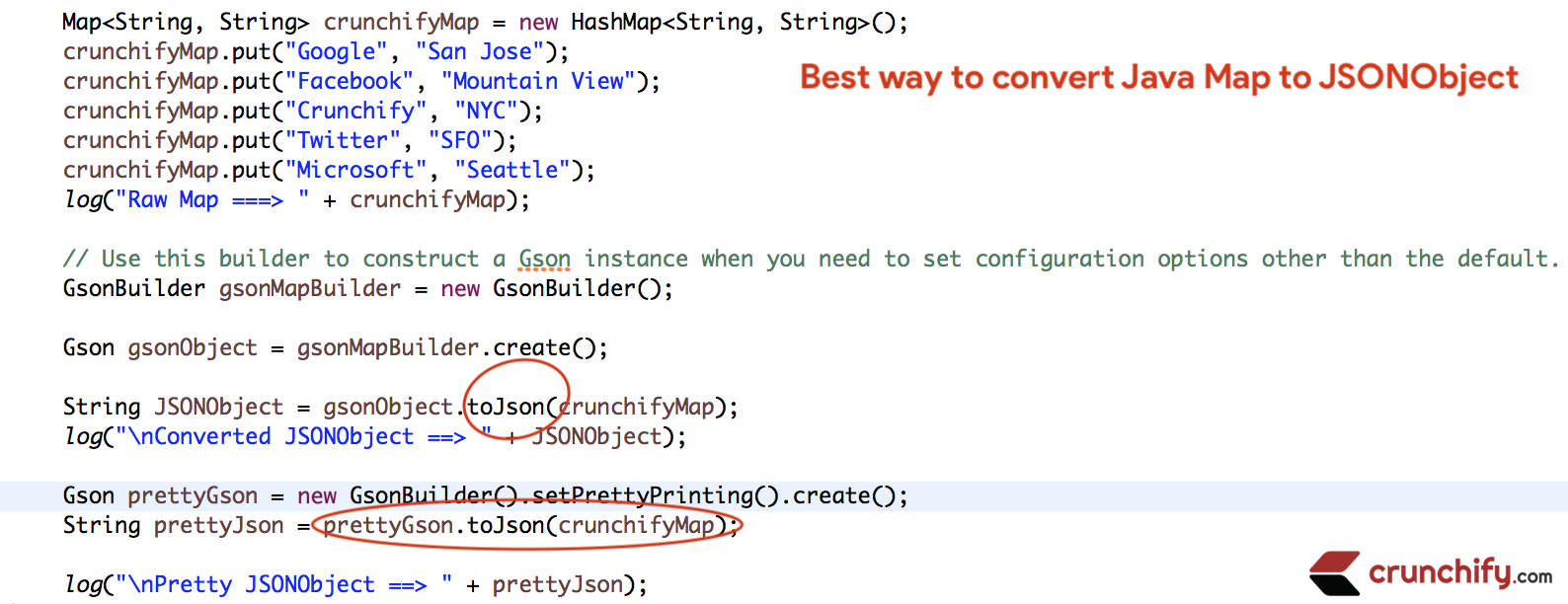
Converting Objects from one form to another is a common request. There are 4 different ways
to convert Java Map/HashMap to JSONObject.
We will go over details on how to convert HashMap to JSONObject in this tutorial.
Let’s get started:
Create class CrunchifyMapToJsonObject.java.
Method-1
Firstly we use Google GSON dependency
to convert HashMap to JSONObject. You need below Maven dependency in your project.
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.0</version> </dependency>
Method-2
Next we will use org.json dependency
using new JSONObject().
<dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20180130</version> </dependency>
Method-3
Using jackson-core dependency
with ObjectMapper().writeValueAsString() operation.
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.9.5</version> <scope>compile</scope> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.9.5</version> <scope>compile</scope> </dependency>
Method-4
Using json-simple dependency
with JSONValue.toJSONString() library.
<dependency> <groupId>com.googlecode.json-simple</groupId> <artifactId>json-simple</artifactId> <version>1.1.1</version> </dependency>
Make sure to add all above maven dependencies to your Java J2EE project. If you don’t have maven project then follow these steps.
Here is a complete example:
package crunchify.com.tutorial; import java.util.HashMap; import java.util.Map; import org.json.JSONObject; import org.json.simple.JSONValue; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import com.google.gson.Gson; import com.google.gson.GsonBuilder; /** * @author Crunchify.com * Program: 4 Best ways to convert Java Map to JSONObject. * Version: 1.0.0 * */ public class CrunchifyMapToJsonObject { public static void main(String a[]) { Map<String, String> crunchifyMap = new HashMap<String, String>(); crunchifyMap.put("Google", "San Jose"); crunchifyMap.put("Facebook", "Mountain View"); crunchifyMap.put("Crunchify", "NYC"); crunchifyMap.put("Twitter", "SFO"); crunchifyMap.put("Microsoft", "Seattle"); log("Raw Map ===> " + crunchifyMap); // Use this builder to construct a Gson instance when you need to set configuration options other than the default. GsonBuilder gsonMapBuilder = new GsonBuilder(); Gson gsonObject = gsonMapBuilder.create(); String JSONObject = gsonObject.toJson(crunchifyMap); log("\nMethod-1: Using Google GSON ==> " + JSONObject); Gson prettyGson = new GsonBuilder().setPrettyPrinting().create(); String prettyJson = prettyGson.toJson(crunchifyMap); log("\nPretty JSONObject ==> " + prettyJson); // Construct a JSONObject from a Map. JSONObject crunchifyObject = new JSONObject(crunchifyMap); log("\nMethod-2: Using new JSONObject() ==> " + crunchifyObject); try { // Default constructor, which will construct the default JsonFactory as necessary, use SerializerProvider as its // SerializerProvider, and BeanSerializerFactory as its SerializerFactory. String objectMapper = new ObjectMapper().writeValueAsString(crunchifyMap); log("\nMethod-3: Using ObjectMapper().writeValueAsString() ==> " + objectMapper); } catch (JsonProcessingException e) { e.printStackTrace(); } // Convert an object to JSON text. If this object is a Map or a List, and it's also a JSONAware, JSONAware will be considered firstly. String jsonValue = JSONValue.toJSONString(crunchifyMap); log("\nMethod-4: Using JSONValue.toJSONString() ==> " + jsonValue); } private static void log(Object print) { System.out.println(print); } }
Just run above Program as Java Application and you should see below output.
Raw Map ===> {Google=San Jose, Twitter=SFO, Microsoft=Seattle, Facebook=Mountain View, Crunchify=NYC} Method-1: Using Google GSON ==> {"Google":"San Jose","Twitter":"SFO","Microsoft":"Seattle","Facebook":"Mountain View","Crunchify":"NYC"} Pretty JSONObject ==> { "Google": "San Jose", "Twitter": "SFO", "Microsoft": "Seattle", "Facebook": "Mountain View", "Crunchify": "NYC" } Method-2: Using new JSONObject() ==> {"Google":"San Jose","Twitter":"SFO","Microsoft":"Seattle","Facebook":"Mountain View","Crunchify":"NYC"} Method-3: Using ObjectMapper().writeValueAsString() ==> {"Google":"San Jose","Twitter":"SFO","Microsoft":"Seattle","Facebook":"Mountain View","Crunchify":"NYC"} Method-4: Using JSONValue.toJSONString() ==> {"Google":"San Jose","Twitter":"SFO","Microsoft":"Seattle","Facebook":"Mountain View","Crunchify":"NYC"}
If you know any other way to convert Java Map to JSONObject then please let us and all readers know by comment below.
Happy coding.