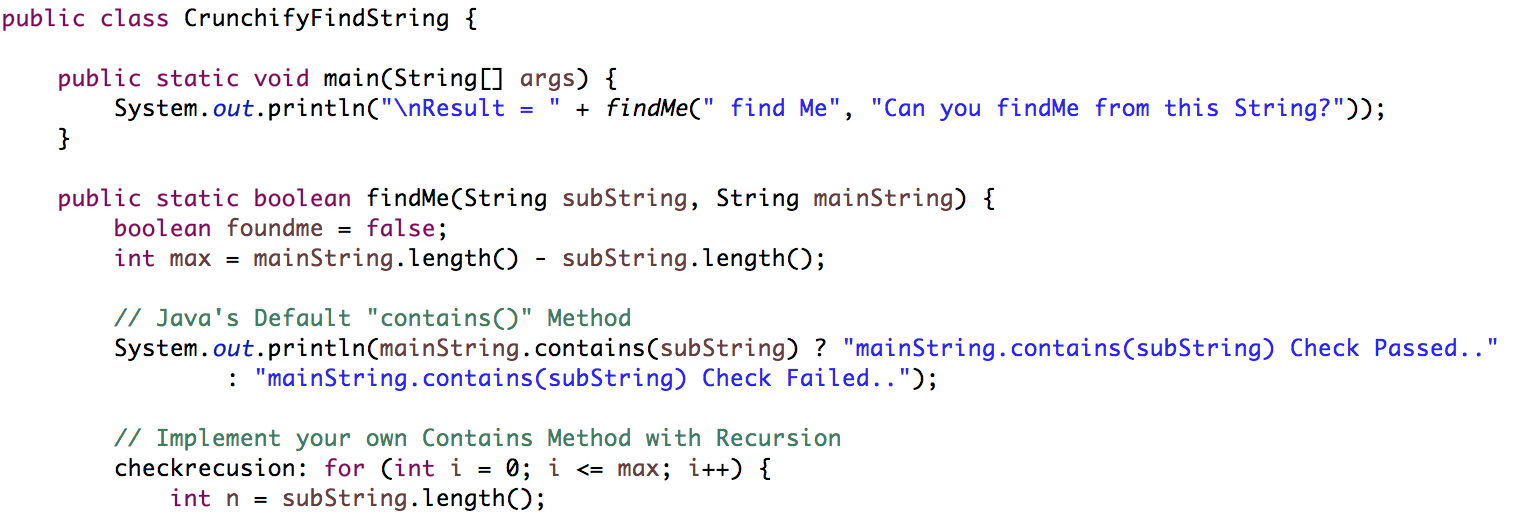
I have two Strings: str1 and str2. How to check if str2 is contained within str1? You can definitely use Java’s own .contains()
method.
In Java, there are several ways to check if a string contains a substring. The most common approach is to use the indexOf
method of the String
class:
public static boolean containsSubstring(String str, String subStr) { return str.indexOf(subStr) != -1; }
This method returns true
if the subStr
is found within the str
and false
otherwise. The indexOf
method returns the index of the first occurrence of the substring within the string. If the substring is not found, it returns -1
.
Another common approach is to use the contains
method of the String
class, which was added in Java 1.5:
public static boolean containsSubstring(String str, String subStr) { return str.contains(subStr); }
This method is more concise and returns true
if the subStr
is found within the str
and false
otherwise.
Implement your own findMe method.
Here by this program we will implement contains() method. Let’s call it as findMe(String subString, String mainString)
. Find below implementation.
package com.crunchify.tutorials; /** * @author Crunchify.com */ public class CrunchifyFindString { public static void main(String[] args) { System.out.println("\nResult = " + findMe(" findMe", "Can you findMe from this String?")); } public static boolean findMe(String subString, String mainString) { boolean foundme = false; int max = mainString.length() - subString.length(); // Java's Default "contains()" Method System.out.println(mainString.contains(subString) ? "mainString.contains(subString) Check Passed.." : "mainString.contains(subString) Check Failed.."); // Implement your own Contains Method with Recursion checkrecusion: for (int i = 0; i <= max; i++) { int n = subString.length(); int j = i; int k = 0; while (n-- != 0) { if (mainString.charAt(j++) != subString.charAt(k++)) { continue checkrecusion; } } foundme = true; break checkrecusion; } System.out .println(foundme ? "\nImplement your own Contains() Method - Result: Yes, Match Found.." : "\nImplement your own Contains() Method - Result: Nope - No Match Found.."); return foundme; } }
Eclipse Console result
Result-1:
mainString.contains(subString) Check Passed.. Implement your own Contains() method - Result: Yes, Match Found.. Result = true
Result-2: (notice blank space between find and Me)
mainString.contains(subString) Check Failed.. Implement your own Contains() Method - Result: Nope - No Match Found.. Result = false
Let me know if you know any better way to implement this.