What if you want to login to a website by filling Username/Email
and Password
automatically?
Well, there is a simple solution to that. This solution works for large number of web sites which have a login and password field contained within a form.
An Automatic HTML Login Demo Page is a web page that demonstrates how to create an HTML form that allows users to log in automatically, without having to enter their username and password each time they visit the website.
The form uses HTML and JavaScript to save the user’s login credentials in the browser’s local storage, which is a feature that allows web applications to store data on the user’s device.
The demo page typically includes a login form that prompts the user for their username and password, and a button that allows the user to save their credentials. Once the user has saved their credentials, the form will automatically fill in their login information the next time they visit the website.
The demo page is often used as a learning resource for web developers who want to add automatic login functionality to their own websites. It can be customized and modified to fit the specific requirements of different websites, and is a useful tool for improving the user experience and increasing engagement on web applications.
Here is a simple Login page (Crunchify-LoginPage.html) in which I have to provide Email and Password.
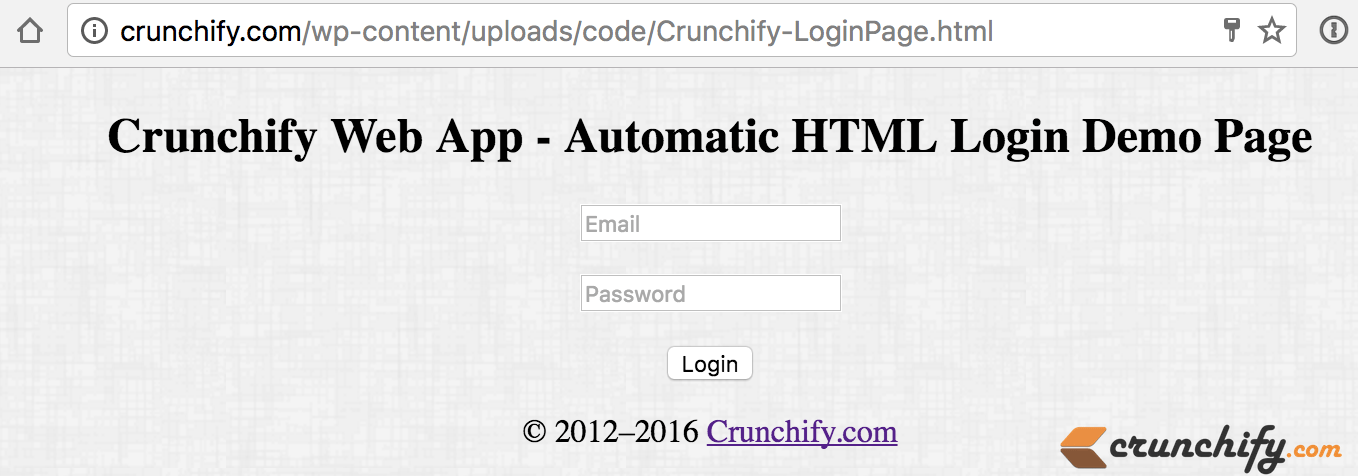
In order for you to fill above fields
automatically just create one sample file Crunchify.html
file on your Desktop or convenient location and put below content.
Crunchify.html File code
<html> <head> <title>Crunchify Login Page</title> <script> function loginForm() { document.myform.submit(); document.myform.action = "https://crunchify.com/wp-content/uploads/code/Crunchify-LoginPage.html"; } </script> <style type="text/css"> body { background-image: url('https://crunchify.com/bg.png'); } </style> </head> <body onload="loginForm()"> <form action="https://crunchify.com/wp-content/uploads/code/success.html" name="myform" method="post"> <input type="text" name="email" value="myemail@crunchify.com"> <input type="password" name="password" value="mypassw@rd"> <input type="submit" value="Login"> </form> </body> </html>
And that’s it. Just double click
on above file and you are all set.
Here is what will happen internally:
- When you open
Crunchify.html
file it will internally open your dedicated pageCrunchify-LoginPage.html
- Internally it will pass your email and password provided in Crunchify.html file
- Once submitted you will be proceeded to
success.html
page
This is very basic HTML tip but sometime it saves big time for repeated login activity.
Crunchify-LoginPage.html page source code
This is frequently used login page for this example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Crunchify - Login Form Example</title> <meta name="description" content="Crunchify - Login Form Example."> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style type="text/css"> body { background-image: url('https://crunchify.com/bg.png'); } p { width: 70%; } </style> </head> <body> <div align="center"> <section class="container"> <div class="login"> <h1>Crunchify Web App - Automatic HTML Login Demo Page</h1> <form name="myform" action="https://crunchify.com/wp-content/uploads/code/success.html"> <p><input type="text" name="email" value="" placeholder="Email"></p> <p><input type="password" name="password" value="" placeholder="Password"></p> <p class="submit"><input type="submit" name="submit" value="Login"></p> </form> </div> </section> <section class="about"> <p class="about-author"> © 2012–2023 <a href="https://crunchify.com" target="_blank">Crunchify.com</a> </section> </div> </body> </html>
Python Example:
Here is a complete example of automatically logging into a website using the HTTP POST method in Python:
import requests # Define the target URL for the login page url = "https://www.example.com/login" # Define the payload to be sent with the POST request, including the username and password payload = { "username": "user", "password": "pass" } # Send a POST request to the URL with the payload response = requests.post(url, data=payload) # Check the response status code to determine if the login was successful if response.status_code == 200: print("Login successful") else: print("Login failed")
Note that the above code is just an example, and the exact details may vary depending on the website you are trying to log in to.
You may need to modify the URL, payload, or other aspects of the code to match the specific requirements of the website.
Additionally, some websites may use additional security measures, such as anti-CSRF tokens or captchas, that would need to be handled in the code.