes counters for total words, total lines, and total characters.
- Opens a buffered reader using a try-with-resources block to automatically close the reader when done.
- Reads each line from the file using
readLine()
until there are no more lines. - For each line, it increments the line count and then splits the line into words using spaces as separators.
- It processes each word in the line to count the characters and adds the word count.
- After processing all lines, it prints the result, including the total characters, words, and lines.
- This method takes a file path as a parameter and performs the following steps:
crunchifyLog
Method:- A simple utility method that prints a given string to the console.
- Main Method (
main
):- This is where the program starts executing.
- The
crunchifyFile
variable stores the path to the input text file. - The
readFileAndPrintCounts
method is called with the file path, and anyFileNotFoundException
is caught and printed.
- Comments:
- The code contains comments that explain its purpose and some of the key steps within the methods.
Java code:
package crunchify.com.tutorials; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; /** * @author Crunchify.com * Program: CrunchifyCountWordsLineCharacters.java * Details: Read file and print total number of characters, words and lines in file * */ public class CrunchifyCountWordsLineCharacters { // Read file and print total number of characters, words and lines in file public static void readFileAndPrintCounts(String crunchifyFile) throws FileNotFoundException { int crunchifyTotalWords = 0; int crunchifyTotalLines = 0; int crunchifyTotalCharacters = 0; String crunchifyLine; // Read file contents // The try-with-resources statement is a try statement that declares one or more resources. A resource is an // object that must be closed after the program is finished with it. The try-with-resources statement ensures // that each resource is closed at the end of the statement. try (BufferedReader crunchifyBuffer = new BufferedReader(new FileReader(crunchifyFile))) { crunchifyLog("========== File Content =========="); // read each line one by one while ((crunchifyLine = crunchifyBuffer.readLine()) != null) { crunchifyLog(crunchifyLine); crunchifyTotalLines++; // ignore multiple white spaces // \s is a character class that includes [ \t\n\x0B\f\r] // + is a quantifier (one or more times) // ignore multiple white spaces String[] myWords = crunchifyLine.replaceAll("\\s+", " ").split(" "); for (String s : myWords) { crunchifyTotalCharacters += s.length(); } crunchifyTotalWords += myWords.length; } } catch (IOException e) { e.printStackTrace(); } crunchifyLog("\n========== Result =========="); crunchifyLog("* Total Characters: " + crunchifyTotalCharacters); crunchifyLog("* Total Words: " + crunchifyTotalWords); crunchifyLog("* Toal Lines: " + crunchifyTotalLines); } private static void crunchifyLog(String string) { System.out.println(string); } public static void main(String[] args) { try { String crunchifyFile = "/Users/appshah/Downloads/crunchify.txt"; readFileAndPrintCounts(crunchifyFile); } catch (FileNotFoundException e) { e.printStackTrace(); } } }
Console Output:
========== File Content ========== <?php // Silence is golden. This is Crunchify's tutorial which counts total number of char, words and lines... ?> ========== Result ========== * Total Characters: 95 * Total Words: 21 * Total Lines: 6
Functionality revolves around reading a text file, analyzing its content, and providing statistics on the total number of characters, words, and lines.
It demonstrates how to use a buffered reader to efficiently read and process text files while calculating different statistics.