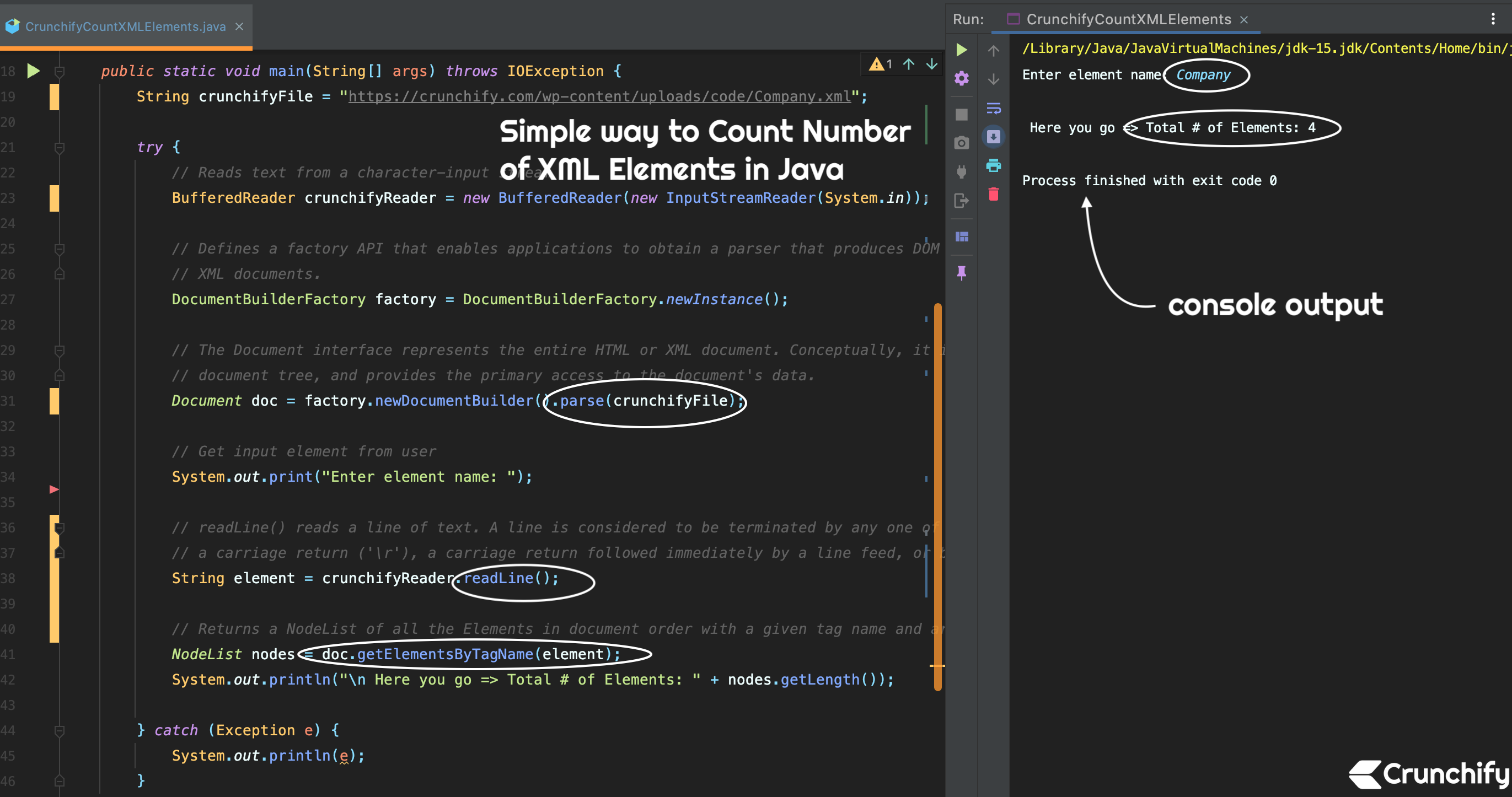
In this example we will learn simple way to count number of XML elements in Java program.
This tutorial works if you have any of below questions:
- Count XML Elements in Java using DOM parser example
- How to count XML Elements in Java
- How count leaf elements in xml file in java
- XPath count example in Java
- Simplest way to get XML node count
The Document
interface represents the entire HTML or XML document. Conceptually, it is the root of the document tree, and provides the primary access to the document’s data.
getElementsByTagName
returns a NodeList
of all the Elements
in document order with a given tag name and are contained in the document.
Here is a sample XML file:
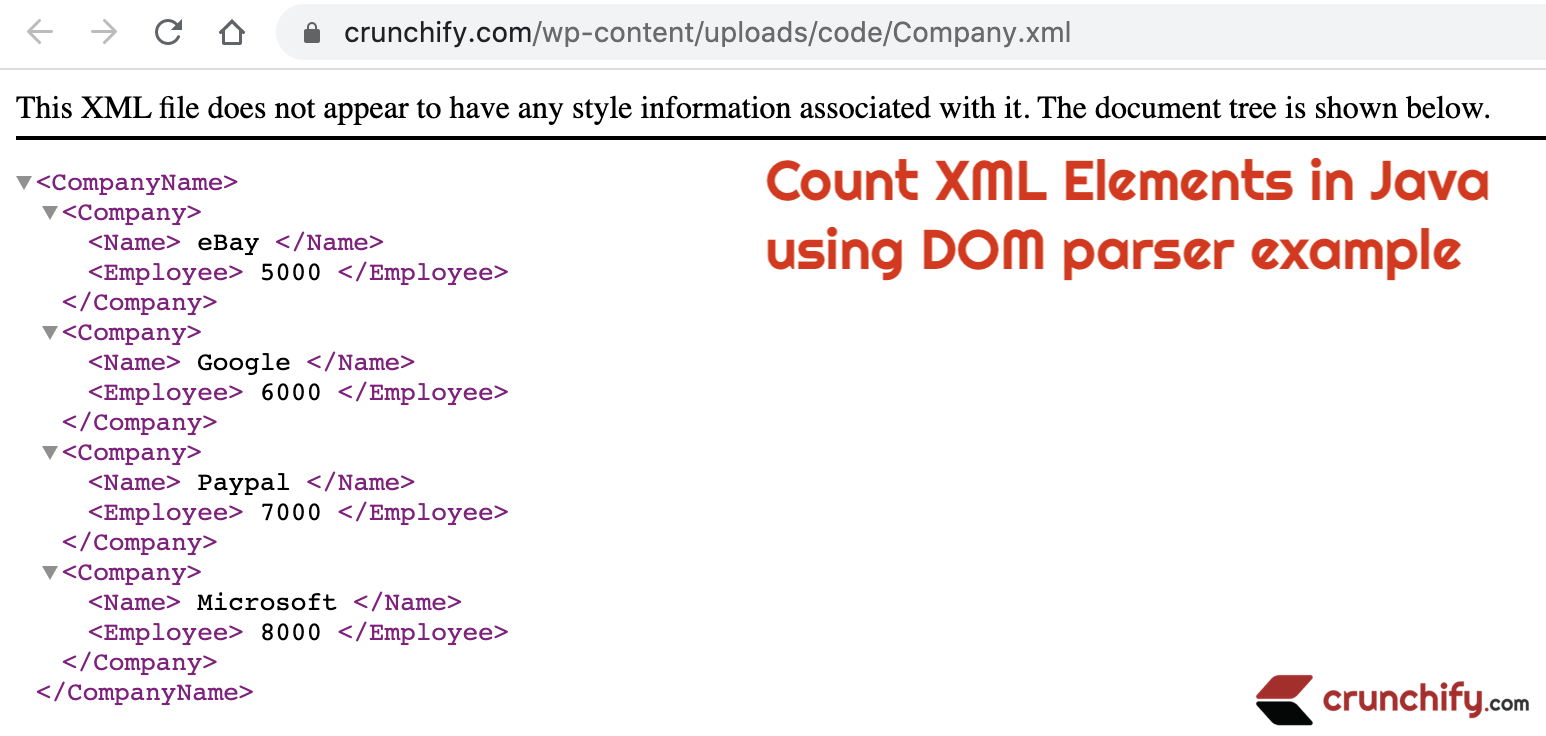
Just create a file called Company.xml
and put it on your laptop/desktop. Make sure to update below java program and update file path location.
<CompanyName> <Company> <Name> eBay </Name> <Employee> 5000 </Employee> </Company> <Company> <Name> Google </Name> <Employee> 6000 </Employee> </Company> <Company> <Name> Paypal </Name> <Employee> 7000 </Employee> </Company> <Company> <Name> Microsoft </Name> <Employee> 8000 </Employee> </Company> </CompanyName>
Java Program:
Create file CrunchifyCountXMLElements.java
. Put below Java code and save it.
package crunchify.com.tutorials; import org.w3c.dom.Document; import org.w3c.dom.NodeList; import javax.xml.parsers.DocumentBuilderFactory; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; /** * @author Crunchify.com * Simple way to Count Number of XML Elements in Java */ public class CrunchifyCountXMLElements { public static void main(String[] args) throws IOException { String crunchifyFile = "https://crunchify.com/wp-content/uploads/code/Company.xml"; try { // Reads text from a character-input stream BufferedReader crunchifyReader = new BufferedReader(new InputStreamReader(System.in)); // Defines a factory API that enables applications to obtain a parser that produces DOM object trees from // XML documents. DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); // The Document interface represents the entire HTML or XML document. Conceptually, it is the root of the // document tree, and provides the primary access to the document's data. Document doc = factory.newDocumentBuilder().parse(crunchifyFile); // Get input element from user System.out.print("Enter element name: "); // readLine() reads a line of text. A line is considered to be terminated by any one of a line feed ('\n'), // a carriage return ('\r'), a carriage return followed immediately by a line feed, or by reaching the end-of-file (EOF). String element = crunchifyReader.readLine(); // Returns a NodeList of all the Elements in document order with a given tag name and are contained in the document. NodeList nodes = doc.getElementsByTagName(element); System.out.println("\n Here you go => Total # of Elements: " + nodes.getLength()); } catch (Exception e) { System.out.println(e); } } }
Details on javax.xml.parsers.DocumentBuilder API:
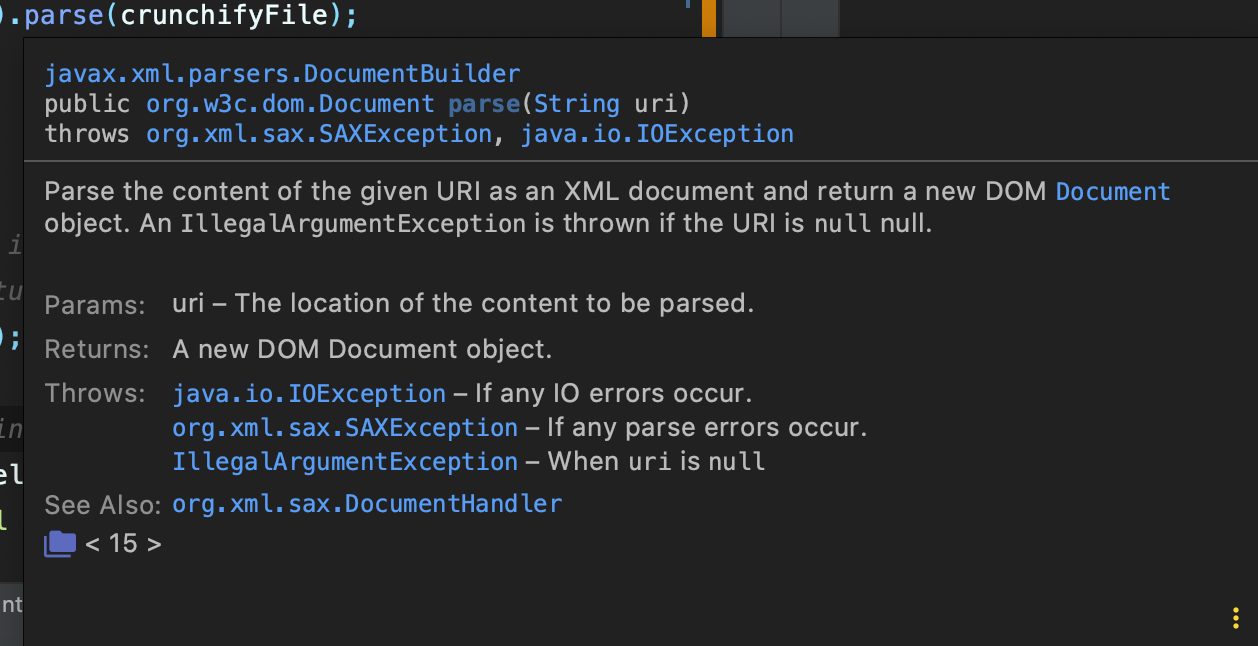
Details on doc.getElementByTagname API:
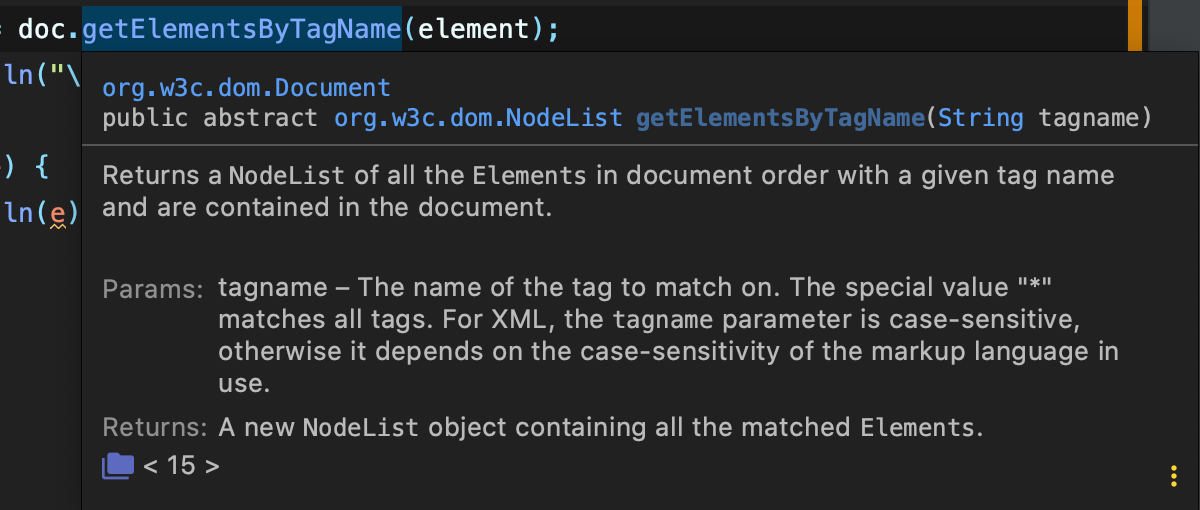
Eclipse Console Result:
Just run above program as Java Application and you should see same result as below in your Eclipse IDE or IntelliJ IDEA.
/Library/Java/JavaVirtualMachines/jdk-15.jdk/Contents/Home/bin/java -javaagent:/Applications/IntelliJ IDEA.app/Contents/lib/idea_rt.jar=57642://Users/app/.m2/repository/org/springframework/spring-context-support/5.1.3.RELEASE/spring-context-support-5.1.3.RELEASE.jar crunchify.com.tutorials.CrunchifyCountXMLElements Enter element name: Company Here you go => Total # of Elements: 4 Process finished with exit code 0