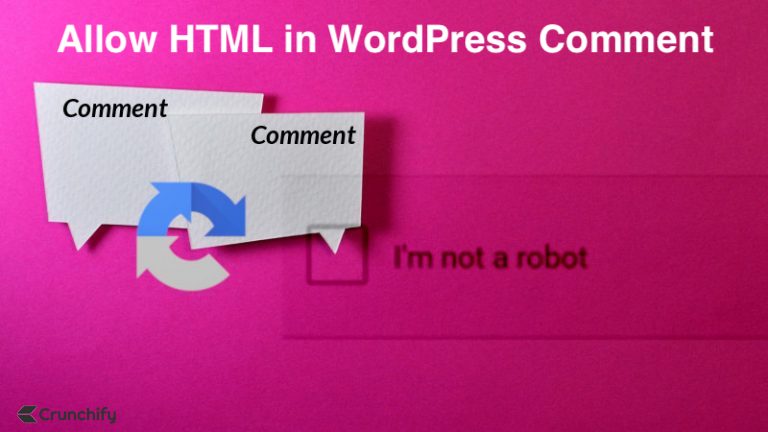
Tips on Expanding the allowed HTML tags in comments
WordPress allows a select few HTML tags within the content of post comments. This is an awesome feature, of course, because it prevents XSS security holes and other malicious code from being injected by spammers, hackers.
Unfortunately there are many other tags that bloggers may want to support; for example, tech bloggers may want to support PRE
tags so commenters can post code. Luckily it’s quite easy to allow more tags within your WordPress comments.
There are two methods for allowing HTML tags in WordPress comment forms:
- Using a Plugin
- Modifying the functions.php file
Using a plugin:
- Installing a plugin is often the easiest and quickest method for allowing HTML tags in WordPress comment forms.
- There are several plugins available in the WordPress plugin repository that are designed specifically for this purpose, such as “Allow HTML in Comments” and “HTML in Comment”.
- To use a plugin, simply navigate to the “Plugins” section of your WordPress dashboard, click the “Add New” button, search for the plugin you want to use, and then install and activate it.
- After activation, the plugin will automatically enable HTML in the comment form, and you can configure the allowed HTML tags in the plugin settings.
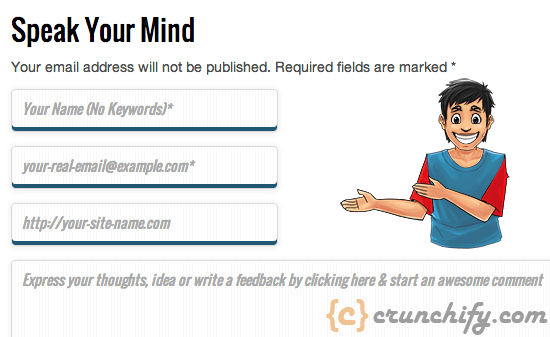
Modifying the functions.php file:
- The functions.php file is a part of your WordPress theme and can be used to add custom functionality to your site.
- To modify the functions.php file, you will need access to your website’s file system, either via FTP or using the file manager in your hosting account control panel.
- Once you have access to your file system, navigate to the “wp-content/themes/[your theme name]” folder, find the functions.php file, and edit it using a text editor.
- Add the following code to the end of the file:
More Details:
In WordPress tags that are allowed in comments are stored in the $allowedtags global variable
. You could try adding HTML elements to that list (the key is the tag name, the value is an array of allowed attributes).
To Add more elements:
Add below code to themes’ functions.php
file.
// Create function which allows more tags within comments function crunchify_allowed_html_tags() { return array( 'a' => array( 'href' => true, 'title' => true, ), 'br' => array(), 'em' => array(), 'strong' => array(), 'pre' => array(), 'p' => array(), 'code' => array(), ); } add_filter( 'wp_kses_allowed_html', 'crunchify_allowed_html_tags', 1 );
- This code creates a function called
my_allowed_html_tags
that defines an array of HTML tags that are allowed in the comment form. In this example, the tags include<a>
,<br>
,<em>
, and<strong>
. - The
add_filter
function then attaches this custom function to thewp_kses_allowed_html
filter, which is used by WordPress to determine which HTML tags are allowed in comments. The1
argument specifies the priority of the filter. - After you have added this code to the functions.php file, save the changes and upload the file back to your website.
Please note: Allowing HTML in comments can make your site vulnerable to security threats such as cross-site scripting (XSS) attacks. It’s important to be careful when allowing HTML and to always keep your site up-to-date with the latest security updates.
The global $allowedtags
variable holds an array of allowed comment tags, so adding the pre
key will allow PRE
elements within comments. The class
key within the pre
array allows the class
attribute for any PRE tags posted within the comment, so not only can you allow additional HTML tags, but you can also specify allowed attributes too!
To Remove more elements:
function crunchify_remove_html_attributes_in_commentform() { global $allowedtags; // remove crunchify_tags_to_remove tags $crunchify_tags_to_remove = array( 'blockquote', 'cite', 'code', 'del', 'pre' ); foreach ( $crunchify_tags_to_remove as $tag ) unset( $allowedtags[$tag] ); // add wanted tags $crunchify_newTags = array( 'span' => array( 'lang' => array()), 'u' => array() ); $allowedtags = array_merge( $allowedtags, $crunchify_newTags ); } add_action('init', 'crunchify_remove_html_attributes_in_commentform', 11 );
Above functions add/remove the comment_post
hook so it only adds desired tags when a comment is posted.