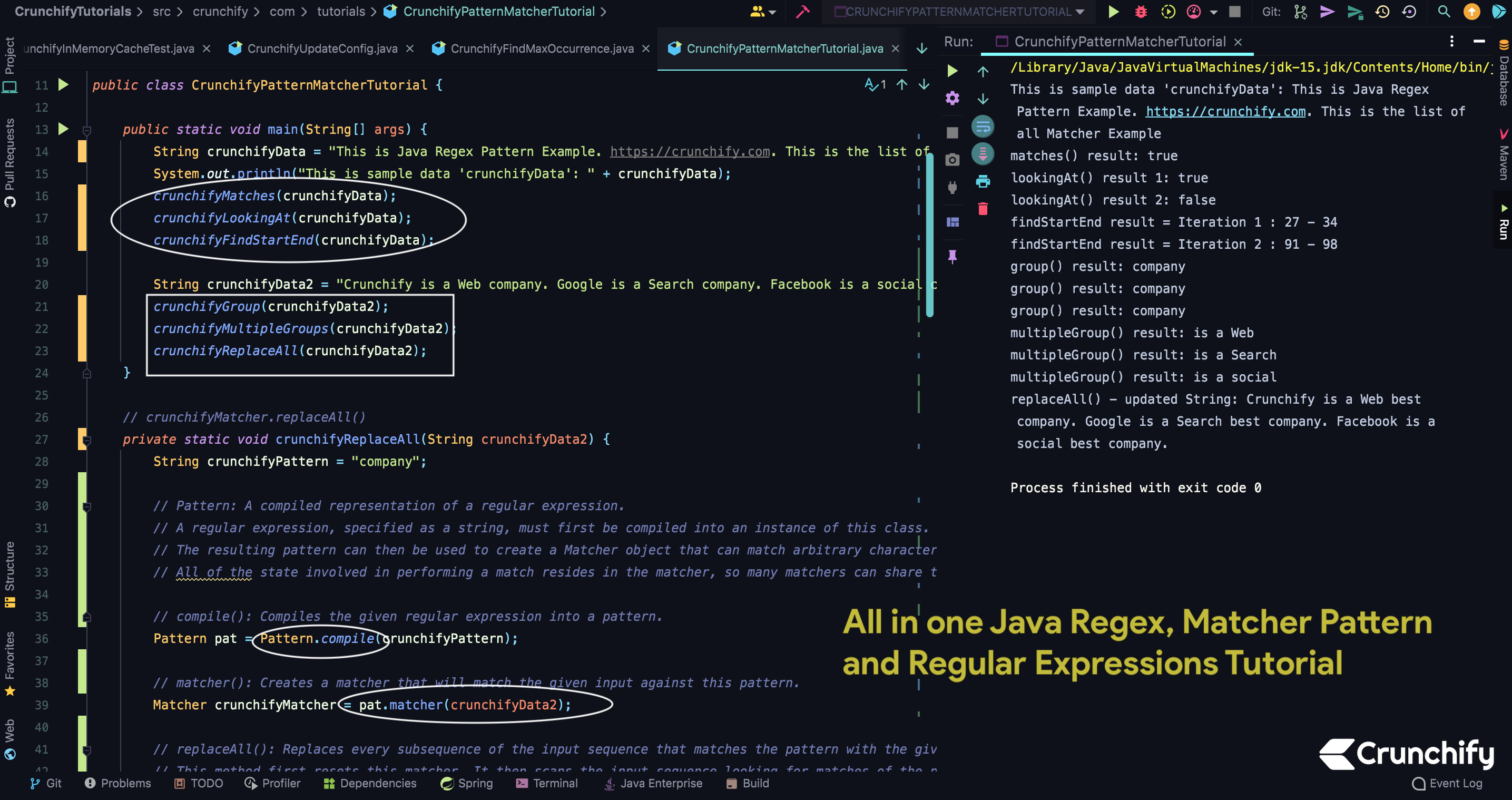
In this tutorial we will go over list of Matcher (java.util.regex.Matcher
) APIs. Sometime back I’ve written a tutorial on Java Regex which covers wide variety of samples.
Regular Expression is a search pattern for String. java.util.regex
Classes for matching character sequences against patterns specified by regular expressions in Java.
This tutorial best works if you have any of below questions:
- Java Regex – Tutorial
- Java Regular Expressions Tutorials
- What is regular Expression in Java?
- What is pattern in Java?
- java pattern matcher example and java regex special characters
Let’s get started.
- Create class
CrunchifyPatternMatcherTutorial.java
- Create different methods to check below Matcher APIs:
- matches()
- lookingAt()
- findStartEnd()
- group()
- multipleGroups()
- replaceAll()
- Print result of each APIs.
matches()
matches()
tries to match entire string against the pattern. It returns true
if, and only if
, the entire region sequence matches this matcher’s pattern.
lookingAt()
lookingAt() functionality is exactly same as matches() except it tries to match the input sequence, starting at the beginning of the region, against the pattern. It returns true if, and only if, a prefix of the input sequence matches this matcher’s pattern.
find(), start() and end()
find()
tries to find the next subsequence of the input sequence that matches the pattern. start()
tries to returns the start index of the previous match and end()
tries to returns the end index of the previous match.
group()
group()
returns the input subsequence matched by the previous match. It’s like match between start() and end().
multipleGroups
multiplegroups
could be represented by “(String) (String)”.
Sample: String crunchifyPattern = "(is) (.+?) (.+?) ";
replaceAll()
replaceAll()
replaces every subsequence of the input sequence that matches the pattern with the given replacement string.
Here is a complete example:
package crunchify.com.tutorials; import java.util.regex.Matcher; import java.util.regex.Pattern; /** * @author Crunchify.com * All in one Java Regex, Matcher Pattern and Regular Expressions Tutorial. */ public class CrunchifyPatternMatcherTutorial { public static void main(String[] args) { String crunchifyData = "This is Java Regex Pattern Example. https://crunchify.com. This is the list of all Matcher Example"; System.out.println("This is sample data 'crunchifyData': " + crunchifyData); crunchifyMatches(crunchifyData); crunchifyLookingAt(crunchifyData); crunchifyFindStartEnd(crunchifyData); String crunchifyData2 = "Crunchify is a Web company. Google is a Search company. Facebook is a social company."; crunchifyGroup(crunchifyData2); crunchifyMultipleGroups(crunchifyData2); crunchifyReplaceAll(crunchifyData2); } // crunchifyMatcher.replaceAll() private static void crunchifyReplaceAll(String crunchifyData2) { String crunchifyPattern = "company"; // Pattern: A compiled representation of a regular expression. // A regular expression, specified as a string, must first be compiled into an instance of this class. // The resulting pattern can then be used to create a Matcher object that can match arbitrary character sequences against the regular expression. // All of the state involved in performing a match resides in the matcher, so many matchers can share the same pattern. // compile(): Compiles the given regular expression into a pattern. Pattern pat = Pattern.compile(crunchifyPattern); // matcher(): Creates a matcher that will match the given input against this pattern. Matcher crunchifyMatcher = pat.matcher(crunchifyData2); // replaceAll(): Replaces every subsequence of the input sequence that matches the pattern with the given replacement string. // This method first resets this matcher. It then scans the input sequence looking for matches of the pattern. Characters that are not part of any match are appended directly to the result string; each match is replaced in the result by the replacement string. // The replacement string may contain references to captured subsequences as in the appendReplacement method. String updatedString = crunchifyMatcher.replaceAll("best company"); System.out.println("replaceAll() - updated String: " + updatedString); } private static void crunchifyMultipleGroups(String crunchifyData2) { String crunchifyPattern = "(is) (.+?) (.+?) "; Pattern pat = Pattern.compile(crunchifyPattern); Matcher crunchifyMatcher = pat.matcher(crunchifyData2); // find(): Attempts to find the next subsequence of the input sequence that matches the pattern. while (crunchifyMatcher.find()) { // group(): Returns the input subsequence matched by the previous match. // For a matcher m with input sequence s, the expressions m.group() and s.substring(m.start(), m. end()) are equivalent. // Note that some patterns, for example a*, match the empty string. // This method will return the empty string when the pattern successfully matches the empty string in the input. System.out.println("multipleGroup() result: " + crunchifyMatcher.group()); } } // crunchifyMatcher.group() private static void crunchifyGroup(String crunchifyData2) { String crunchifyPattern = "company"; Pattern pat = Pattern.compile(crunchifyPattern); Matcher crunchifyMatcher = pat.matcher(crunchifyData2); while (crunchifyMatcher.find()) { System.out.println("group() result: " + crunchifyMatcher.group()); } } // crunchifyMatcher.find() - start() - end() private static void crunchifyFindStartEnd(String crunchifyData) { String crunchifyPattern = "Example"; Pattern pat = Pattern.compile(crunchifyPattern); Matcher crunchifyMatcher = pat.matcher(crunchifyData); int totalCount = 0; while (crunchifyMatcher.find()) { totalCount++; // start(): Returns the start index of the previous match. // end(): Returns the offset after the last character matched. System.out.println("findStartEnd result = Iteration " + totalCount + " : " + crunchifyMatcher.start() + " - " + crunchifyMatcher.end()); } } // crunchifyMatcher.lookingAt() private static void crunchifyLookingAt(String crunchifyData) { String crunchifyPattern = "This is Java"; Pattern pat = Pattern.compile(crunchifyPattern); Matcher crunchifyMatcher = pat.matcher(crunchifyData); // lookingAt(): Attempts to match the input sequence, starting at the beginning of the region, against the pattern. boolean isLookingAt = crunchifyMatcher.lookingAt(); System.out.println("lookingAt() result 1: " + isLookingAt); crunchifyPattern = " is Java"; pat = Pattern.compile(crunchifyPattern); crunchifyMatcher = pat.matcher(crunchifyData); isLookingAt = crunchifyMatcher.lookingAt(); System.out.println("lookingAt() result 2: " + isLookingAt); } // crunchifyMatcher.matches() public static void crunchifyMatches(String crunchifyData) { String crunchifyPattern = ".*https://.*"; Pattern pat = Pattern.compile(crunchifyPattern); Matcher crunchifyMatcher = pat.matcher(crunchifyData); boolean isMatched = crunchifyMatcher.matches(); System.out.println("matches() result: " + isMatched); } }
Eclipse Console Result:
This is sample data 'crunchifyData': This is Java Regex Pattern Example. https://crunchify.com. This is the list of all Matcher Example matches() result: true lookingAt() result 1: true lookingAt() result 2: false findStartEnd result = Iteration 1 : 27 - 34 findStartEnd result = Iteration 2 : 90 - 97 group() result: company group() result: company group() result: company multipleGroup() result: is a Web multipleGroup() result: is a Search multipleGroup() result: is a social replaceAll() - updated String: Crunchify is a Web best company. Google is a Search best company. Facebook is a social best company.
Let me know if you have any question running above program.