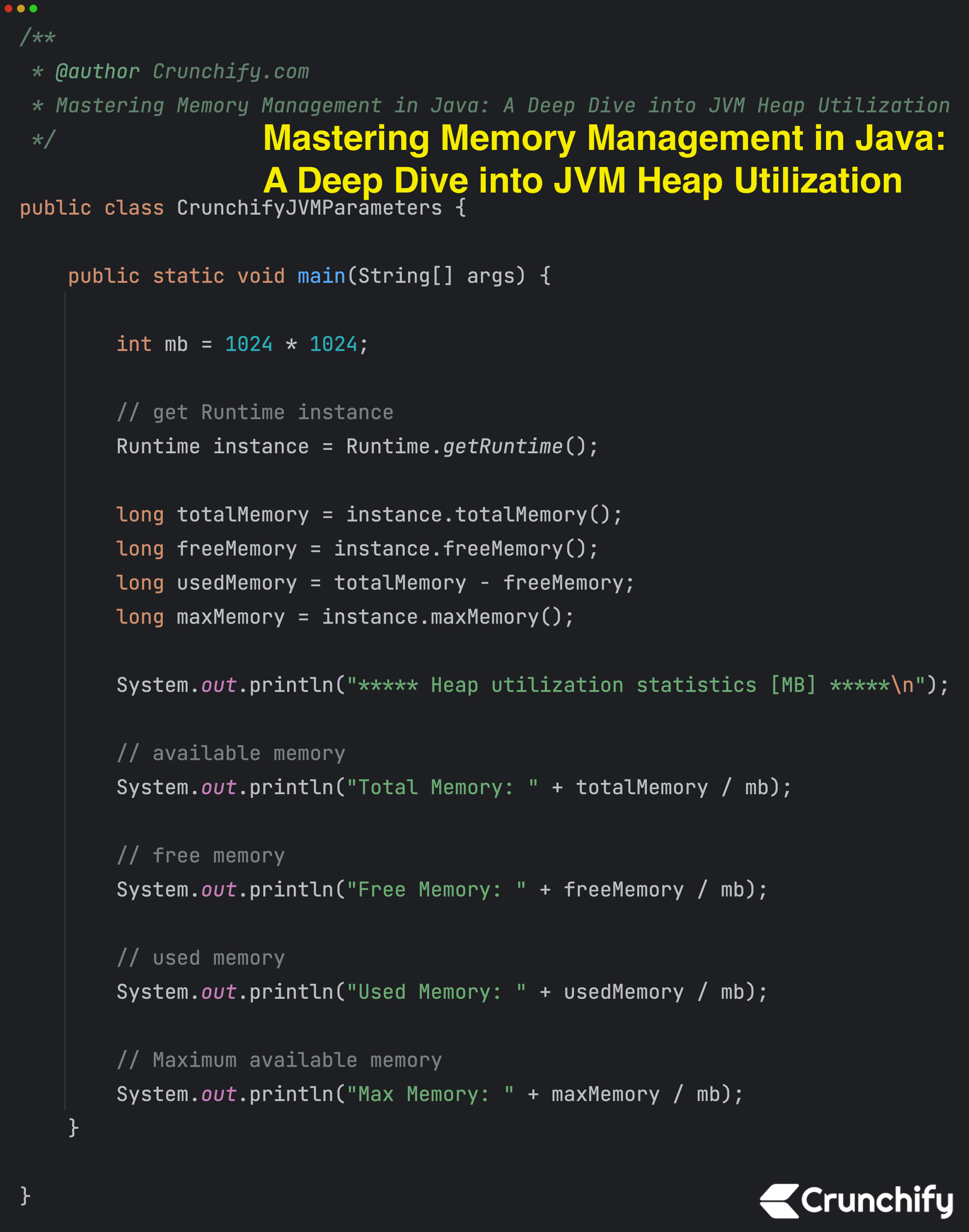
Although Java provides automatic garbage collection, sometimes you will want to know how large the object heap is and how much of it is left. You can use this information, for example, to check your code for efficiency or to approximate how many more objects of a certain type can be instantiated. To obtain these values, use the totalMemory( ) and freeMemory( ) methods.
The java.lang.Runtime.totalMemory()
method returns the total amount of memory in the Java virtual machine. The value returned by this method may vary over time, depending on the host environment. Note that the amount of memory required to hold an object of any given type may be implementation-dependent.
This is a simple Java code that demonstrates how to retrieve and display various memory-related statistics of the Java Virtual Machine (JVM) using the Runtime
class. The JVM is the environment in which Java programs run, and it manages memory allocation and other system resources.
Here’s a breakdown of what the code does:
- Import Statements:
package com.crunchify.tutorials;
: This declares the package in which the class resides.
- Class Declaration:
public class CrunchifyJVMParameters { ... }
: This defines a public class namedCrunchifyJVMParameters
.
- Main Method:
public static void main(String[] args) { ... }
: This is the entry point of the program. The code inside this method will be executed when the program is run.
- Memory Calculation:
int mb = 1024 * 1024;
: This creates an integer variablemb
and initializes it with the value 1024 * 1024, which represents one megabyte (MB) in bytes.
- Runtime Instance:
Runtime instance = Runtime.getRuntime();
: This line gets an instance of theRuntime
class, which provides information about the Java runtime environment, including memory usage.
- Output:
- System.out.println(“***** Heap utilization statistics [MB] *****\n”);: This prints a title for the memory statistics section.
- System.out.println(“Total Memory: ” + instance.totalMemory() / mb);: Prints the total amount of memory allocated to the JVM.
- System.out.println(“Free Memory: ” + instance.freeMemory() / mb);: Prints the amount of free memory available in the JVM.
- System.out.println(“Used Memory: ” + (instance.totalMemory() – instance.freeMemory()) / mb);: Calculates and prints the amount of memory currently in use by subtracting the free memory from the total memory.
- System.out.println(“Max Memory: ” + instance.maxMemory() / mb);: Prints the maximum amount of memory that the JVM can allocate.
- Closing Braces:
- The closing braces
}
mark the end of themain
method and theCrunchifyJVMParameters
class.
- The closing braces
Java Code
package crunchify.com.tutorials; /** * @author Crunchify.com * Mastering Memory Management in Java: A Deep Dive into JVM Heap Utilization */ public class CrunchifyJVMParameters { public static void main(String[] args) { int mb = 1024 * 1024; // get Runtime instance Runtime instance = Runtime.getRuntime(); long totalMemory = instance.totalMemory(); long freeMemory = instance.freeMemory(); long usedMemory = totalMemory - freeMemory; long maxMemory = instance.maxMemory(); System.out.println("***** Heap utilization statistics [MB] *****\n"); // available memory System.out.println("Total Memory: " + totalMemory / mb); // free memory System.out.println("Free Memory: " + freeMemory / mb); // used memory System.out.println("Used Memory: " + usedMemory / mb); // Maximum available memory System.out.println("Max Memory: " + maxMemory / mb); } }
Eclipse console Output:
##### Heap utilization statistics [MB] ##### Total Memory: 71 Free Memory: 70 Used Memory: 0 Max Memory: 1061
When you run this Java program, it will provide you with information about the heap memory utilization of the JVM, including total memory, free memory, used memory, and the maximum available memory.
The program is useful for understanding how memory is managed within a Java application and can help diagnose memory-related issues.