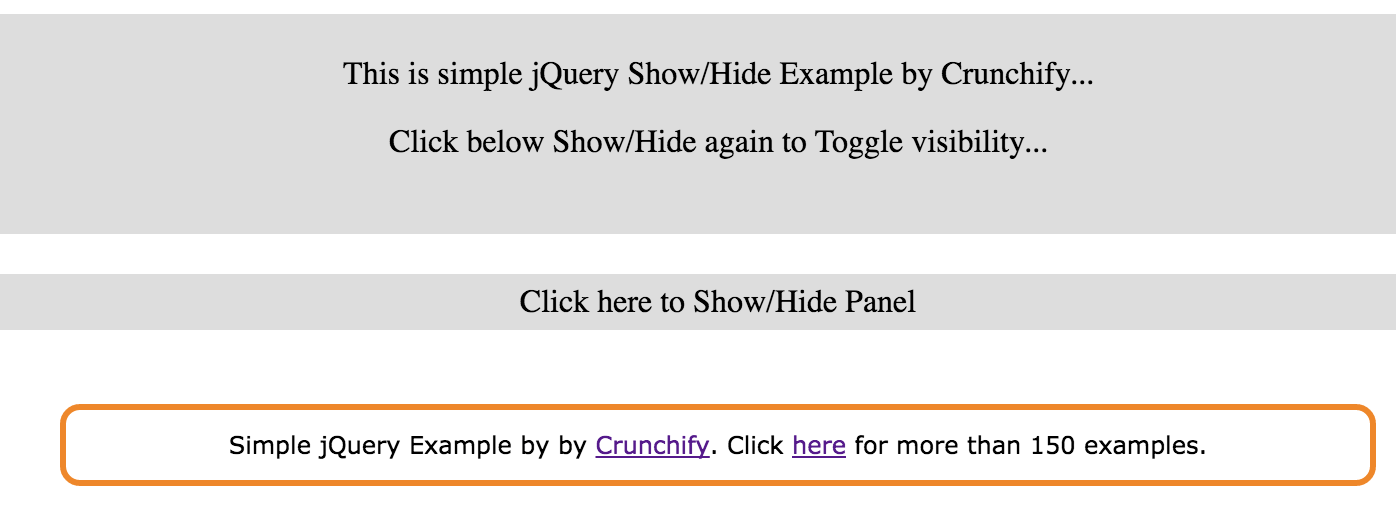
Here’s a simple tutorial on how to show/ hide a div using jQuery.
The simple jQuery Show/Hide Example is a user interface component that allows users to toggle the visibility of a specific element on a webpage. It is implemented using the jQuery library, a popular JavaScript library that simplifies the process of working with the Document Object Model (DOM).
The Show/Hide component typically consists of a button or link that the user can click to toggle the visibility of a particular element, such as a menu, a form, or a message. The component uses the jQuery show() and hide() methods to toggle the display of the selected element.
The jQuery Show/Hide Example can be customized to fit the specific requirements of different web applications, and can be enhanced with animations or other visual effects to provide a more engaging user experience. It can also be used in conjunction with other jQuery plugins to create more complex user interfaces.
The simple jQuery Show/Hide Example is a useful tool for web developers who need to implement a basic element toggling functionality in their web applications. It is easy to implement, lightweight, and can be used to enhance the usability and functionality of web applications.
Live Example:
This is simple jQuery Show/Hide Example by Crunchify…
Click below Show/Hide again to Toggle visibility…
Click here to Show/Hide Panel
Crunchify.jQuery.Show.Hide.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta rel="author" href="https://Crunchify.com" content=""> <title>This is simple jQuery Show/Hide Example by Crunchify</title> <meta name="description" content="This is simple jQuery Show/Hide Example by Crunchify."> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <script type="text/javascript" src="https://code.jquery.com/jquery-1.10.1.min.js"></script> <script type="text/javascript"> $(document).ready(function() { $(".flip").click(function() { $(".panel").slideToggle("slow"); }); }); </script> <style type="text/css"> div.panel,p.flip { margin: 0px; padding: 5px; text-align: center; background: #ddd; border: solid 1px #fff; } div.panel { widht: 50%; height: 100px; display: none; } p { width: 70%; } </style> </head> <body> <br><br> <div align="center"> <h1>Part of Crunchify Tutorial Series...</h1> </div> <div class="panel"> <p>This is simple jQuery Show/Hide Example by Crunchify...</p> <p>Click below Show/Hide again to Toggle visibility...</p> </div> <br> <p class="flip">Click here to Show/Hide Panel</p> <br> <br> <div align="center"> <div style="font-family: verdana; padding: 10px; border-radius: 10px; border: 3px solid #EE872A; width: 50%; font-size: 12px;"> Simple jQuery Example by by <a href='https://crunchify.com'>Crunchify</a>. Click <a href='https://crunchify.com/category/java-tutorials/'>here</a> for more than 1050 examples.<br> </div> </div> </body> </html>
Another must read:
Is there a link to the “latest” jQuery library on Google APIs?
The jQuery API has a number of hosts that have recent and up-to-date versions:
https://code.jquery.com/jquery-3.6.3.min.js
– Most recent version, jQuery hostedhttps://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js
– Google hosted
For example:
<script src="https://code.jquery.com/jquery-3.6.3.min.js" type="text/javascript"></script>
If you remove the http:
prefix, using Google’s hosted version of the jQuery API works seamlessly over both secure (https) and insecure (http
) connections.
What if you want to expand div
after 2 seconds onLoad (instead of mouse click)
Just put below code also after first jQuery function.
$(document).ready(function() { $(".panel").delay(2000).fadeIn(500); });
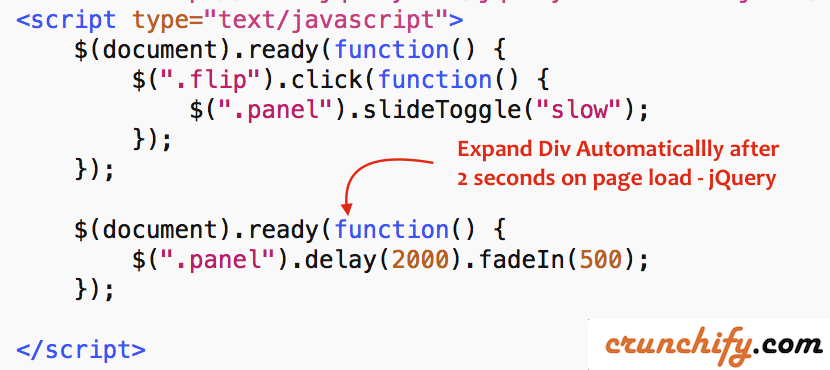
Let me know if you face any issue running this code.