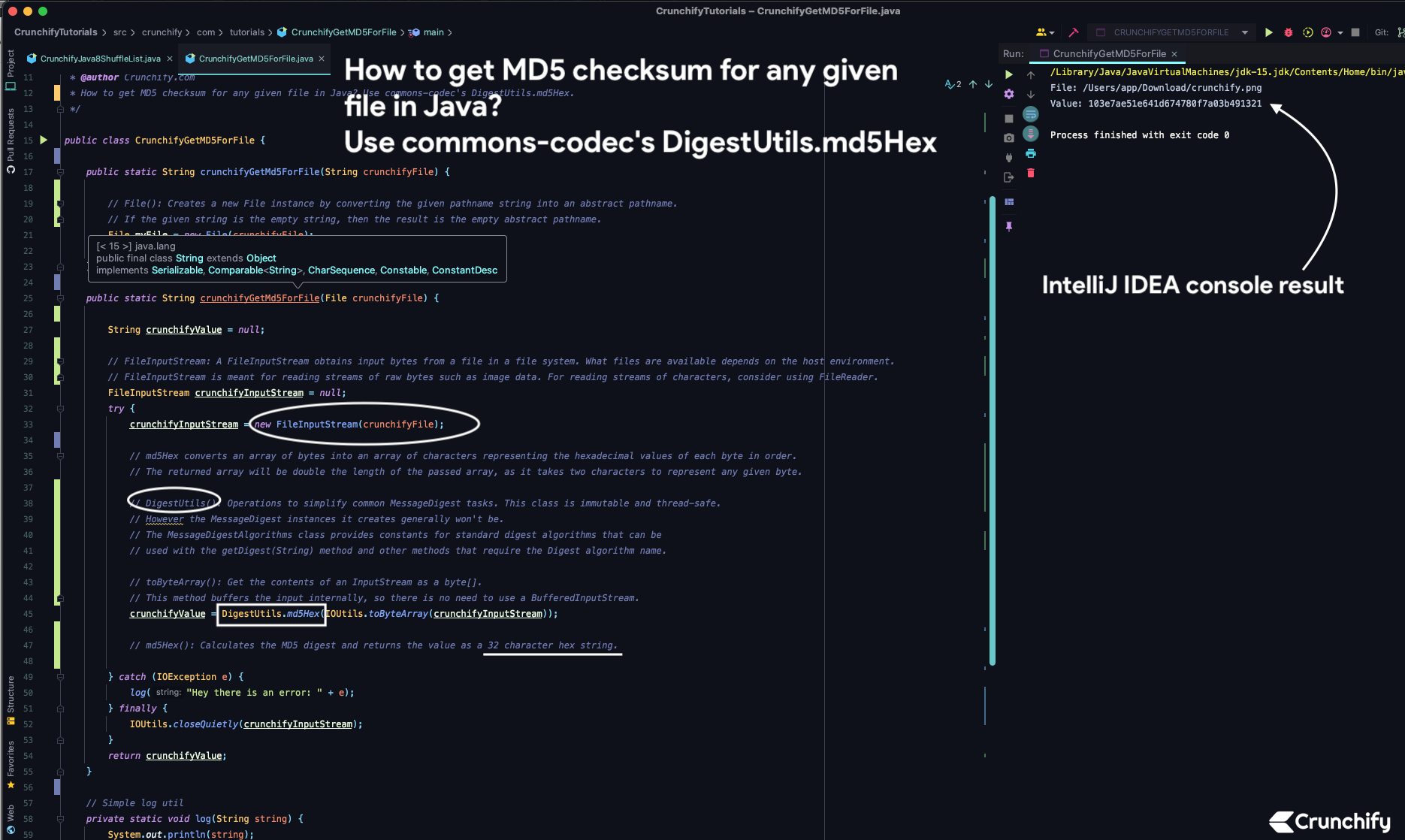
Tighten the security of your enterprise application is the biggest challenge of any organization.
Consider this actual production scenario:
- You have an application which
reads the value
from a file - Based on the file value it
performs some operations
like add/delete/execute - You have deployed this application in production environment
- What if some
unauthorized person
changes the value
of that file without your knowledge? - Your application
simply gets new value
from a file and runs the logic which may causeunexpected outcome
- If you may have MD5 checksum enabled for that file – you
could have created an exception
with clear error message andyou could have prevented disaster
or unexpected outcome
What is MD5 checksum?
The MD5 checksum for a file is a 128-bit value
, something like a fingerprint of the file. It can be useful both for comparing the files and their integrity control.
To get the same value for different file is nearly impossible. In this tutorial we will create simple Java program which creates and returns MD5 values for a given file. In our case it’s index.php
file.
Let’s get started:
Step-1
Create public class CrunchifyGetMD5ForFile.java
Step-2
Import below two maven dependencies to your project’s pom.xml
file. Tutorial to convert project to maven project.
<dependency> <groupId>commons-codec</groupId> <artifactId>commons-codec</artifactId> <version>1.10</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.4</version> </dependency>
We are using commons-codec
and commons-io
libraries.
Step-3
Create MD5 checksum using DigestUtils.md5Hex
utility and print result on console.
DigestUtils.md5Hex => encodeHex Implementation
protected static char[] encodeHex(final byte[] data, final char[] toDigits) { final int l = data.length; final char[] out = new char[l << 1]; // two characters form the hex value. for (int i = 0, j = 0; i < l; i++) { out[j++] = toDigits[(0xF0 & data[i]) >>> 4]; out[j++] = toDigits[0x0F & data[i]]; } return out; }
Here is a full program.
Create class crunchifyGetMd5ForFile.java
package crunchify.com.tutorials; import org.apache.commons.codec.digest.DigestUtils; import org.apache.commons.io.IOUtils; import java.io.File; import java.io.FileInputStream; import java.io.IOException; /** * @author Crunchify.com * How to get MD5 checksum for any given file in Java? Use commons-codec's DigestUtils.md5Hex. */ public class CrunchifyGetMD5ForFile { public static String crunchifyGetMd5ForFile(String crunchifyFile) { // File(): Creates a new File instance by converting the given pathname string into an abstract pathname. // If the given string is the empty string, then the result is the empty abstract pathname. File myFile = new File(crunchifyFile); return crunchifyGetMd5ForFile(myFile); } public static String crunchifyGetMd5ForFile(File crunchifyFile) { String crunchifyValue = null; // FileInputStream: A FileInputStream obtains input bytes from a file in a file system. What files are available depends on the host environment. // FileInputStream is meant for reading streams of raw bytes such as image data. For reading streams of characters, consider using FileReader. FileInputStream crunchifyInputStream = null; try { crunchifyInputStream = new FileInputStream(crunchifyFile); // md5Hex converts an array of bytes into an array of characters representing the hexadecimal values of each byte in order. // The returned array will be double the length of the passed array, as it takes two characters to represent any given byte. // DigestUtils(): Operations to simplify common MessageDigest tasks. This class is immutable and thread-safe. // However the MessageDigest instances it creates generally won't be. // The MessageDigestAlgorithms class provides constants for standard digest algorithms that can be // used with the getDigest(String) method and other methods that require the Digest algorithm name. // toByteArray(): Get the contents of an InputStream as a byte[]. // This method buffers the input internally, so there is no need to use a BufferedInputStream. crunchifyValue = DigestUtils.md5Hex(IOUtils.toByteArray(crunchifyInputStream)); // md5Hex(): Calculates the MD5 digest and returns the value as a 32 character hex string. } catch (IOException e) { log("Hey there is an error: " + e); } finally { // closeQuietly(): Unconditionally close an InputStream. // Equivalent to InputStream.close(), except any exceptions will be ignored. // This is typically used in finally blocks. IOUtils.closeQuietly(crunchifyInputStream); } return crunchifyValue; } // Simple log util private static void log(String string) { System.out.println(string); } public static void main(String[] agrs) { // Let's get MD5 for File index.php located at /Users/app/Download/ String file = "/Users/app/Download/crunchify.png"; String md5Value = crunchifyGetMd5ForFile(file); log("File: " + file + " \nValue: " + md5Value); } }
Just run above program as a Java Application and you will see result similar to this.
IntelliJ IDEA console result:
File: /Users/app/Download/crunchify.png Value: 103e7ae51e641d674780f7a03b491321 Process finished with exit code 0
How are you going to use this MD5 checksum at runtime to verify file integrity?
You could compare this MD5 checksum at runtime with value stored in y0ur database, like, MySQL, Oracle, etc.
There are number of other ways you could achieve the same but we will discuss this in future tutorials.