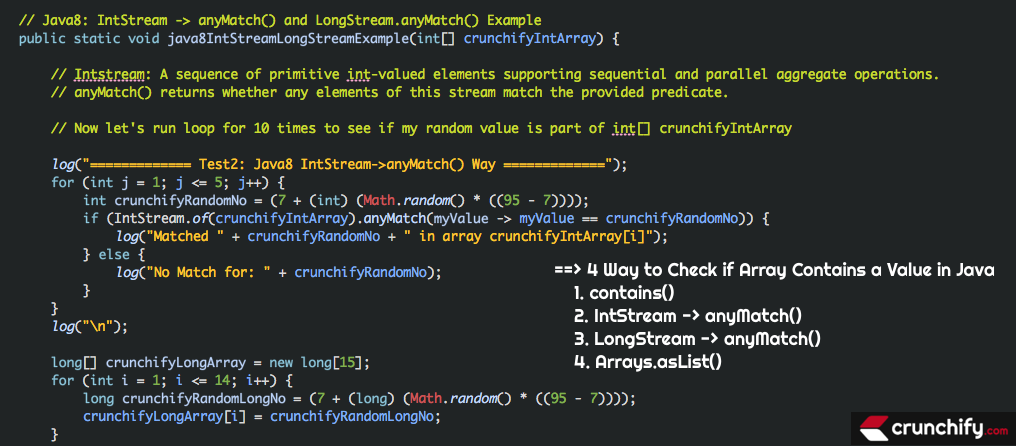
Java program for linear search algorithm.
This is the simplest and complete solution for your if you want to check if ArrayList contains specific value such as String, Integer, Long or Double.
With the combination of Java7 and Java8 – there are 4 different ways
you could perform contains check.
- Legacy String.contains() method
- Java8’s
IntStream -> anyMatch()
method - Java8’s
LongStream -> anyMatch()
method Arrays.asList
() method
We are going to discuss and check all above 4 methods in this Java Program. This java tutorial will work if you have below questions:
- How can I test if an array contains a certain value?
- check if array contains value in java
- java array contains int
- check if array contains string javascript
If you wonder, is there any way to override contains() method in Java? Well, then follow detailed tutorial on how to override contains() / findMe() method by yourself.
Let’s get started on implementing program with all above 4 methods:
Step-1
Let’s understand first our logic and what we are going to do in this Java tutorial.
- Create Java class
CrunchifyCheckIfContains
.java - Create int[]
crunchifyIntArray
with 15 elements in it - Print (system.out.println) all 15 elements
- Then Test-1: get Random 5 values and check
- if value contains then we will print
Matched
- if value doesn’t match then print
No Match
- if value contains then we will print
- Test-2: Perform same check with Java8 Utility using IntStream -> anyMatch()
- Test-3: Create
crunchifyLongArray
with 15 elements and perform check with Java8 Utility using LongStream -> anyMatch() - Test-4: Perform same contains check using Arrays.asList() method
Step-2
Copy complete below program in Eclipse IDE.
package crunchify.com.tutorials; import java.util.Arrays; import java.util.List; import java.util.stream.IntStream; import java.util.stream.LongStream; /** * @author Crunchify.com * version: 1.0 */ public class CrunchifyCheckIfContains { public static void main(String[] args) { int crunchifyRandomNo; // Let's create integer array with 15 values in it int[] crunchifyIntArray = new int[15]; for (int i = 1; i <= 14; i++) { crunchifyRandomNo = (7 + (int) (Math.random() * ((95 - 7)))); crunchifyIntArray[i] = crunchifyRandomNo; } log("Here is an array crunchifyIntArray[i] ==> " + Arrays.toString(crunchifyIntArray)); log("\n"); // Now let's run loop for 10 times to see if my random value is part of int[] crunchifyIntArray log("============= Test1: Legacy Java7 Way ============="); for (int j = 1; j <= 5; j++) { crunchifyRandomNo = (7 + (int) (Math.random() * ((95 - 7)))); if (crunchifyContainsMethod(crunchifyIntArray, crunchifyRandomNo)) { log("Matched: " + crunchifyRandomNo + " in array crunchifyIntArray[i]"); } else { log("No Match for: " + crunchifyRandomNo); } } log("\n"); // this is java8 way to find if Array contains specified value. java8IntStreamLongStreamExample(crunchifyIntArray); java8ArraysAsListExample(crunchifyIntArray); } // Java7: Simple Legacy way to check if specified value is there in Array :) public static boolean crunchifyContainsMethod(int[] crunchifyIntArray, int matchedValue) { for (int isMatched : crunchifyIntArray) { if (isMatched == matchedValue) { return true; } } return false; } // Java8: IntStream -> anyMatch() and LongStream.anyMatch() Example public static void java8IntStreamLongStreamExample(int[] crunchifyIntArray) { // Intstream: A sequence of primitive int-valued elements supporting sequential and parallel aggregate operations. // anyMatch() returns whether any elements of this stream match the provided predicate. // Now let's run loop for 10 times to see if my random value is part of int[] crunchifyIntArray log("============= Test2: Java8 IntStream->anyMatch() Way ============="); for (int j = 1; j <= 5; j++) { int crunchifyRandomNo = (7 + (int) (Math.random() * ((95 - 7)))); if (IntStream.of(crunchifyIntArray).anyMatch(myValue -> myValue == crunchifyRandomNo)) { log("Matched " + crunchifyRandomNo + " in array crunchifyIntArray[i]"); } else { log("No Match for: " + crunchifyRandomNo); } } log("\n"); long[] crunchifyLongArray = new long[15]; for (int i = 1; i <= 14; i++) { long crunchifyRandomLongNo = (7 + (long) (Math.random() * ((95 - 7)))); crunchifyLongArray[i] = crunchifyRandomLongNo; } log("Here is an array crunchifyLongArray[i] ==> " + Arrays.toString(crunchifyLongArray)); log("\n============= Test3: Java8 LongStream->anyMatch() Way ============="); for (int j = 1; j <= 5; j++) { long crunchifyRandomNo = (7 + (long) (Math.random() * ((95 - 7)))); if (LongStream.of(crunchifyLongArray).anyMatch(myValue -> myValue == crunchifyRandomNo)) { log("Matched " + crunchifyRandomNo + " in array crunchifyLongArray[i]"); } else { log("No Match for: " + crunchifyRandomNo); } } log("\n"); } // Java8: Arrays.asList() contains() and containsAll() Example private static void java8ArraysAsListExample(int[] crunchifyIntArray) { String[] crunchifyCompany = new String[] { "Facebook", "Twitter", "Google" }; // Convert String Array to List List<String> crunchifyList = Arrays.asList(crunchifyCompany); log("============= Test4: Arrays.asList() contains() and containsAll() Example ============="); if (crunchifyList.contains("Twitter")) { log("Matched Found for Twitter"); } if (crunchifyList.contains("Twitter") || crunchifyList.contains("Facebook")) { log("Matched Found for Twitter and Facebook"); } // A and B if (crunchifyList.containsAll(Arrays.asList("Google", "Facebook"))) { log("Matched Found for Google and Facebook"); } } // Simple log utility private static void log(String string) { System.out.println(string); } }
Step-3
Run the program in Eclipse using right click on program and click on Run as Java Application. You should see console result similar to this:
https://crunchify.com/how-to-find-alexa-rank-meta-tag-value-for-all-in-one-webmaster-premium/comment-page-1/#comment-59190 Here is an array crunchifyIntArray[i] ==> [0, 72, 26, 87, 12, 45, 27, 34, 12, 89, 9, 65, 79, 15, 16] ============= Test1: Legacy Java7 Way ============= No Match for: 32 No Match for: 33 Matched 79 in array crunchifyIntArray[i] No Match for: 40 Matched 12 in array crunchifyIntArray[i] ============= Test2: Java8 IntStream->anyMatch() Way ============= Matched 34 in array crunchifyIntArray[i] No Match for: 58 No Match for: 62 Matched 27 in array crunchifyIntArray[i] No Match for: 17 Here is an array crunchifyLongArray[i] ==> [0, 74, 93, 61, 78, 42, 74, 52, 59, 88, 61, 34, 27, 59, 84] ============= Test3: Java8 LongStream->anyMatch() Way ============= No Match for: 15 Matched 84 in array crunchifyLongArray[i] No Match for: 23 No Match for: 39 No Match for: 69 ============= Test4: Arrays.asList() contains() and containsAll() Example ============= Matched Found for Twitter Matched Found for Twitter and Facebook Matched Found for Google and Facebook
Let me know if you have any question.