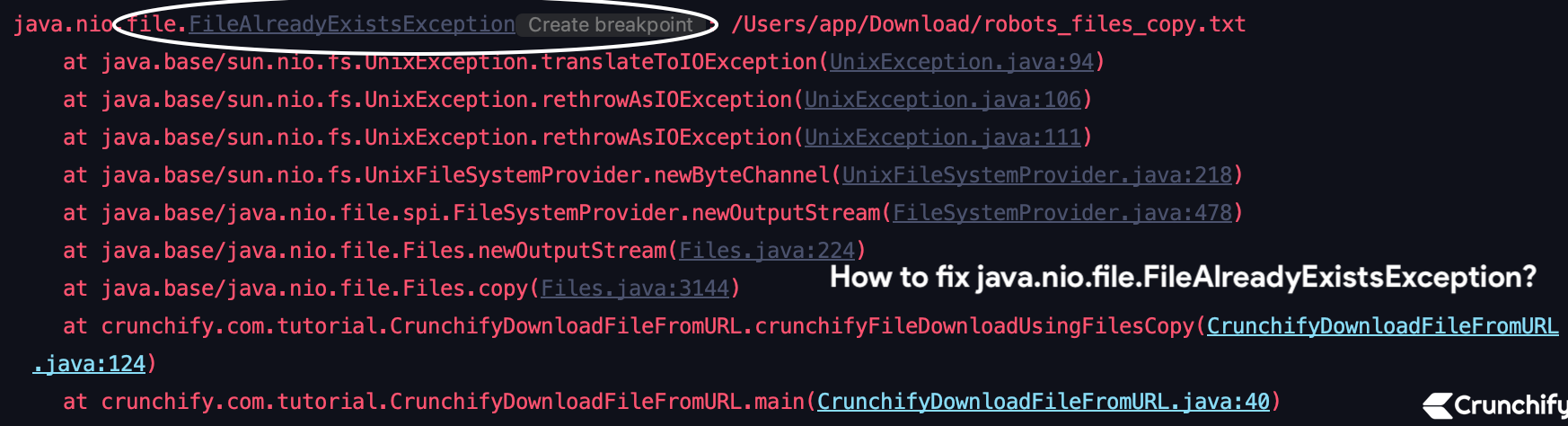
Are you getting java.nio.file.FileAlreadyExistsException error?
java.nio.file.FileAlreadyExistsException: /Users/app/Download/robots_files_copy.txt at java.base/sun.nio.fs.UnixException.translateToIOException(UnixException.java:94) at java.base/sun.nio.fs.UnixException.rethrowAsIOException(UnixException.java:106) at java.base/sun.nio.fs.UnixException.rethrowAsIOException(UnixException.java:111) at java.base/sun.nio.fs.UnixFileSystemProvider.newByteChannel(UnixFileSystemProvider.java:218) at java.base/java.nio.file.spi.FileSystemProvider.newOutputStream(FileSystemProvider.java:478) at java.base/java.nio.file.Files.newOutputStream(Files.java:224) at java.base/java.nio.file.Files.copy(Files.java:3144) at crunchify.com.tutorial.CrunchifyDownloadFileFromURL.crunchifyFileDownloadUsingFilesCopy(CrunchifyDownloadFileFromURL.java:124) at crunchify.com.tutorial.CrunchifyDownloadFileFromURL.main(CrunchifyDownloadFileFromURL.java:40)
On Crunchify, we have more than 700 Java tutorials and 500+ WordPress tutorials. While running few Java programs today, I suddenly started getting above error from one of my Java class.
Here is my code:
// Method-4: File Download using Files.copy() private static void crunchifyFileDownloadUsingFilesCopy(String crunchifyURL, String crunchifyFileLocalPath) { // URI Creates a URI by parsing the given string. // This convenience factory method works as if by invoking the URI(String) constructor; // any URISyntaxException thrown by the constructor is caught and wrapped in a new IllegalArgumentException object, which is then thrown. try (InputStream crunchifyInputStream = URI.create(crunchifyURL).toURL().openStream()) { // Files: This class consists exclusively of static methods that operate on files, directories, or other types of files. // copy() Copies all bytes from an input stream to a file. On return, the input stream will be at end of stream. // Paths: This class consists exclusively of static methods that return a Path by converting a path string or URI. // Paths.get() Converts a path string, or a sequence of strings that when joined form a path string, to a Path. Files.copy(crunchifyInputStream, Paths.get(crunchifyFileLocalPath)); } catch (IOException e) { e.printStackTrace(); } }
In order to fix above error I had to replace one line with extra parameter StandardCopyOption.REPLACE_EXISTING
.
Before:
Files.copy(crunchifyInputStream, Paths.get(crunchifyFileLocalPath));
After:
Files.copy(crunchifyInputStream, Paths.get(crunchifyFileLocalPath), StandardCopyOption.REPLACE_EXISTING);
REPLACE_EXISTING
replaces an existing file if it exists.
That’s it. Now you won’t get above error any more. This step works for File.move() operation too.